C# Performance Optimization: Tips and Tricks
In the world of software development, performance optimization is a crucial aspect to consider. Whether you’re building a small utility or a large-scale enterprise application, ensuring that your C# code runs efficiently can have a significant impact on user experience and overall system performance. In this blog post, we’ll explore some essential tips and tricks for optimizing C# performance, along with code samples and best practices to help you achieve maximum efficiency.
Measure and Identify Bottlenecks
Before diving into optimization techniques, it’s important to identify the areas of your code that require improvement. By measuring and profiling your application’s performance, you can pinpoint the exact bottlenecks and focus your optimization efforts where they matter the most.
1. Profiling Tools
Utilize profiling tools like Visual Studio Profiler, JetBrains dotTrace, or ANTS Performance Profiler to identify hotspots in your code. These tools provide valuable insights into CPU usage, memory allocation, and execution time, allowing you to identify performance bottlenecks.
2. Benchmarking
Benchmarking is another useful technique to measure and compare the performance of different code implementations. Libraries like BenchmarkDotNet make it easy to create benchmarks and analyze the results. By benchmarking critical sections of your code, you can identify areas that need optimization.
Optimize Memory Usage
Efficient memory management is crucial for C# performance optimization. Unoptimized memory usage can lead to unnecessary garbage collection, memory leaks, and decreased overall performance. Consider the following techniques to optimize memory usage in your C# application:
1. Object Pooling
Object pooling is a technique where you reuse objects instead of creating new instances. This reduces the overhead of object creation and garbage collection. The .NET framework provides the ObjectPool class to help you implement object pooling effectively.
csharp // Example of object pooling using ObjectPool<T> ObjectPool<MyClass> objectPool = new ObjectPool<MyClass>(() => new MyClass(), 10); // Retrieve an object from the pool MyClass obj = objectPool.Get(); // Use the object // Return the object to the pool objectPool.Return(obj);
2. Dispose and Finalizers
Properly managing resources using IDisposable and using blocks is essential for efficient memory management. Dispose unmanaged resources explicitly to avoid relying on finalizers, which may impact performance. Finalizers should be used sparingly and only when dealing with unmanaged resources that require cleanup.
csharp // Example of implementing IDisposable public class MyClass : IDisposable { private bool disposed = false; protected virtual void Dispose(bool disposing) { if (!disposed) { if (disposing) { // Dispose managed resources } // Dispose unmanaged resources disposed = true; } } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ~MyClass() { Dispose(false); } }
Optimize Looping and Iteration
Loops and iterations are common in most C# applications. Optimizing them can yield significant performance improvements. Consider the following tips to enhance looping and iteration efficiency:
1. Minimize Loop Overhead
Reduce unnecessary computations and function calls within loops. Move invariant calculations outside the loop to avoid repetitive calculations.
csharp // Example of minimizing loop overhead int length = array.Length; for (int i = 0; i < length; i++) { // Loop body }
2. Use foreach for Enumerations
When iterating over collections, use the foreach loop instead of a traditional for loop. The foreach loop is optimized for iterating over collections, leading to cleaner and more efficient code.
csharp // Example of using foreach loop foreach (var item in collection) { // Loop body }
Optimize String Manipulation
String manipulation can be a performance bottleneck, especially when dealing with large amounts of data. To optimize string manipulation, consider the following techniques:
1. Use StringBuilder
When concatenating multiple strings, use the StringBuilder class instead of the + operator. The StringBuilder minimizes memory allocations and performs better for extensive string manipulations.
csharp // Example of using StringBuilder StringBuilder sb = new StringBuilder(); sb.Append("Hello"); sb.Append(" "); sb.Append("World"); string result = sb.ToString();
2. Use String Interpolation
String interpolation provides a concise and efficient way to concatenate variables within a string. It performs better than traditional concatenation using the + operator.
csharp // Example of string interpolation string name = "John"; string message = $"Hello, {name}!";
Optimize Database Operations
Database operations are often a performance bottleneck in C# applications. Consider the following tips to optimize database interactions:
1. Use Parameterized Queries
Avoid dynamically constructing queries by concatenating strings. Instead, use parameterized queries or prepared statements to reduce the risk of SQL injection and improve query execution performance.
csharp // Example of parameterized query using SqlCommand string query = "SELECT * FROM Users WHERE Id = @userId"; using (SqlCommand command = new SqlCommand(query, connection)) { command.Parameters.AddWithValue("@userId", userId); // Execute the command }
2. Efficient Data Retrieval
Retrieve only the necessary data from the database. Use appropriate SQL queries with filtering, pagination, and indexing to minimize the amount of data transferred between the database and the application.
Conclusion
C# performance optimization is essential for ensuring the smooth execution of your applications. By following the tips and tricks mentioned in this blog post, you can significantly enhance your code’s efficiency. Remember to measure and profile your code, optimize memory usage, streamline loops and iterations, optimize string manipulation, and employ efficient database operations. By employing these techniques, you’ll be able to build high-performing C# applications that deliver a seamless user experience.
Table of Contents
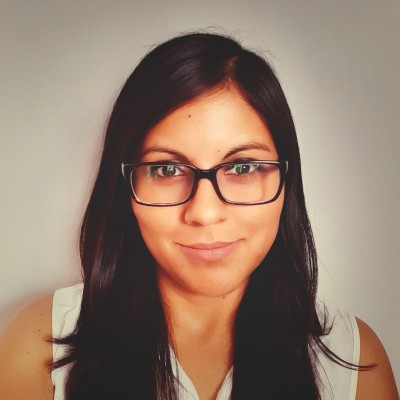
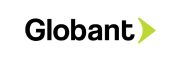