Hiring Yii Developers: A Comprehensive Interview Questions Guide
In the realm of web application development, Yii has gained recognition as a powerful PHP framework that enables the creation of robust and feature-rich applications. To navigate the process of hiring Yii developers effectively, this guide equips you with essential interview questions and strategies to identify top-tier candidates with the technical expertise, problem-solving acumen, and Yii proficiency your team requires.
Table of Contents
1. How to Hire Yii Developers
Embark on your journey of hiring Yii developers with these strategic steps:
- Job Requirements: Define specific job prerequisites outlining the desired skills and experience for your Yii development role.
- Search Channels: Utilize job boards, online platforms, and developer communities to identify potential Yii developer candidates.
- Screening: Evaluate candidates based on their Yii proficiency, PHP knowledge, and supplementary skills.
- Technical Assessment: Develop a comprehensive technical evaluation to assess candidates’ coding abilities, understanding of Yii’s MVC architecture, and problem-solving capabilities.
2. Key Skills to Look for in Yii Developers
When evaluating Yii developers, focus on these core skills:
- Yii Framework Proficiency: An in-depth understanding of Yii’s features, components, and conventions for building efficient and scalable web applications.
- PHP Expertise: Proficiency in PHP programming, as Yii is a PHP framework, and familiarity with PHP best practices.
- MVC Architecture: Knowledge of the Model-View-Controller (MVC) architectural pattern and its implementation in Yii for separating concerns and promoting code organization.
- Database Integration: Experience in working with databases, including Yii’s ActiveRecord implementation, and proficiency in SQL queries and database design.
- Security Practices: Understanding of security measures such as input validation, output escaping, authentication, and authorization to ensure secure applications.
- Version Control: Proficiency in using version control systems like Git to collaborate effectively with team members on codebases.
3. Yii Developer Hiring Process Overview
Here’s a high-level overview of the Yii developer hiring process:
3.1 Define Job Requirements and Skillsets
Lay a strong foundation by outlining clear job requirements, specifying the skills and experience you’re seeking in Yii developer candidates.
3.2 Craft Compelling Job Descriptions
Create engaging job descriptions that accurately convey the responsibilities and opportunities associated with the Yii development role.
3.3 Develop Yii Developer Interview Questions
Design a comprehensive set of interview questions covering Yii intricacies, MVC concepts, database interactions, and web development technologies.
4. Sample Yii Developer Interview Questions and Answers
Explore these sample questions and answers to assess candidates’ Yii skills:
Q1. Explain the MVC architecture and its role in Yii framework. How does Yii implement the MVC pattern?
A: The MVC architecture separates an application into three components: Model (data), View (presentation), and Controller (user interaction). Yii implements MVC by providing classes like CActiveRecord for models, CViewRenderer for views, and CController for controllers.
Q2. Describe the purpose of Yii’s ActiveRecord. How can you use it to interact with databases and perform CRUD operations?
A: Yii’s ActiveRecord is an implementation of the Active Record design pattern. It allows developers to interact with databases using object-oriented syntax. You can define models representing database tables and use methods like find(), save(), and delete() to perform CRUD operations.
Q3. Implement a Yii controller action that fetches a list of users from the database and renders them using a Yii view.
class UserController extends CController { public function actionIndex() { $users = User::model()->findAll(); $this->render('index', array('users' => $users)); } }
Q4. Explain the concept of Yii widgets. How can you use built-in widgets and create custom widgets in Yii?
A: Yii widgets are reusable UI components that encapsulate functionality and presentation. Built-in widgets like CGridView and CListView can be used to render data in tabular or list format. You can create custom widgets by extending CWidget and implementing the renderContent() method.
Q5. What is Yii’s approach to handling form input validation? How can you validate user inputs in Yii forms?
A: Yii provides a validation framework that allows you to define validation rules for form inputs. You can specify rules in model classes using the rules()
method. When the form is submitted, Yii automatically validates the inputs based on these rules.
Q6. Describe Yii’s approach to handling internationalization (i18n) and localization (l10n). How can you translate text in Yii applications?
A: Yii supports internationalization and localization through message translation. You can use Yii’s Yii::t()
method to translate messages based on the application’s configured language and locale.
Q7. Explain the concept of Yii modules. How can you use modules to organize and modularize code in Yii applications?
A: Yii modules are self-contained components that encapsulate functionality and can be reused across applications. They allow you to organize code into separate directories, with their own controllers, models, views, and assets.
Q8. What are Yii filters? How can you use filters to implement cross-cutting concerns such as access control or authentication?
A: Yii filters are pre- and post-action methods that can be applied to controller actions. They are used to implement cross-cutting concerns like access control, authentication, and caching. Filters can be applied globally or to specific actions.
Q9. Describe the process of handling authentication and authorization in Yii applications. How can you use Yii’s built-in RBAC system for access control?
