An Introductory Guide to the Fundamentals of C# Programming
If you’re eager to dive into the world of programming, seeking to add another language to your coding repertoire, or looking to hire C# developers for your team, then C# (pronounced “C-sharp”) could be an excellent choice. Developed by Microsoft as part of the .NET initiative, C# is a simple, modern, versatile, and type-safe object-oriented language. Whether you’re an aspiring programmer or a team leader intending to hire C# developers, understanding the language’s fundamentals is crucial.
In this blog post, we’ll cover the essentials of C# programming to get you started on your coding journey or to equip you with the knowledge needed to evaluate potential hires effectively.
Introduction to C#
Before we delve into the nuts and bolts, it’s important to understand a bit about C#. Primarily used for Windows desktop applications and game development, C# is also popular in web and mobile app development, thanks to cross-platform frameworks like Xamarin and .NET Core. C#’s syntax is similar to other C-style languages like C and Java, making it easier to learn if you already have experience with these languages.
Setting Up Your Environment
The first step in learning C#, or preparing to hire C# developers, is setting up the right environment. The most widely-used Integrated Development Environment (IDE) for C# is Microsoft’s Visual Studio. Other alternatives include Visual Studio Code, a lightweight, open-source IDE, or JetBrains Rider. Understanding these tools isn’t just crucial for aspiring programmers, it’s also important for those looking to hire C# developers as it allows you to gauge their familiarity with the tools of the trade. For this tutorial, we’ll use Visual Studio as our IDE of choice.
- Download and install Visual Studio from the official Microsoft website.
- During the installation process, select “.NET desktop development” to install the necessary workloads for C# programming.
- After installation, open Visual Studio and select “Create a new project.”
- Choose the “Console App (.NET Core)” template and name your project. This will create a new console application, a simple program that takes input and displays output in a command-line interface.
Basic Syntax and Concepts
Hello World!
Once your environment is set up, let’s start with the classic “Hello, World!” program to understand the basics of C# syntax.
```csharp using System; namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } } }
Let’s break down this code:
- `using System;` – This line imports the `System` namespace, which contains fundamental classes and base classes, like `Console`, which we will use later.
- `namespace HelloWorld` – Namespaces are used to organize code and avoid naming conflicts. Here, `HelloWorld` is the namespace we’ve defined.
- `class Program` – This defines a class, the blueprint from which objects are created. `Program` is the class name.
- `static void Main(string[] args)` – This is the Main method, the entry point of the program. When the program is executed, this is the first method that’s called.
- `Console.WriteLine(“Hello, World!”);` – This line prints “Hello, World!” to the console.
Variables and Data Types
C# is a statically-typed language, which means you have to declare the variable type when you create it. Here are some basic data types in C#:
– `int`: for integers (e.g., `int score = 100;`).
– `double`: for floating-point numbers (e.g., `double pi = 3.14;`).
– `char`: for single characters (e.g., `char letter = ‘A’;`).
– `bool`: for Boolean values (e.g., `bool isTrue = false;`).
– `string`: for text (e.g., `string name = “John”;`).
Control Flow
Control flow refers to the order in which the program’s code executes. The most common control flow elements are conditionals (if, else if, else) and loops (for, while).
An `if` statement allows the program to execute a block of code only if a specified condition is true.
```csharp int score = 85; if (score >= 90) { Console.WriteLine("Excellent"); } else if (score >= 70) { Console.WriteLine("Good"); } else { Console.WriteLine("Try harder"); }
A `for` loop repeats a block of code a specified number of times.
```csharp for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
Arrays
An array is a collection of variables of the same type. You must specify the size of an array at the time of creation.
```csharp int[] numbers = new int[5] {1, 2, 3, 4, 5}; foreach (int number in numbers) { Console.WriteLine(number); }
Methods
Methods are a way to group together code that performs a specific task. The Main method, which we’ve seen before, is an example of a method.
```csharp static void SayHello(string name) { Console.WriteLine("Hello, " + name); } static void Main(string[] args) { SayHello("John"); }
Conclusion
While we’ve just scratched the surface of C#, these fundamental concepts form the backbone of the language. Mastering these will provide a solid base to delve into more complex topics like object-oriented programming, exception handling, and file I/O operations in C#.
Learning to program in C# opens up a multitude of opportunities. Whether you aspire to create simple console applications, build robust web applications, venture into game development, or even aim to hire C# developers for your team, understanding the basics of C# is essential. With patience, practice, and consistency, you’ll find yourself proficient in C# in no time – either as a capable programmer or as an informed recruiter. So, why wait? Start your C# coding journey today!
Table of Contents
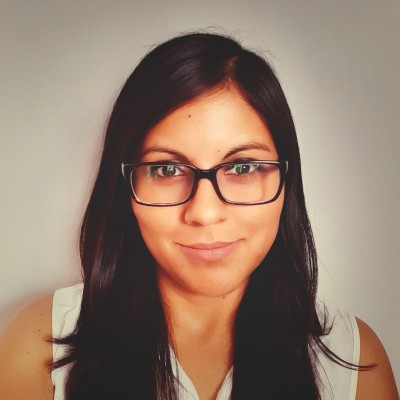
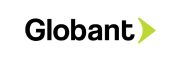