C# and Entity Framework: Building Data-Driven Applications
In today’s digital era, data is at the heart of every application. Whether you’re building a small business website or a large enterprise system, the ability to effectively manage and manipulate data is crucial. This is where C# and Entity Framework shine. In this blog post, we will dive into the world of C# and Entity Framework, exploring the best practices and techniques for building data-driven applications that are both powerful and efficient.
Understanding Data-Driven Applications
Data-driven applications are software systems that rely on data as their primary source of input, output, and decision-making. These applications store, retrieve, and manipulate data to provide meaningful insights, drive business processes, and deliver value to end-users. C# and Entity Framework together provide a robust foundation for building such applications.
Introduction to C# and Entity Framework
1. What is C#?
C# (pronounced C sharp) is a powerful, object-oriented programming language developed by Microsoft. It is widely used for building a variety of applications, including web, desktop, mobile, and cloud-based systems. C# offers a rich set of features, strong type safety, and seamless integration with the .NET Framework.
2. What is Entity Framework?
Entity Framework (EF) is an object-relational mapping (ORM) framework for .NET applications. It enables developers to work with databases using strongly-typed objects and provides a higher level of abstraction for data access. With Entity Framework, you can create, retrieve, update, and delete data without writing complex SQL queries manually.
Getting Started with C# and Entity Framework
1. Setting up the Development Environment:
To begin building data-driven applications with C# and Entity Framework, you’ll need to set up your development environment. Install Visual Studio, the Integrated Development Environment (IDE) for C#, and ensure that the Entity Framework package is available.
2. Creating the Data Model:
In Entity Framework, the data model represents the structure of your database. You define entities (classes) that map to database tables and relationships between them. Use attributes or Fluent API to configure the model, specifying properties, keys, and associations.
Code Sample:
csharp public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } }
3. Connecting to the Database:
Entity Framework supports various database providers, including SQL Server, MySQL, and SQLite. Configure the database connection string in your application’s configuration file and establish a connection to the database.
Code Sample:
csharp using Microsoft.EntityFrameworkCore; public class MyDbContext : DbContext { protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { optionsBuilder.UseSqlServer("your_connection_string"); } public DbSet<Product> Products { get; set; } }
Querying Data with LINQ
1. Introduction to LINQ:
Language Integrated Query (LINQ) allows you to query and manipulate data using a consistent syntax within C#. With LINQ, you can express complex data operations using a set of query operators, making it easier to work with data in a strongly-typed manner.
2. LINQ Query Syntax vs. Method Syntax:
LINQ provides two syntaxes: query syntax and method syntax. The query syntax resembles SQL, while the method syntax uses lambda expressions. Both syntaxes are equivalent and offer flexibility in writing queries.
Code Sample (Query Syntax):
var expensiveProducts = from p in dbContext.Products where p.Price > 100 select p;
-
- <li style=”font-weight: 400;” aria-level=”1″>
3. Filtering, Sorting, and Projection:
LINQ supports various operators for filtering, sorting, and projecting data. Use operators like Where, OrderBy, and Select to perform these operations effectively.
Code Sample (Method Syntax):
csharp var cheapProductNames = dbContext.Products .Where(p => p.Price < 50) .OrderBy(p => p.Name) .Select(p => p.Name);
Working with Data Relationships
1. Understanding Associations:
Data relationships define how entities are related to each other. In Entity Framework, you can represent associations using navigation properties and foreign keys. This allows you to query and manipulate related data efficiently.
2. One-to-One Relationships:
A one-to-one relationship occurs when one entity is associated with exactly one related entity. Use navigation properties and foreign keys to define and work with one-to-one relationships in Entity Framework.
3. One-to-Many Relationships:
A one-to-many relationship occurs when one entity is associated with multiple related entities. Use collection navigation properties and foreign keys to establish and handle one-to-many relationships.
4. Many-to-Many Relationships:
A many-to-many relationship occurs when multiple entities are associated with multiple related entities. To represent this relationship in Entity Framework, you create a junction table and define navigation properties accordingly.
Advanced Querying and Performance Optimization:
1. Eager Loading vs. Lazy Loading:
Entity Framework offers two loading strategies: eager loading and lazy loading. Eager loading retrieves all related entities upfront, while lazy loading loads related entities as needed. Choose the appropriate strategy to balance performance and resource consumption.
2. Using Compiled Queries:
Compiled queries can improve query execution performance by precompiling LINQ queries. By caching query execution plans, compiled queries eliminate the overhead of query compilation for frequently used queries.
3. Query Performance Optimization Techniques:
Optimizing query performance involves techniques like indexing, query caching, and query tuning. Use appropriate indexing strategies, cache query results when applicable, and analyze and optimize slow-performing queries.
Modifying Data with Entity Framework
1. Inserting Data:
To insert new data into the database using Entity Framework, create instances of entities and add them to the appropriate DbSet. Call SaveChanges to persist the changes to the database.
2. Updating Data:
Updating existing data involves fetching the entity, modifying its properties, and calling SaveChanges to save the changes to the database. Entity Framework tracks changes automatically, simplifying the update process.
3. Deleting Data:
To delete data, retrieve the entity to be deleted, remove it from the DbSet, and call SaveChanges to commit the deletion.
Handling Concurrency and Transactions
1. Handling Concurrent Updates:
Concurrency issues can arise when multiple users try to update the same data simultaneously. Entity Framework offers various concurrency control mechanisms, such as optimistic concurrency and row versioning, to handle these scenarios effectively.
2. Using Transactions:
Transactions ensure that a group of database operations is treated as a single unit of work. Use transactions to maintain data consistency and integrity when executing multiple operations that depend on each other.
Building Scalable Applications with Entity Framework:
1. Sharding and Partitioning Data:
Sharding and partitioning techniques allow you to distribute data across multiple databases or servers. This helps improve scalability by dividing the workload and reducing contention on a single database.
2. Using Caching Mechanisms:
Caching frequently accessed data can significantly improve the performance of data-driven applications. Implement caching mechanisms, such as in-memory caching or distributed caching, to store and retrieve data efficiently.
Conclusion
In this blog post, we explored the world of C# and Entity Framework, discovering how they work together to build powerful and efficient data-driven applications. We covered essential concepts, best practices, and code samples to help you get started on your journey to mastering this technology stack. With C# and Entity Framework, you have the tools to create robust, scalable, and high-performance applications that leverage the power of data. Start exploring and building your data-driven applications today!
Table of Contents
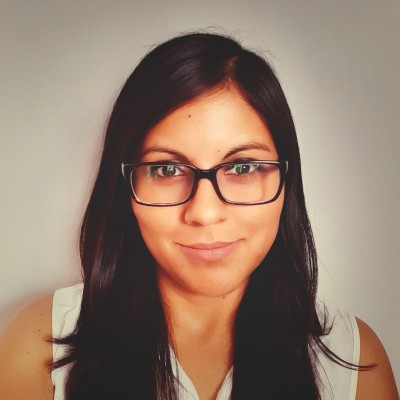
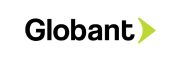