What is dependency injection in C#?
Dependency Injection (DI) is a software design pattern widely used in C# and other programming languages. It is a technique for achieving the Inversion of Control (IoC) principle, which promotes loose coupling and better maintainability in your code.
In DI, a class or component’s dependencies (e.g., other objects or services it relies on) are provided or “injected” from the outside, rather than being created or managed internally. This approach has several advantages:
- Decoupling: By injecting dependencies, a class is not tightly bound to the concrete implementations of its dependencies. This decoupling allows for easier unit testing and makes your code more flexible and adaptable to change.
- Testability: DI facilitates unit testing because you can easily substitute real dependencies with mock objects or stubs during testing. This ensures that tests focus on the class’s behavior rather than the behavior of its dependencies.
- Reusability: With DI, dependencies can be reused across different parts of your application. This reusability promotes a more modular and maintainable codebase.
- Configurability: You can configure the injection of different implementations or configurations of dependencies at runtime or through configuration files, making your application more adaptable and configurable.
- Maintainability: DI simplifies the management of dependencies, making it easier to modify or extend your application without causing widespread code changes.
In C#, DI is commonly implemented using frameworks and libraries like Microsoft’s built-in Dependency Injection framework, third-party libraries such as Autofac, Unity, or Castle Windsor, or even through simple constructor injection without any third-party tools. Here’s a basic example of constructor injection:
```csharp public class OrderService { private readonly IOrderRepository _repository; public OrderService(IOrderRepository repository) { _repository = repository; } public void PlaceOrder(Order order) { // Use _repository to interact with the data store _repository.Add(order); } } ```
In this example, `OrderService` relies on an `IOrderRepository` interface, and the concrete implementation of the repository is injected into the service through its constructor. This approach adheres to the principles of DI and allows for flexibility and maintainability in your codebase.
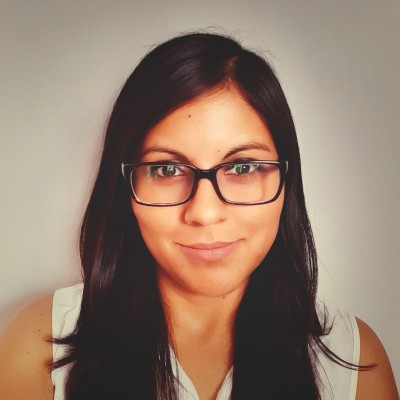
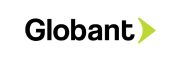