A: Yii provides built-in support for authentication and authorization. Authentication involves verifying user identities, while authorization determines what actions a user is allowed to perform. Yii’s RBAC (Role-Based Access Control) system allows you to define roles, permissions, and access rules for users.
Q10. How can you optimize the performance of a Yii application? Mention some strategies and techniques.
A: Yii performance optimization involves techniques such as caching, database query optimization, using Yii’s built-in pagination and data providers, enabling Gzip compression, and using a content delivery network (CDN) for assets.
Q11. Explain the concept of Yii migrations and how they are used to manage database schema changes. Provide an example of creating a migration to add a new table.
A: Yii migrations are a way to manage database schema changes in a versioned and systematic manner. They allow developers to define and apply changes to the database structure. Here’s an example of creating a migration to add a new table:
// Create a new migration file using the Yii command line tool yii migrate/create create_new_table // In the generated migration file class m210818_123456_create_new_table extends CDbMigration { public function up() { $this->createTable('new_table', array( 'id' => 'pk', 'name' => 'string NOT NULL', 'created_at' => 'datetime', )); } public function down() { $this->dropTable('new_table'); } }
Q12. Describe the use of Yii’s Active Record pattern. Provide an example of defining an Active Record model for a “User” table with basic CRUD operations.
A: Yii’s Active Record pattern allows developers to work with database records using an object-oriented approach. Here’s an example of defining an Active Record model for a “User” table and performing basic CRUD operations:
// User.php (Active Record model) class User extends CActiveRecord { public static function model($className=__CLASS__) { return parent::model($className); } public function tableName() { return 'user'; } // Perform CRUD operations public function createNewUser($attributes) { $user = new User; $user->attributes = $attributes; return $user->save(); } public function updateUser($id, $attributes) { $user = User::model()->findByPk($id); if ($user !== null) { $user->attributes = $attributes; return $user->save(); } return false; } public function deleteUser($id) { $user = User::model()->findByPk($id); if ($user !== null) { return $user->delete(); } return false; } public function getUserById($id) { return User::model()->findByPk($id); } }
Q13. Explain how Yii handles input validation using model rules. Provide an example of defining validation rules for a “Post” model.
A: Yii uses model rules to define input validation rules, ensuring that data entering the application is valid. Here’s an example of defining validation rules for a “Post” model:
// Post.php (Active Record model) class Post extends CActiveRecord { public function rules() { return array( array('title, content', 'required'), array('title', 'length', 'max' => 100), array('content', 'length', 'max' => 500), ); } }
Q14. Describe the purpose of Yii behaviors. Provide an example of creating a behavior that automatically timestamps the “created_at” and “updated_at” fields for models.
A: Yii behaviors allow developers to attach reusable functionality to models. They are a way to modularize and share common functionalities. Here’s an example of creating a behavior that automatically timestamps the “created_at” and “updated_at” fields for models:
// TimestampBehavior.php class TimestampBehavior extends CActiveRecordBehavior { public function beforeSave($event) { if ($this->getOwner()->isNewRecord) { $this->getOwner()->created_at = new CDbExpression('NOW()'); } $this->getOwner()->updated_at = new CDbExpression('NOW()'); return parent::beforeSave($event); } } // Usage in a model class Post extends CActiveRecord { public function behaviors() { return array( 'timestamp' => array( 'class' => 'TimestampBehavior', ), ); } }
5. Hiring Yii Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your Yii development project’s specifics, preferred skills, and desired experience levels.
Step 2: Identify the Ideal Fit: Within a short timeframe, CloudDevs presents you with carefully selected Yii developers from their network of pre-vetted professionals. Review their profiles and select the candidate whose expertise aligns seamlessly with your project’s goals.
Step 3: Begin a Trial Engagement: Engage in discussions with your chosen developer to ensure a smooth integration into your team. Once satisfied, formalize the collaboration and embark on a risk-free trial period spanning one week.
By leveraging the expertise of CloudDevs, you can seamlessly identify and hire top-tier Yii developers, ensuring your team possesses the skills necessary to craft remarkable web applications.
6. Conclusion
Armed with these comprehensive interview questions and insights, you’re well-prepared to assess Yii developers comprehensively. Whether you’re building enterprise applications or dynamic web solutions, securing the right Yii developers through CloudDevs is essential for achieving success in your PHP development projects.
Table of Contents
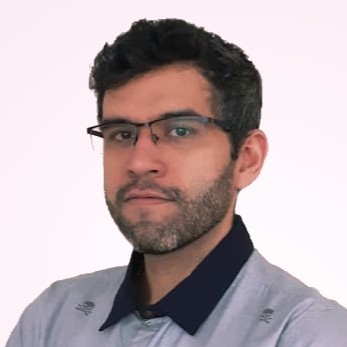
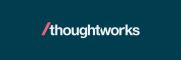