What is dependency injection in ASP.NET Core?
Dependency injection (DI) is a fundamental concept in ASP.NET Core, as well as in modern software development in general. It plays a crucial role in managing the dependencies and improving the testability, maintainability, and flexibility of your ASP.NET Core applications.
At its core, dependency injection is a design pattern that facilitates the separation of concerns by allowing you to inject the dependencies of a class rather than having the class create them internally. In ASP.NET Core, this pattern is widely used to provide services, components, or objects to other parts of your application in a loosely coupled manner.
Here’s how dependency injection works in ASP.NET Core:
- Service Registration:
– In the `Startup.cs` file of your ASP.NET Core application, you register your application’s services in the `ConfigureServices` method using the built-in dependency injection container provided by ASP.NET Core.
```csharp public void ConfigureServices(IServiceCollection services) { services.AddTransient<IMyService, MyService>(); // Register other services... } ```
In this example, we’re registering `IMyService` as an interface with its corresponding implementation `MyService`. ASP.NET Core will manage the lifecycle of `MyService` and provide it when requested.
- Dependency Injection:
– In your controllers, services, or other parts of the application, you can request dependencies via constructor injection or method injection.
```csharp public class MyController : ControllerBase { private readonly IMyService _myService; public MyController(IMyService myService) { _myService = myService; } // Use _myService in controller actions... } ```
By injecting the `IMyService` dependency into the controller’s constructor, ASP.NET Core will automatically provide an instance of `MyService` when creating the `MyController` instance.
- Benefits of Dependency Injection:
– Testability: Dependency injection makes it easy to replace real services with mock or test implementations for unit testing.
– Flexibility: You can change the behavior of your application by swapping implementations without modifying the consuming classes.
– Maintainability: Dependencies are clearly defined and centralized in the `Startup.cs` file, making it easier to manage and update them.
– Decoupling: Your classes become loosely coupled because they don’t create their own dependencies, reducing the risk of tight coupling and simplifying future changes or extensions.
Dependency injection is a powerful technique in ASP.NET Core that promotes clean, modular, and maintainable code by decoupling components and providing a mechanism for managing and injecting dependencies into your application’s classes.
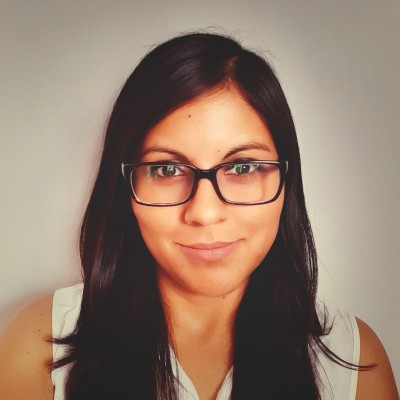
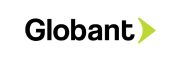