A Step-by-Step C# Programming Guide for Beginners to Experts
C# (pronounced C-sharp) is a popular, versatile, and general-purpose programming language developed by Microsoft as part of its .NET platform. While being easy to learn, especially for those familiar with Java or C++, it’s also robust enough to build all kinds of applications, from web applications, desktop software, game development, and even mobile applications with Xamarin.
This guide aims to equip beginners with the foundational knowledge of C# and provide experienced programmers with tips and tricks to master the language. Additionally, for organizations seeking expertise in this domain, it also serves as a helpful primer on what to look for when they hire C# developers.
Getting Started with C#
Getting started with C# involves several steps:
- Setup Development Environment: The first step in starting with C# is setting up a development environment. There are several Integrated Development Environments (IDEs) available, but the most popular is Visual Studio. You can also use Visual Studio Code, which is a lighter version.
To install Visual Studio, you can download it from the official Microsoft website. Follow the prompts for installation and make sure to select the “.NET desktop development” workload. This will give you all the tools necessary for building desktop applications with C#.
- Understanding C# Syntax: Once you have the development environment set up, the next step is understanding the basic syntax. Here’s a simple C# program:
```csharp // This is a basic C# Program using System; class Program { static void Main() { Console.WriteLine("Hello, World!"); } }
This program prints “Hello, World!” to the console. Here’s what’s happening:
– `using System;` This line is using the System namespace, which contains fundamental classes and base classes that define commonly-used value and reference data types, events and event handlers, interfaces, attributes, and processing exceptions.
– `class Program` This line declares a class, a construct that enables you to create your own custom types by grouping together variables of other types, methods, and events. In C#, every line of code that executes must be inside a class. In our example, the class name is `Program`.
– `static void Main()` This line declares a method. The Main method is the entry point for every C# application and it’s called when the program starts. The static keyword tells us that this method belongs to the class, not an instance of the class.
– `Console.WriteLine(“Hello, World!”);` This line is a statement. It invokes the `WriteLine` method of the `Console` class, which prints the string passed as an argument to the console.
- Running C# Code: You can run your code within Visual Studio by pressing the “Start Debugging” button or hitting the F5 key. Your output will be displayed in a console window.
- Exploring More Concepts: Once you’re comfortable with the basic syntax and running programs, you can start exploring more concepts such as variables, data types, loops, conditionals, arrays, classes and methods, inheritance, interfaces, etc.
The best way to learn is by doing. Keep experimenting with different code snippets and keep building increasingly complex applications as you learn new concepts. C# is a deep language with a lot of capabilities, making it highly sought after. Therefore, if you’re an organization looking to leverage these capabilities, it’s crucial to hire C# developers who have honed their skills through such hands-on experiences.
Variables and Data Types
C# is a strongly typed language, meaning you have to declare the type of data you want to store in a variable. C# supports several data types, including int (for integers), double (for floating-point numbers), char (for characters), and string (for text). Here’s an example:
int myNumber = 5; double myDouble = 5.5; char myCharacter = 'A'; string myString = "Hello World";
Control Structures
Control structures allow you to control the flow of execution in a program. The basic control structures in C# are the if-else statement, switch-case statement, while loop, for loop, and the do-while loop. Mastering these structures can help you write complex programs with ease.
Functions and Methods
A method in C# is a block of code that contains a series of statements. A program causes the statements to be executed by calling the method and specifying any required method arguments. Learning how to create methods in C# is crucial as they promote reusability and modularity of code.
void MyMethod() { Console.WriteLine("This is a custom method"); }
Classes and Objects
As an object-oriented language, C# is built around the concept of classes and objects. A class is a blueprint for creating objects. An object is an instance of a class. This allows programmers to create complex applications with ease.
public class MyClass { public string MyProperty { get; set; } } MyClass myObject = new MyClass();
Exception Handling
In C#, you can handle exceptions (errors) using the try-catch-finally blocks. It’s a critical aspect of the language to ensure your program runs smoothly without crashing.
Advanced Topics
After getting a solid foundation in the basic elements of C#, you can then proceed to learn the advanced topics like:
- Inheritance and Polymorphism: This allows you to create a hierarchy of classes and leverage code reusability.
- Interfaces and Abstract Classes: These are important for creating complex and extendable systems.
- Generics: Generics are used to make reusable code classes that work with different data types.
- LINQ (Language Integrated Query): LINQ is a powerful feature in C# that allows you to work with data in a more intuitive and declarative way.
- Asynchronous Programming: This is a modern way of handling long-running tasks in the background, a key requirement in today’s responsive and interactive applications.
- Memory Management and Garbage Collection: Understanding this helps in efficient memory use and enhancing application performance.
- Unit Testing and TDD: Testing is a key aspect of modern software development practices.
Practical Learning
Apart from learning the theoretical aspects of the language, you need to practice writing C# code to fully grasp the concepts. This involves taking on mini-projects, solving problems on coding platforms, and contributing to open-source projects, as you always strive to write clean, efficient code. This practical experience not only enhances your skills but also makes you a valuable candidate for businesses looking to hire C# developers.
Conclusion
“Mastering C# involves a combination of understanding its syntax and key concepts, as well as lots of practice writing code. This powerful, versatile language forms the backbone of many applications in the real world, making it a valuable language to master for any developer. By following the guide above, beginners and experienced programmers alike can gain a solid understanding of C#, setting them on the path to mastery.
For companies seeking to leverage this power and versatility, the ability to hire C# developers who have embarked on this journey to mastery can make a significant difference in their tech-driven projects.
Remember, the journey to mastering C# is a marathon, not a sprint. The more you use C#, the better you’ll understand and be able to effectively use this language. This continuous learning and application not only benefit individual developers but are also a compelling reason for organizations to hire C# developers. Happy coding!”
Table of Contents
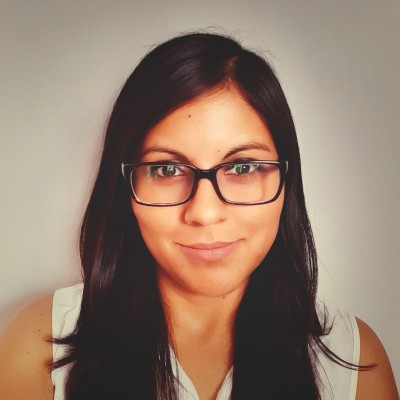
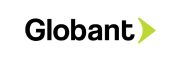