Crafting Dynamic Web Experiences: A Guide to Building Responsive User Interfaces with Vue.js
In the realm of modern web development, creating responsive and user-friendly interfaces is crucial to engaging users and ensuring a superior overall user experience. Vue.js, a progressive JavaScript framework, equips web developers, especially those you may want to hire as Vue.js developers, with an efficient, adaptable toolset for building advanced single-page applications. Its reactivity system is arguably its most powerful feature, enabling developers to build highly responsive interfaces that users love to interact with.
In this blog post, we’re going to delve into how to build responsive user interfaces (UI) using Vue.js, an area of expertise for competent Vue.js developers. We’ll begin by explaining some fundamental concepts and then apply these in practical, example-driven contexts. Whether you’re looking to understand the framework or planning to hire Vue.js developers, this guide should be beneficial.
Vue.js – A Quick Overview
Vue.js, created by Evan You in 2014, is a popular JavaScript framework for building interactive and responsive web interfaces. Vue.js follows the component-based architecture, which makes it easy to reuse and organize code. Its core library is focused on the view layer only, but it has the capability to be incrementally adoptable, meaning you can use as little or as much as you need, making it perfect for both small and large-scale projects.
Core Concepts in Vue.js
Before we delve into examples, let’s take a brief look at some core Vue.js concepts that will form the backbone of our responsive user interface:
– Reactivity: This is the principle that when data changes in Vue, the framework automatically updates the UI to reflect these changes.
– Components: In Vue.js, a component is a reusable instance with a name. Components are used to build UIs in Vue, and they are essentially Vue instances with predefined options.
– Directives: Directives in Vue.js are special attributes that start with `v-` and are used to bind reactive data to the DOM.
Building a Responsive UI: A Simple Task Tracker
To better understand how Vue.js can be used to build responsive UIs, let’s create a simple task tracker.
Firstly, we start by setting up a new Vue application:
```bash npm install -g @vue/cli vue create task-tracker ```
Once the project is created, navigate into the directory and start the application:
```bash cd task-tracker npm run serve ```
Step 1: Creating the Task Component
Our task tracker will consist of individual tasks. So, let’s start by creating a component for a task.
```javascript <template> <div class="task"> <h3>{{ task.text }}</h3> <p>{{ task.day }}</p> </div> </template> <script> export default { name: 'Task', props: { task: Object } } </script> ```
In this component, we’re accepting a `task` prop which we then use in the template to display the task’s text and day.
Step 2: Creating the Task List Component
Next, let’s create a task list component that will display all the tasks:
```javascript <template> <div> <task v-for="task in tasks" :key="task.id" :task="task"></task> </div> </template> <script> import Task from './Task.vue'; export default { name: 'TaskList', components: { Task }, props: { tasks: Array } } </script> ```
We’re accepting a `tasks` prop, which is an array of tasks, and then using the `v-for` directive to loop over each task and display it.
Step 3: Creating the Add Task Component
The next step is to create a component that allows the user to add a new task:
```javascript <template> <form @submit.prevent ="addTask"> <input type="text" v-model="text" placeholder="Add Task" required /> <input type="text" v-model="day" placeholder="Add Day & Time" required /> <input type="submit" value="Save Task" /> </form> </template> <script> export default { name: 'AddTask', data() { return { text: '', day: '' } }, methods: { addTask() { this.$emit('add-task', { text: this.text, day: this.day }); this.text = ''; this.day = ''; } } } </script> ```
In this component, we have two input fields bound to the `text` and `day` data properties using `v-model`. When the form is submitted, we prevent the default form submission action using `@submit.prevent`, then call the `addTask` method. This method emits an ‘add-task‘ event with the new task data, and then resets the input fields.
Step 4: Combining It All
Finally, we bring it all together in the `App` component:
```javascript <template> <div id="app"> <add-task @add-task="addNewTask"></add-task> <task-list :tasks="tasks"></task-list> </div> </template> <script> import AddTask from './components/AddTask.vue'; import TaskList from './components/TaskList.vue'; export default { name: 'App', components: { AddTask, TaskList }, data() { return { tasks: [ { id: 1, text: 'Doctor appointment', day: 'July 7 at 2:30pm' }, //...more tasks ] } }, methods: { addNewTask(task) { const id = this.tasks.length + 1; this.tasks.push({ id, ...task }); } } } </script> ```
In our `App` component, we include the `AddTask` and `TaskList` components. We pass the `tasks` data property to the `TaskList` and listen for the ‘add-task’ event on the `AddTask` component. When a new task is added, we create a new task with a unique id and the task data, then add it to our list of tasks.
Conclusion
In this post, we’ve demonstrated how Vue.js is a powerful tool for building responsive user interfaces, a skill mastered by proficient Vue.js developers. Thanks to its reactivity system, components, and directives, Vue.js allows developers to create interactive and intuitive web applications that greatly enhance user experiences.
While our simple task tracker merely scratches the surface of what Vue.js can achieve, it provides a solid foundation for those interested to hire Vue.js developers for more complex applications. By understanding the principles highlighted in this post, you can confidently evaluate the competence of Vue.js developers and ensure they leverage Vue.js’s full potential to create even more engaging and responsive user interfaces. Happy coding!
Table of Contents
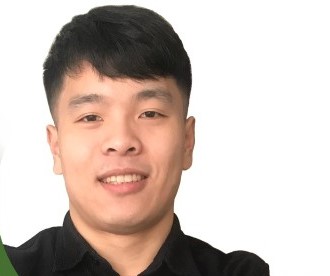
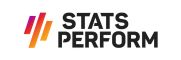