What is Dependency Injection in ASP.NET Core Razor Pages?
Dependency Injection (DI) in ASP.NET Core Razor Pages is a design pattern and framework feature that plays a crucial role in building maintainable, testable, and scalable web applications. It simplifies the management of object dependencies and promotes loose coupling between different components of your application.
In Razor Pages, DI is used to provide instances of services or dependencies to your Razor Page classes, allowing you to access and utilize these services within your page logic. Here’s how it works:
- Service Registration: The first step in using DI is to register your services with the built-in IoC (Inversion of Control) container provided by ASP.NET Core. This is typically done in your application’s `Startup.cs` file within the `ConfigureServices` method. For example:
```csharp services.AddTransient<IMyService, MyService>(); ```
In this example, we register an interface `IMyService` and associate it with the concrete implementation `MyService`. ASP.NET Core will handle the creation and management of instances of `MyService`.
- Dependency Injection: In your Razor Page class, you can request instances of these registered services through constructor injection. The IoC container will automatically provide the required dependencies when creating an instance of your Razor Page. For example:
```csharp public class MyPageModel : PageModel { private readonly IMyService _myService; public MyPageModel(IMyService myService) { _myService = myService; } // Use _myService within your page logic } ```
Here, `IMyService` is injected into `MyPageModel`’s constructor, making it available for use throughout the page.
- Benefits: Dependency Injection in Razor Pages offers several advantages. It promotes a separation of concerns, making your code more modular and easier to maintain. It also facilitates unit testing by allowing you to easily substitute real services with mock implementations during testing. Additionally, it enables better scalability as you can swap out implementations or change behavior by modifying service registrations.
Dependency Injection in ASP.NET Core Razor Pages is a powerful tool for managing dependencies and promoting clean architecture in your web applications. It simplifies the process of obtaining and using services within your Razor Pages, making your code more modular, testable, and maintainable.
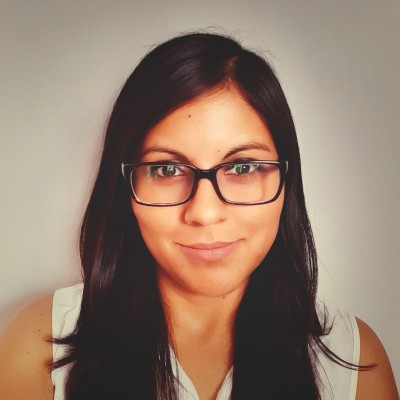
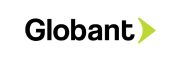