Demystifying Vue.js: An In-Depth Guide to Building Dynamic Web Applications
As JavaScript frameworks continue to rise in popularity, developers and businesses are increasingly adopting Vue.js for building user interfaces. In this surge of adoption, the demand to hire Vue.js developers has also seen a significant rise. Vue.js is powerful, flexible, and easy to adopt, making it a favorite choice for many developers and businesses alike. However, one often overlooked but critical factor in any web development project is SEO (Search Engine Optimization) and performance. To achieve the best results in these areas, Server-Side Rendering (SSR) comes into play, and skilled Vue.js developers are perfectly positioned to implement this effectively.
Understanding Server-Side Rendering (SSR)
Traditionally, JavaScript frameworks like Vue.js operate on the client side, meaning all the rendering of content, including HTML and CSS, happens in the user’s browser. However, this can lead to certain issues. For instance, search engines sometimes have difficulties in properly indexing websites that are heavily reliant on JavaScript. This can result in less-than-optimal SEO performance.
SSR can help solve these problems by rendering pages on the server before they reach the user’s browser. This is where the expertise of professional Vue.js developers can play a critical role. When you hire Vue.js developers, they utilize this technique to ensure that the server sends a fully rendered page to the client, which can then be instantly displayed. This can significantly enhance the performance of your application and improve SEO, as search engines can easily index the fully rendered content. Such SEO and performance improvements are key reasons why businesses are keen to hire Vue.js developers for their projects.
Vue.js and SSR
Vue.js provides built-in support for SSR, which is a major advantage for developers. In the following sections, we’ll delve deeper into how Vue.js and SSR work together to enhance SEO and performance.
Initial Setup
To get started, you’ll need to install Vue.js and Node.js. Node.js is crucial because SSR with Vue.js is achieved through a Node.js server.
First, install Vue.js using the Vue CLI:
```bash npm install -g @vue/cli vue create ssr-project ```
Next, install Node.js, if you haven’t already. You can download it directly from the official Node.js website.
Creating a Vue SSR Application
First, install the required Vue server-renderer and vue-router modules:
```bash npm install vue-server-renderer vue-router ```
Next, create two new files: `app.js` and `entry-server.js` in your src folder.
`app.js`:
```javascript import Vue from 'vue'; import App from './App.vue'; import { createRouter } from './router'; export function createApp() { const router = createRouter(); const app = new Vue({ router, render: h => h(App) }); return { app, router }; } ``` `entry-server.js`: ```javascript import { createApp } from './app'; export default context => { return new Promise((resolve, reject) => { const { app, router } = createApp(); router.push(context.url); router.onReady(() => { resolve(app); }, reject); }); }; ```
Here, `app.js` exports a function that can be used for both server and client entry points to bootstrap the app, and `entry-server.js` exports a server-side entry file that resolves a Promise which provides the app instance.
Configuring the Server
Create a new `server.js` file. This is where the server logic will be handled.
```javascript const express = require('express'); const fs = require('fs'); const server = express(); const { createBundleRenderer } = require('vue-server-renderer'); const serverBundle = require('./dist/vue-ssr-server-bundle.json'); const clientManifest = require('./dist/vue-ssr-client-manifest.json'); const renderer = createBundleRenderer(serverBundle, { runInNewContext: false, template: fs.readFileSync('./src/index.template.html', 'utf-8'), clientManifest }); server.get('*', (req, res) => { const context = { title: 'Vue.js and Server-Side Rendering', url: req.url }; renderer.renderToString(context, (err, html) => { if (err) { res.status(500).end('Internal Server Error'); return; } res.end(html); }); }); server.listen(8080); ```
In this script, we’re using Express.js to create a server, and then using Vue’s `createBundleRenderer` to create a renderer that can render our Vue application. The renderer requires the server bundle and client manifest, which we’ll create in the next step.
Building and Running the Application
For this, we will need to create two different webpack configurations – one for the client and one for the server. They can be in the same webpack config file but exported as an array. Below is a simple example:
```javascript // webpack.config.js const VueSSRClientPlugin = require('vue-server-renderer/client-plugin'); const VueSSRServerPlugin = require('vue-server-renderer/server-plugin'); const nodeExternals = require('webpack-node-externals'); const merge = require('webpack-merge'); const baseConfig = require('./webpack.base.config.js'); module.exports = [ merge(baseConfig, { entry: '/path/to/entry-client.js', plugins: [ new VueSSRClientPlugin() ] }), merge(baseConfig, { entry: '/path/to/entry-server.js', target: 'node', output: { libraryTarget: 'commonjs2' }, externals: nodeExternals({ whitelist: /\.css$/ }), plugins: [ new VueSSRServerPlugin() ] }) ] ```
This webpack configuration uses VueSSRClientPlugin and VueSSE Server Plugin to generate the `vue-ssr-client-manifest.json` and `vue-ssr-server-bundle.json` files that we mentioned in the server setup.
To build the application, run:
```bash npm run build ``` To start the server, run: ```bash node server.js ```
You should now be able to access your SSR Vue.js application at `http://localhost:8080`.
Benefits of SSR with Vue.js
There are a few key benefits of using SSR with Vue.js:
- Better SEO: Since SSR renders pages on the server, all content appears in the source code of the page, making it easier for search engine crawlers to index.
- Improved performance: With SSR, the browser can start displaying the received HTML while the JavaScript bundle is still downloading, leading to faster Time-To-Contentful-Paint.
- More compatibility: SSR applications can still run in browsers with disabled or limited JavaScript, increasing overall compatibility.
Conclusion
While implementing SSR with Vue.js can be a bit complex, it’s often worth the effort for the enhanced SEO and improved performance. This is where the expertise of those who hire Vue.js developers can really shine, leveraging their skills to create a highly efficient, SEO-friendly Vue.js application, a goal that is very achievable with the built-in SSR support that Vue.js provides.
Remember to carefully plan your application structure and consider how SSR will impact your app’s architecture. If you’re unsure, it may be beneficial to hire Vue.js developers who can provide insights and guidance. Also, consider that not every project may benefit from SSR; small projects or projects where SEO is not a concern may not need the added complexity of SSR. As always, evaluate your needs and choose the best approach for your specific situation. With the right team of Vue.js developers, you can achieve outstanding results. Happy coding!
Table of Contents
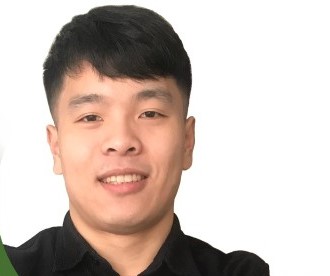
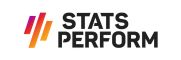