Yii Performance Optimization: Boosting Speed and Efficiency
Yii is a high-performance, component-based PHP framework for developing modern web applications. While Yii is known for its speed, there’s always room for improvement, especially for larger applications. This article explores various techniques to optimize your Yii applications, ensuring they run faster and more efficiently.
Setting Up Yii for Optimal Performance
To get started with performance optimization in Yii, you should first ensure your Yii installation is configured correctly for production use. This includes setting up caching, optimizing database interactions, and minimizing resource usage.
Example: Configuring Yii for Production
Modify your `config/web.php` to ensure your application is set up for production:
```php 'components' => [ 'db' => [ 'class' => 'yii\db\Connection', 'dsn' => 'mysql:host=localhost;dbname=yourdbname', 'username' => 'yourusername', 'password' => 'yourpassword', 'charset' => 'utf8', 'enableSchemaCache' => true, 'schemaCacheDuration' => 3600, 'schemaCache' => 'cache', ], 'cache' => [ 'class' => 'yii\caching\FileCache', ], ], ```
Enabling Caching
Caching is one of the most effective ways to improve application performance. Yii supports several caching mechanisms, including data caching, fragment caching, and page caching.
Example: Implementing Data Caching in Yii
```php $data = Yii::$app->cache->get('myData'); if ($data === false) { // The data is not found in the cache, calculate it from scratch $data = $this->calculateMyData(); // Store the data in cache for future requests Yii::$app->cache->set('myData', $data, 3600); // Cache for 1 hour } return $data; ```
Optimizing Database Queries
Efficient database queries are crucial for application performance. Yii provides Active Record for database interactions, but for performance-critical operations, you should consider using Query Builder or raw SQL queries.
Example: Using Query Builder for Optimized Queries
```php $rows = (new \yii\db\Query()) ->select(['id', 'username']) ->from('user') ->where(['status' => 1]) ->limit(10) ->all(); ```
Minimizing Resource Usage
Minimizing the number of resources loaded on each request can significantly boost performance. This includes reducing the size of JavaScript and CSS files, as well as optimizing images.
Example: Minifying JavaScript and CSS
You can use Yii’s Asset Manager to combine and minify CSS and JavaScript files. In your `config/web.php`:
```php 'components' => [ 'assetManager' => [ 'bundles' => [ 'yii\web\JqueryAsset' => [ 'sourcePath' => null, 'js' => [ '//ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', ] ], ], ], ], ```
Leveraging Lazy Loading and Eager Loading
Yii provides both lazy loading and eager loading for fetching related data. Using eager loading (`with()`) can help reduce the number of database queries, improving performance.
Example: Using Eager Loading to Improve Performance
```php $orders = Order::find()->with('customer')->all(); ```
Using Yii Debug Toolbar
The Yii Debug Toolbar is a powerful tool for profiling your application and identifying performance bottlenecks. It provides detailed information about database queries, memory usage, and request times.
Implementing Opcode Caching
Opcode caching can significantly reduce the execution time of PHP scripts by caching the compiled bytecode of PHP scripts.
Example: Enabling OPcache
To enable OPcache, add the following to your `php.ini`:
```ini opcache.enable=1 opcache.memory_consumption=128 opcache.interned_strings_buffer=8 opcache.max_accelerated_files=4000 opcache.revalidate_freq=60 ```
Conclusion
By implementing these performance optimization techniques, you can significantly improve the speed and efficiency of your Yii applications. From caching and optimized database queries to minimizing resource usage and leveraging Yii’s built-in tools, these strategies will help you create faster, more responsive applications.
Further Reading:
Table of Contents
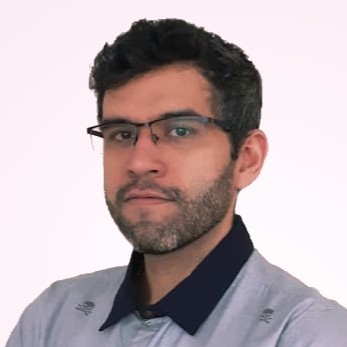
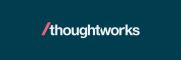