Improve Software Reliability with Effective Error Management in C#
When developing software in C#, it’s a good bet that at some point, something will go wrong. It’s not a matter of if, but when. When errors occur, they can be fatal to the software, leading to crashes, data corruption, and general instability. Therefore, it’s crucial that software developers, especially when you’re looking to hire C# developers, adopt a robust approach to error handling. Their familiarity with effective strategies for handling exceptions in C# can significantly impact the reliability of your software.
In this blog post, we’ll explore these best practices and strategies for exception handling in C#, providing valuable insights for anyone looking to hire C# developers.
Understanding Exceptions in C#
An exception is a runtime error that disrupts the normal flow of a program. It could be anything from a NullReferenceException, indicating that you’re trying to access an object that doesn’t exist, to an IOException, signifying an error occurred while trying to read or write from a file.
C# provides a structured approach to handling these exceptions, offering a way to detect and correct them without causing a complete crash. The basics of exception handling in C# revolves around four keywords: try, catch, finally, and throw.
The Try-Catch-Finally Construct
– Try: The code that could potentially throw an exception is enclosed within a try block. If an exception occurs within this block, it is thrown.
– Catch: This block catches the exception thrown from the try block. You can specify the type of exception you are catching, or catch all exceptions.
– Finally: This block is executed regardless of whether an exception occurs. It is typically used for cleaning up resources such as file handles, database connections, etc.
– Throw: This keyword is used to raise an exception manually.
Here is an example:
```csharp try { // Code that could throw an exception } catch (SpecificException ex) { // Handle the specific exception } catch (Exception ex) { // Handle any other exceptions } finally { // Clean up code, always executed }
Best Practices and Strategies
- Use Specific Exceptions
While it’s possible to catch all exceptions with the base Exception class, it’s usually not the best approach. Catching specific exceptions allows you to handle different error scenarios in a more tailored way.
```csharp try { // Code that could throw an exception } catch (FileNotFoundException ex) { // Handle file not found } catch (IOException ex) { // Handle other I/O errors }
- Don’t Catch Exceptions You Can’t Handle
If you cannot meaningfully handle an exception, it is better to let it propagate up to a higher level in your application where it can be dealt with appropriately.
- Avoid Empty Catch Blocks
An empty catch block can make it difficult to debug an application because it effectively swallows the exception and does nothing. If you catch an exception, ensure that you either handle it appropriately or log it for future debugging.
- Use the Finally Block for Cleanup
Any cleanup code should be put in the final block to ensure it is executed, regardless of whether an exception was thrown.
```csharp FileStream file = null; try { file = new FileStream("file.txt", FileMode.Open); // Use the file } catch (FileNotFoundException ex) { // Handle file not found } finally { if (file != null) { file.Close(); } }
- Use Using for Resource Management
For any object that implements the IDisposable interface, use the using statement. It automatically takes care of object disposal, even in the case of exceptions, eliminating the need for a final block.
```csharp using (FileStream file = new FileStream("file.txt", FileMode.Open)) { // Use the file }
This is equivalent to:
```csharp FileStream file = null; try { file = new FileStream("file.txt", FileMode.Open); // Use the file } finally { if (file != null) { file.Close(); } }
- Exception Propagation
You can allow exceptions to propagate up the call stack by not catching them, or by catching and then rethrowing them. If you catch and rethrow, use the `throw;` statement without an object to preserve the stack trace.
```csharp try { // Code that could throw an exception } catch (Exception ex) { // Perform some action before re-throwing throw; }
- Custom Exceptions
In certain cases, you may need to create custom exceptions to better represent the errors in your application. When you do, make sure to inherit from the Exception base class or one of its subclasses.
```csharp public class CustomException : Exception { public CustomException() { } public CustomException(string message) : base(message) { } public CustomException(string message, Exception inner) : base(message, inner) { } }
- Logging Exceptions
Always log exceptions. This includes the exception message, stack trace, and any inner exceptions. This information can be crucial for debugging and troubleshooting errors in a live system.
Conclusion
Proper exception handling is a critical aspect of developing reliable and robust software. This is a key consideration when you plan to hire C# developers, as their ability to implement these strategies and best practices can significantly impact the stability of your applications. By incorporating these guidelines in your C# projects, you can handle errors more effectively, resulting in applications that are not only more robust but also easier to debug and maintain.
Remember, the key to good exception handling is to anticipate and plan for potential errors. This should be a core skill of any C# developers you hire – they must be able to catch and handle only those exceptions they can effectively deal with, allow others to propagate, and always clean up after themselves. As you proceed with your future C# endeavors, whether it’s developing in-house or choosing to hire C# developers, ensure that these best practices are closely followed. Good luck!
Table of Contents
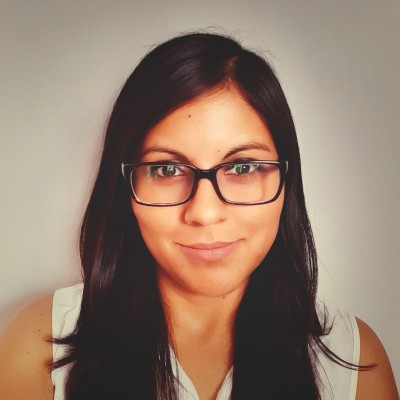
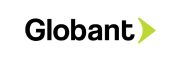