Mastering Yii: Essential Tips and Tricks for Effective Development
Yii is a powerful and popular PHP framework that simplifies web application development and promotes code reusability and maintainability. Whether you’re a beginner or an experienced developer, mastering Yii can take your development skills to the next level. In this blog, we’ll explore essential tips and tricks that will help you become a proficient Yii developer. From key concepts to best practices and code samples, we’ll cover everything you need to know to create efficient and scalable web applications with Yii.
Understanding Yii Framework Architecture
Before diving into Yii development, it’s crucial to understand its architecture. Yii follows the Model-View-Controller (MVC) pattern, separating business logic, presentation, and user interaction. We’ll explore Yii’s component-based architecture and its core components, such as models, views, and controllers.
Code Sample: Yii Controllers
php class PostController extends \yii\web\Controller { public function actionIndex() { $posts = Post::find()->all(); return $this->render('index', ['posts' => $posts]); } }
Effective Database Management with Yii
Yii provides robust database integration and offers multiple ways to interact with databases. We’ll cover Yii’s ActiveRecord pattern, database migrations, and techniques to optimize database queries for improved performance.
Code Sample: Yii ActiveRecord
php class Post extends \yii\db\ActiveRecord { public static function tableName() { return 'posts'; } }
Optimizing Performance with Caching Techniques
Yii incorporates various caching techniques to enhance application performance. We’ll explore Yii’s caching features, including page caching, data caching, and fragment caching, and learn when and how to use them effectively.
Code Sample: Yii Fragment Caching
php <?php if ($this->beginCache('widget_cache', ['duration' => 3600])): ?> <!-- Widget content goes here --> <?php $this->endCache(); endif; ?>
Creating Secure Applications with Yii
Security is a vital aspect of web application development. We’ll delve into Yii’s security features, such as input validation, output filtering, cross-site scripting (XSS) prevention, and protection against SQL injection attacks.
Code Sample: Yii Input Validation
php public function rules() { return [ ['username', 'required'], ['email', 'email'], // More validation rules... ]; }
Leveraging Extensions and Widgets
Yii has a vast ecosystem of extensions and widgets that can significantly simplify development. We’ll explore popular Yii extensions and widgets and learn how to integrate them into your projects to add functionality and improve the user experience.
Code Sample: Yii GridView Widget
php <?= \yii\grid\GridView::widget([ 'dataProvider' => $dataProvider, 'columns' => [ 'id', 'title', 'created_at', // More columns... ], ]) ?>
Implementing AJAX and RESTful APIs in Yii
Asynchronous JavaScript and XML (AJAX) and RESTful APIs are essential for building modern web applications. We’ll see how Yii makes it seamless to incorporate AJAX functionality and create RESTful APIs using Yii’s built-in tools and conventions.
Code Sample: Yii AJAX Request
php $.ajax({ url: '/site/data', method: 'POST', data: {param1: 'value1', param2: 'value2'}, success: function(response) { // Handle the response } });
Debugging and Logging in Yii
Debugging and logging are indispensable for identifying and resolving issues during development and in production environments. We’ll explore Yii’s debugging and logging capabilities, including debugging toolbar, logging targets, and custom log messages.
Code Sample: Yii Logging
php Yii::info('This is an informational message.', 'app\components\Logger'); Yii::error('An error occurred.', 'app\components\Logger');
Testing and Test-Driven Development in Yii
Yii offers excellent support for testing, enabling developers to build reliable and robust applications. We’ll dive into Yii’s testing framework and learn how to write unit tests, functional tests, and acceptance tests using Yii’s testing utilities.
Code Sample: Yii Unit Test
php class PostTest extends \yii\codeception\TestCase { public function testCreate() { $post = new Post(); $post->title = 'Test Title'; $post->content = 'Test Content'; $this->assertTrue($post->save()); } }
Optimizing Yii Applications for SEO
Search Engine Optimization (SEO) plays a crucial role in driving organic traffic to websites. We’ll explore techniques and Yii features to optimize your applications for better search engine visibility, including URL management, meta tags, and structured data.
Deployment and Maintenance Tips
Finally, we’ll cover best practices for deploying Yii applications, including server configuration, performance optimization, and ongoing maintenance tasks to ensure your Yii application runs smoothly in production.
Conclusion
By mastering Yii and applying the essential tips and tricks discussed in this blog, you’ll become a proficient Yii developer. You’ll be equipped with the knowledge and skills to build efficient, secure, and scalable web applications with ease. Keep exploring Yii’s documentation, participate in the vibrant Yii community, and continue refining your skills to become an expert in Yii development. Happy coding!
Table of Contents
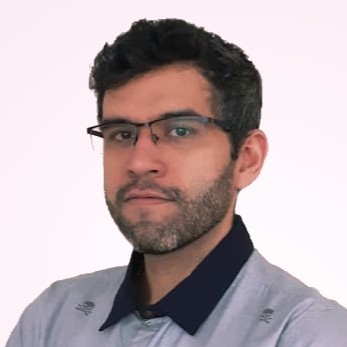
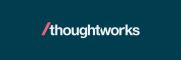