What is Entity Framework Core In-Memory Database in C#?
Entity Framework Core In-Memory Database is a lightweight and efficient database provider that allows developers to work with an in-memory database when building and testing their C# applications. It’s particularly useful for unit testing, integration testing, or scenarios where you want to avoid interactions with a physical database but still test your data access logic effectively.
Key features and characteristics of Entity Framework Core In-Memory Database include:
- In-Memory Storage: Instead of interacting with a real database server, Entity Framework Core In-Memory Database stores data in memory, which is much faster than traditional database operations.
- No Configuration Required: Setting up an in-memory database with Entity Framework Core is straightforward and doesn’t require complex configurations or connection strings. You can create an instance of the in-memory database context directly in your test code.
- Isolation: Each test typically runs in isolation, and the in-memory database ensures that data changes made during one test won’t affect other tests. This makes it easy to write unit tests that are predictable and reliable.
- Speed: In-memory databases are blazingly fast because they don’t involve disk I/O or network communication. This can significantly reduce the time it takes to run tests.
- No Persistence: Data stored in the in-memory database doesn’t persist beyond the lifetime of your application or tests. Once the application or test is complete, the data is discarded.
- Queryable: You can perform LINQ queries against the in-memory database, just like you would with a traditional database. This allows you to test your data access logic thoroughly.
- Useful for Mocking: In scenarios where you want to isolate your data access layer from external dependencies, such as a production database, you can use the in-memory database to mock database interactions effectively.
Here’s a basic example of how to use Entity Framework Core In-Memory Database in a C# unit test:
```csharp // Create an in-memory database context var options = new DbContextOptionsBuilder<MyDbContext>() .UseInMemoryDatabase(databaseName: "TestDatabase") .Options; using (var context = new MyDbContext(options)) { // Arrange: Add test data to the in-memory database context.MyEntities.Add(new MyEntity { Id = 1, Name = "TestEntity" }); context.SaveChanges(); // Act: Perform operations using the context // Assert: Verify the results var entity = context.MyEntities.FirstOrDefault(e => e.Id == 1); Assert.NotNull(entity); Assert.Equal("TestEntity", entity.Name); } ```
Entity Framework Core In-Memory Database is a valuable tool for C# developers, especially when it comes to writing efficient and isolated unit tests for their data access code without the overhead of a physical database.
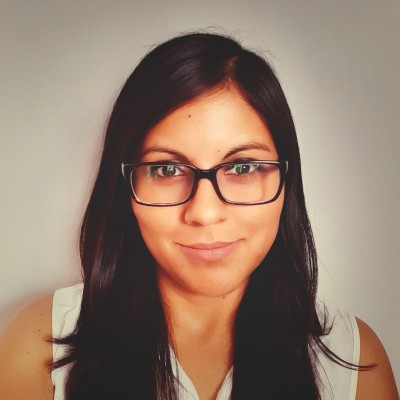
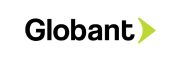