Introduction to LINQ in C#: Simplifying Data Queries
In the world of software development, data querying and manipulation are common tasks that developers face on a regular basis. Traditionally, these operations required writing complex and lengthy code, often involving multiple iterations and conditions. However, with the advent of Language Integrated Query (LINQ) in C#, developers can now simplify and streamline data queries, making their code more efficient and expressive.
What is LINQ?
LINQ, short for Language Integrated Query, is a powerful feature in C# that allows developers to query various data sources using a unified syntax. It enables developers to write queries in a declarative manner, similar to SQL, which greatly simplifies the process of extracting, transforming, and filtering data from different collections, databases, XML files, and more.
Key Benefits of LINQ
- Simplified Syntax: LINQ provides a concise and intuitive syntax for querying data, reducing the need for verbose and error-prone code. Developers can express complex queries using a few lines of code, resulting in cleaner and more maintainable codebases.
- Type Safety and IntelliSense: LINQ is integrated with the C# language, which means that queries are checked at compile-time for type safety. This ensures that the query will only operate on appropriate data types, minimizing runtime errors. Additionally, LINQ’s integration with IDEs like Visual Studio provides IntelliSense support, making it easier to explore available query operators and their parameters.
- Increased Productivity: By eliminating the need for manual iteration and conditional statements, LINQ simplifies the development process and reduces the amount of boilerplate code. This allows developers to focus more on the logic of their queries and spend less time on low-level data manipulation.
LINQ Query Expressions
LINQ introduces query expressions, which are syntactic sugar for writing LINQ queries in a familiar SQL-like syntax. Query expressions make it easier to express complex queries and combine various operators, such as filtering, sorting, grouping, and joining. Here’s an example of a LINQ query expression:
csharp var result = from product in products where product.Price > 50 orderby product.Name select product;
In this example, we select all products from a collection where the price is greater than 50, and then order the results by product name.
LINQ Extension Methods
Apart from query expressions, LINQ also provides a set of extension methods that can be directly applied to collections, arrays, and other data sources. These extension methods, defined in the System.Linq namespace, allow developers to chain together operations and perform complex queries in a fluent and readable manner. Here’s an example:
javascript var result = products .Where(product => product.Price > 50) .OrderBy(product => product.Name) .Select(product => product);
This code achieves the same result as the previous query expression example, but uses the extension methods syntax.
LINQ to SQL and LINQ to XML
LINQ is not limited to querying in-memory collections. It also provides specialized providers for querying databases using LINQ to SQL and XML data using LINQ to XML. These providers allow developers to interact with relational databases and XML files using LINQ syntax, seamlessly integrating data querying and manipulation into their C# code.
Conclusion
With its powerful and intuitive querying capabilities, LINQ revolutionizes the way developers interact with data in C#. By providing a unified syntax and a wide range of operators, LINQ simplifies data queries, making code more readable, maintainable, and efficient. Whether you’re working with in-memory collections, databases, or XML files, LINQ offers a versatile and flexible solution for data manipulation tasks. Embrace LINQ in your C# projects, and experience the benefits of streamlined data querying.
Table of Contents
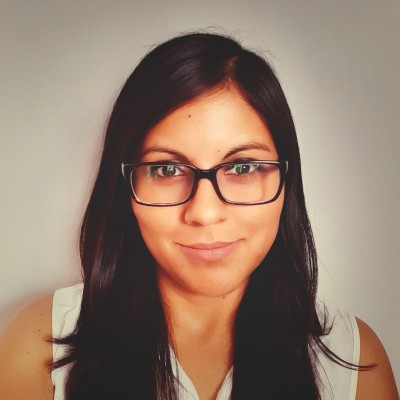
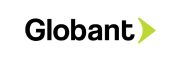