Leveraging the Power of Entity Framework for Efficient Database Operations in C#
Software development is increasingly data-driven, and managing databases efficiently is integral to the process. This is true for a range of applications, from web services to desktop applications. In this post, we will delve into working with databases in C#, a robust and versatile language well-suited to this task, which is one of many reasons to hire C# developers for your projects. For our practical approach, we will use Microsoft’s Entity Framework (EF), a powerful Object-Relational Mapping (ORM) tool that any skilled and dedicated C# developers should be comfortable with.
Understanding the Basics
What is Entity Framework?
Entity Framework is an open-source ORM framework for .NET applications. It enables developers to work with data in the form of domain-specific objects, without focusing on the underlying database tables and columns where the data is stored. With EF, you can write code to perform CRUD operations (Create, Read, Update, and Delete) without writing SQL queries.
Establishing a Database Connection
Before you start interacting with your database, you need to establish a connection to it. Entity Framework simplifies this process, abstracting much of the code necessary. To set up your connection, you just need to define the connection string in your application’s configuration file.
```csharp <connectionStrings> <add name="DefaultConnection" connectionString="Data Source=(LocalDb)\MSSQLLocalDB;Initial Catalog=YourDatabaseName;Integrated Security=True" providerName="System.Data.SqlClient" /> </connectionStrings>
Setting Up Entity Framework
Firstly, ensure you have installed the Entity Framework in your project. You can do this via the NuGet Package Manager Console by running:
``` Install-Package EntityFramework
Once installed, we can start defining our data models. These are classes that represent the tables in our database.
Suppose we are creating a blogging system, we might have a `Blog` and a `Post` class:
```csharp public class Blog { public int BlogId { get; set; } public string Name { get; set; } public virtual List<Post> Posts { get; set; } } public class Post { public int PostId { get; set; } public string Title { get; set; } public string Content { get; set; } public int BlogId { get; set; } public virtual Blog Blog { get; set; } }
In the above code, `BlogId` in the `Blog` class and `PostId` in the `Post` class are the primary keys. Entity Framework conventionally treats properties named `Id` or `<ClassName>Id` as primary keys.
We also need a context class that derives from the `DbContext` class to manage entity objects during run time, which includes populating objects with data from a database, change tracking, and persisting data to the database.
```csharp public class BloggingContext : DbContext { public DbSet<Blog> Blogs { get; set; } public DbSet<Post> Posts { get; set; } }
Performing CRUD Operations
With our models and context defined, we can perform CRUD operations.
Create Operation
To create and save a new blog:
```csharp using (var db = new BloggingContext()) { var blog = new Blog { Name = "My New Blog" }; db.Blogs.Add(blog); db.SaveChanges(); }
Read Operation
To fetch blogs:
```csharp using (var db = new BloggingContext()) { var blogs = db.Blogs.ToList(); }
Update Operation
To update a blog:
```csharp using (var db = new BloggingContext()) { var blog = db.Blogs.Find(1); // Find blog with Id 1 blog.Name = "Updated Blog Name"; db.SaveChanges(); }
Delete Operation
To delete a blog:
```csharp using (var db = new BloggingContext()) { var blog = db.Blogs.Find(1); // Find blog with Id 1 db.Blogs.Remove(blog); db.SaveChanges(); }
LINQ Queries
One of the benefits of Entity Framework is its compatibility with LINQ (Language Integrated Query). LINQ allows us to write queries using C# syntax. For instance, if we want to retrieve all blogs with a name that contains “tech”:
```csharp using (var db = new BloggingContext()) { var techBlogs = db.Blogs .Where(b => b.Name.Contains("tech")) .ToList(); }
Conclusion
Entity Framework in C# makes it possible to interact with databases in an intuitive, object-oriented manner, abstracting away much of the intricacy of SQL queries. We’ve discussed how to set up and use Entity Framework in your project, define your models and context, and perform CRUD operations and LINQ queries.
Working with databases can be a challenging aspect of software development, but tools like Entity Framework make this process much simpler and more manageable. If your team doesn’t have the necessary expertise, you might want to consider the option to hire C# developers. These professionals have a deep understanding of how to leverage powerful tools like Entity Framework for data-driven projects. So, when you’re faced with your next data-centric project in C#, consider the power of Entity Framework and the value that skilled C# developers can bring to your team.
Remember, learning is a continuous journey. So, keep exploring, keep learning, and have fun while at it! Happy coding!
Table of Contents
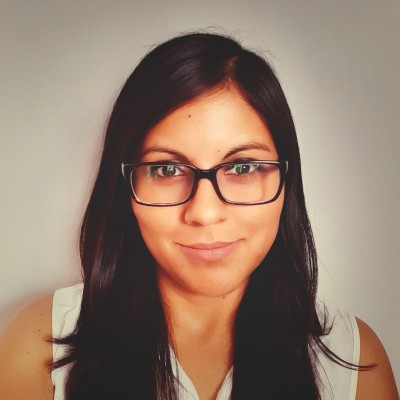
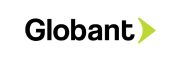