Vue.js and Webpack: Building Optimal Bundles for Your Web App
Vue.js is a popular JavaScript framework for building modern web applications, known for its ease of use and flexibility. Webpack is a powerful module bundler that optimizes and packages JavaScript files and other assets. Combining Vue.js with Webpack can greatly enhance the performance and maintainability of your web applications. This blog explores how to leverage these tools to build optimal bundles for your Vue.js projects.
Understanding Vue.js and Webpack
Vue.js provides a reactive and component-based architecture for developing user interfaces. It simplifies building interactive and dynamic web applications. Webpack, on the other hand, is a module bundler that takes your application code and assets, bundles them into a single or multiple optimized files, and handles various transformations like minification and transpilation.
Setting Up Vue.js with Webpack
To start using Vue.js with Webpack, you need to set up your development environment. Vue CLI provides a convenient way to initialize a Vue.js project with Webpack configuration.
Example: Creating a New Vue.js Project with Vue CLI
```bash Install Vue CLI globally npm install -g @vue/cli Create a new Vue.js project vue create my-vue-app Navigate to your project directory cd my-vue-app Start the development server npm run serve ```
Vue CLI automatically configures Webpack and provides a default setup that works well for most projects.
Optimizing Bundles with Webpack
Webpack offers several techniques for optimizing your bundles to ensure faster load times and better performance.
1. Code Splitting
Code splitting allows you to split your code into smaller chunks that can be loaded on demand. This improves the initial load time of your application.
Example: Implementing Code Splitting in Vue.js
```javascript // In your router/index.js import Vue from 'vue'; import Router from 'vue-router'; Vue.use(Router); export default new Router({ routes: [ { path: '/home', name: 'Home', component: () => import(/* webpackChunkName: "home" */ '../views/Home.vue'), }, { path: '/about', name: 'About', component: () => import(/* webpackChunkName: "about" */ '../views/About.vue'), }, ], }); ```
Using dynamic imports with `/* webpackChunkName: “chunk-name” */` helps Webpack generate separate bundles for each route.
2. Tree Shaking
Tree shaking removes unused code from your final bundle, reducing its size. This is particularly useful for libraries that import a lot of functions or components.
Example: Using Tree Shaking with ES6 Modules
Ensure you use ES6 module syntax (`import` and `export`) in your code. Webpack will automatically perform tree shaking when you build your project.
3. Minification and Compression
Minification reduces the size of your JavaScript and CSS files by removing unnecessary characters and whitespace. Compression can be done with tools like gzip or Brotli to further reduce the file size.
Example: Enabling Minification and Compression
Vue CLI already includes minification by default in production builds. You can configure additional compression by installing the `compression-webpack-plugin`:
```bash npm install compression-webpack-plugin --save-dev ```
Add the plugin to your `vue.config.js`:
```javascript // vue.config.js const CompressionPlugin = require('compression-webpack-plugin'); module.exports = { configureWebpack: { plugins: [ new CompressionPlugin({ algorithm: 'gzip', }), ], }, }; ```
Managing Dependencies with Webpack
Proper management of dependencies ensures that your bundles are not bloated with unnecessary libraries.
1. Analyzing Bundle Size
Use tools like `webpack-bundle-analyzer` to visualize the size of your bundles and understand what is contributing to them.
Example: Analyzing Bundle Size
```bash npm install webpack-bundle-analyzer --save-dev ```
Add the plugin to your `vue.config.js`:
```javascript // vue.config.js const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin; module.exports = { configureWebpack: { plugins: [ new BundleAnalyzerPlugin(), ], }, }; ```
Run the build command to open the analyzer:
```bash npm run build ```
2. Using External Libraries
For large libraries, consider using CDN links instead of bundling them into your application. Configure Webpack to treat these libraries as external dependencies.
Example: Configuring External Libraries
```javascript // vue.config.js module.exports = { configureWebpack: { externals: { lodash: '_', }, }, }; ```
Conclusion
Combining Vue.js with Webpack allows you to build highly optimized bundles for your web applications. By implementing code splitting, tree shaking, minification, and compression, and by effectively managing your dependencies, you can significantly enhance the performance and maintainability of your projects.
Further Reading:
Table of Contents
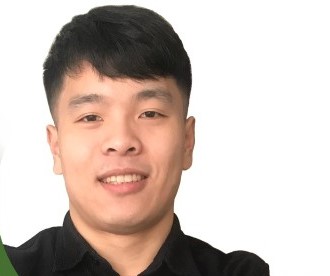
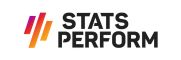