Vue.js Routing Demystified: A Comprehensive Guide to Navigating Your Web Application
If you’re working on a Vue.js project, or planning to hire Vue.js developers, chances are you’ve come across the need for routing. Routing is an integral part of any web application, as it allows users to navigate from one page to another seamlessly, based on their interaction or actions. Vue.js, a popular choice among Vue.js developers, provides a robust solution for routing through Vue Router, a plugin that works hand-in-hand with the Vue.js core. This blog post will guide you on Vue.js routing, making it an easy task to accomplish even if you’re just starting to hire Vue.js developers for your projects.
What is a Vue Router?
Vue Router is the official routing library for Vue.js. It allows you to create a single-page application (SPA) with ease, mapping different components to various paths, managing page history, lazy-loading components, and more. It also supports nested routes and transition effects, adding more power to your Vue.js applications.
Let’s get started on how you can set up routing in your Vue.js application.
Setting Up Vue Router
First, install Vue Router in your application. If you are using npm, you can use the following command:
```bash npm install vue-router ```
After installation, import Vue Router into your main.js file and tell Vue.js to use it:
```javascript import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) ```
Configuring Routes
To start using Vue Router, you need to configure routes for your application. A route configuration is an object with `path` and `component` properties.
Here’s a simple example:
```javascript import Home from './components/Home.vue' import About from './components/About.vue' const routes = [ { path: '/home', component: Home }, { path: '/about', component: About } ] ```
The `path` is the URL where the route will be triggered, and the `component` is the Vue component that will be loaded when the route is matched.
Then, create a router instance and pass in your routes:
```javascript const router = new VueRouter({ routes // short for `routes: routes` }) ``` Finally, pass your router instance to your root Vue instance: ```javascript new Vue({ router, render: h => h(App) }).$mount('#app') ```
Now, whenever users navigate to /home or /about in your application, the corresponding component will be loaded.
Router-View and Router-Link
Vue Router provides two components that help you deal with your routes: `<router-view>` and `<router-link>`.
– `<router-view>`: This is a functional component that serves as a placeholder where matched components will be rendered.
– `<router-link>`: This component helps you navigate between pages in your application. It is equivalent to an `<a>` tag in HTML but with extra powers.
Here’s how to use them in your application:
```html <div id="app"> <p> <!-- use router-link component for navigation. --> <router-link to="/home">Go to Home</router-link> <router-link to="/about">Go to About</router-link> </p> <!-- route outlet --> <!-- component matched by the route will render here --> <router-view></router-view> </div> ```
Nested Routes
Vue Router allows you to nest your routes, providing more structuring of your components. Let’s say you have a User component that has Profile and Posts as its child components. Here’s how you can set up nested routes:
```javascript import User from './components/User.vue' import Profile from './components/Profile.vue' import Posts from './components/Posts.vue' const routes = [ { path : '/user', component: User, children: [ { path: 'profile', component: Profile }, { path: 'posts', component: Posts } ] } ] ```
Then, in your User component, use `<router-view>` to render the child components:
```html <template> <div> <h2>User Component</h2> <!-- child components will render here --> <router-view></router-view> </div> </template> ```
Programmatic Navigation
You might not always want to use `<router-link>` for navigation. Sometimes, you need to navigate to a route programmatically. Vue Router provides `this.$router.push` method for this:
```javascript // navigate to '/home' this.$router.push('/home') // with a path object this.$router.push({ path: '/home' }) // named route this.$router.push({ name: 'home' }) // with query, resulting in /home?plan=premium this.$router.push({ path: 'home', query: { plan: 'premium' }}) ```
Route Guards
Route guards or navigation guards allow you to protect routes from unauthorized access or confirm navigation away from a route. Vue Router provides several navigation guards, including `beforeEach`, `beforeResolve`, and `afterEach`.
Here’s an example of using `beforeEach` guard to check if a user is authenticated:
```javascript router.beforeEach((to, from, next) => { if (to.matched.some(record => record.meta.requiresAuth)) { if (!auth.isAuthenticated()) { next({ path: '/login', query: { redirect: to.fullPath } }) } else { next() } } else { next() // make sure to always call next()! } }) ```
In the above example, we check if any matched route record has `meta.requiresAuth` set to true. If yes, we then check if the user is authenticated. If the user isn’t authenticated, we redirect them to the login page; otherwise, we proceed to the next hook or render the route.
Lazy Loading Routes
To improve the initial load time of your application, Vue Router allows you to lazy load your routes. Instead of loading all routes and their corresponding components at once, components are only loaded when their routes are hit. Here’s how to do it:
```javascript const routes = [ { path: '/home', component: () => import('./components/Home.vue') }, { path: '/about', component: () => import('./components/About.vue') } ] ```
By using the dynamic import (`import()`), we are telling webpack to split our code and lazily load the Home and About components.
Conclusion
Vue.js routing, powered by Vue Router, provides a robust and flexible way to manage navigation in your Vue.js applications. This makes it an attractive feature for businesses looking to hire Vue.js developers. Vue Router is packed with features like nested routes, route guards, programmatic navigation, and lazy loading that help you build performant and user-friendly web applications.
With Vue Router, navigating through your Vue.js web application is indeed made easy! This demonstrates why the demand to hire Vue.js developers is growing, as they can leverage these advanced features for efficient application development.
Remember, the key to mastering Vue Router, like any other tool or library, is practice. This is something you can expect from skilled Vue.js developers. Try out different scenarios, play around with nested routes, and create complex navigation guards to see how much you can do with Vue Router.
Happy coding!
Table of Contents
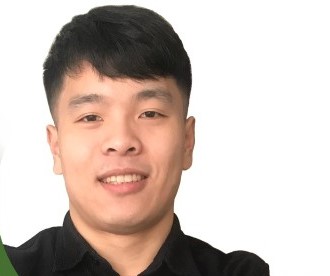
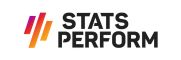