How to implement an interface in C#?
Implementing an interface in C# involves defining a class that provides concrete implementations for all the methods and properties declared within the interface. The process ensures that the class adheres to the contract specified by the interface, which includes implementing the required members with the correct method signatures. To implement an interface, follow these steps:
- Define the Interface: First, you need to have an interface with the method and property signatures you want the implementing class to adhere to. Interface declarations typically do not contain any method bodies; they only define the method signatures.
- Create a Class: Next, create a class that you want to implement the interface. The class should include the necessary logic for the methods and properties defined in the interface.
- Use the Implements Keyword: In the class declaration, use the `: interfaceName` syntax to indicate that the class implements the specified interface. For example, `class MyClass : IMyInterface` signifies that `MyClass` implements the `IMyInterface` interface.
- Implement Interface Members: Once the class is marked as implementing the interface, you must provide concrete implementations for all the methods and properties declared in the interface. These implementations should match the method signatures defined in the interface.
Here’s an example:
```csharp // Step 1: Define the Interface public interface IShape { double CalculateArea(); } // Step 2: Create a Class public class Circle : IShape { private double radius; // Step 3: Use the Implements Keyword public Circle(double r) { radius = r; } // Step 4: Implement Interface Members public double CalculateArea() { return Math.PI * Math.Pow(radius, 2); } } ```
In this example, the `Circle` class implements the `IShape` interface by providing an implementation for the `CalculateArea` method. This ensures that any instance of `Circle` adheres to the contract defined by the `IShape` interface.
By following these steps, you can implement interfaces in C# to define a common contract for classes, enabling polymorphism, code organization, and consistency in your codebase.
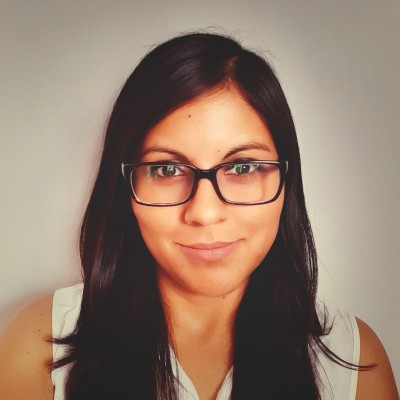
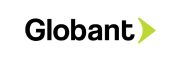