Mastering Delegates and Events in C#
In the world of C# programming, delegates and events are powerful tools that allow you to achieve loose coupling, enhance extensibility, and enable event-driven programming. Whether you’re a beginner or an experienced developer, mastering delegates and events is essential to building robust and scalable applications.
In this blog post, we’ll explore delegates and events in depth, starting with the fundamentals and gradually moving towards advanced concepts and best practices. By the end, you’ll have a solid understanding of how delegates and events work, how to use them effectively, and how they can take your C# programming skills to the next level.
Understanding Delegates:
1. Introduction to Delegates:
Delegates in C# are objects that can hold references to methods with a compatible signature. They provide a way to treat methods as first-class citizens, allowing you to pass them as parameters, store them in variables, and invoke them dynamically. Delegates serve as powerful abstractions for callbacks and event handling.
2. Defining and Using Delegates:
To define a delegate, you need to declare a delegate type that specifies the signature of the methods it can reference. You can then create an instance of the delegate and associate it with a method. When the delegate is invoked, all the associated methods are executed.
csharp // Define a delegate type delegate void MyDelegate(int x, int y); // Create an instance of the delegate MyDelegate myDelegate = AddNumbers; // Associate methods with the delegate myDelegate += SubtractNumbers; myDelegate += MultiplyNumbers; // Invoke the delegate myDelegate(10, 5); // Output: 15, 5, 50
3. Multicast Delegates:
C# delegates support multicast, which means they can hold references to multiple methods. When a multicast delegate is invoked, all the associated methods are executed in the order they were added. This feature allows you to create powerful event systems and implement the observer pattern.
4. Anonymous Methods and Lambda Expressions:
Anonymous methods and lambda expressions provide concise syntax for defining delegate methods inline, without explicitly declaring a separate method. They are especially useful when you need to pass a short piece of code as a callback.
csharp // Using an anonymous method MyDelegate myDelegate = delegate (int x, int y) { Console.WriteLine(x + y); }; // Using a lambda expression MyDelegate myDelegate = (x, y) => Console.WriteLine(x + y);
Working with Events
1. Introduction to Events:
Events build upon delegates and provide a standardized way to implement the publisher-subscriber pattern. Publishers raise events, and subscribers listen to those events and respond accordingly. Events facilitate loose coupling and enable components to communicate without having explicit knowledge of each other.
2. Declaring and Raising Events:
To declare an event, you need to define a delegate type that represents the event’s signature. The event is then declared using the event keyword and associated with an instance of the delegate. When the event is raised, all the subscribed event handlers are called.
csharp // Define an event delegate type delegate void MyEventHandler(object sender, EventArgs e); // Declare an event event MyEventHandler MyEvent; // Raise the event MyEvent?.Invoke(this, EventArgs.Empty);
3. Subscribing to Events:
To subscribe to an event, you need to provide a method that matches the event delegate’s signature. You can subscribe to an event using the += operator, and unsubscribe using the -= operator. It’s essential to manage subscriptions properly to prevent memory leaks.
csharp // Subscribe to an event myObject.MyEvent += MyEventHandlerMethod; // Unsubscribe from an event myObject.MyEvent -= MyEventHandlerMethod;
4. Custom Event Arguments:
Events often provide additional data to subscribers using event arguments. You can define custom event argument classes by deriving from the EventArgs base class. Custom event arguments allow you to pass relevant information to event handlers.
Advanced Topics:
1. Covariance and Contravariance:
C# supports covariance and contravariance for delegate types, which enable more flexible assignment compatibility. Covariance allows assigning a method with a more derived return type, while contravariance allows assigning a method with more general parameter types. These features enhance code reuse and flexibility.
2. Delegate Chaining and Invocation Lists:
Multicast delegates can be combined and invoked using the + and Invoke() operators, respectively. Understanding delegate chaining and invocation lists is crucial to building extensible systems with multiple subscribers and handling complex scenarios.
3. Event Accessors and Event Handlers:
In addition to raising events, you can provide accessors for events to control subscription and unsubscription. Event handlers are methods that handle events and follow a specific signature. By convention, event handlers have the suffix “Handler” to differentiate them from regular methods.
4. Event Patterns in C# 8 and Above:
C# 8 introduced event patterns, which provide a concise and expressive syntax for working with events. The add and remove accessors are replaced with add and remove keywords, making event declaration and handling more intuitive.
Best Practices:
1. Naming Conventions:
Follow established naming conventions to make your code more readable and maintainable. Use descriptive names for delegates, events, and event handlers to convey their purpose clearly.
2. Choosing Between Delegates and Interfaces:
Consider the requirements of your application when deciding between delegates and interfaces. Delegates excel at defining callbacks, while interfaces provide a more general contract. Choose the appropriate approach based on your design goals.
3. Avoiding Memory Leaks with Weak Event Patterns:
When subscribing to events, be cautious of potential memory leaks, especially in long-lived objects. Weak event patterns, such as using WeakReference or WeakEventManager, can help mitigate this issue by allowing automatic garbage collection of event handlers.
4. Unit Testing Delegates and Events:
Ensure the correctness of your delegates and events by writing unit tests. Test the invocation of delegates, event subscriptions, event raising, and event handler behavior to verify the expected functionality.
Conclusion
Delegates and events are powerful constructs in C# that enable flexible and extensible programming. By mastering delegates and events, you can build robust applications that adhere to the principles of loose coupling, event-driven design, and separation of concerns. With the knowledge gained from this comprehensive guide, you’re well-equipped to leverage the full potential of delegates and events in your C# projects. Happy coding!
Table of Contents
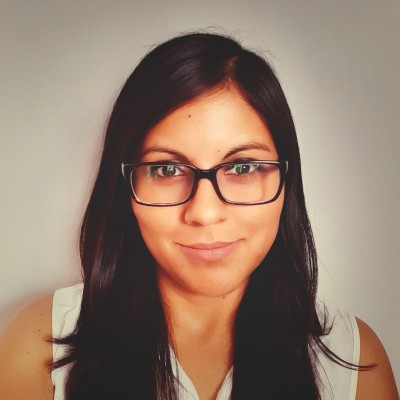
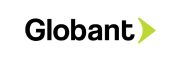