Advanced C# Programming Techniques
As a seasoned C# programmer, you’ve likely mastered the basics and are eager to explore more advanced techniques to further improve your skills. In this blog post, we will delve into advanced C# programming techniques that will elevate your development abilities and empower you to write more efficient, maintainable, and scalable code. We’ll cover a range of topics, including advanced language features, design patterns, asynchronous programming, and performance optimization. So let’s dive in!
Advanced Language Features
1. Delegates and Events:
Delegates and events are powerful features in C# that enable you to implement event-driven architectures and decoupled communication between components. Here’s an example of using delegates and events:
csharp public delegate void MyEventHandler(object sender, EventArgs e); public class Publisher { public event MyEventHandler MyEvent; public void PublishEvent() { // Trigger the event MyEvent?.Invoke(this, EventArgs.Empty); } } public class Subscriber { public void HandleEvent(object sender, EventArgs e) { // Handle the event } } // Usage: var publisher = new Publisher(); var subscriber = new Subscriber(); publisher.MyEvent += subscriber.HandleEvent; publisher.PublishEvent();
2. Generics:
Generics allow you to create reusable code by writing classes and methods that work with various types. This enhances code flexibility and eliminates the need for duplicating logic. Here’s an example of using generics:
csharp public class Stack<T> { private List<T> items = new List<T>(); public void Push(T item) { items.Add(item); } public T Pop() { var lastIndex = items.Count - 1; var item = items[lastIndex]; items.RemoveAt(lastIndex); return item; } } // Usage: var stack = new Stack<int>(); stack.Push(42); int value = stack.Pop();
3. Extension Methods:
Extension methods allow you to add functionality to existing types without modifying their original implementation. They can be useful for adding custom behavior to third-party libraries or enhancing the capabilities of built-in types. Here’s an example of using extension methods:
csharp public static class StringExtensions { public static string Reverse(this string str) { var charArray = str.ToCharArray(); Array.Reverse(charArray); return new string(charArray); } } // Usage: string reversed = "Hello, World!".Reverse();
Design Patterns in C#
1. Singleton Pattern:
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. It is often used for resource sharing and managing global state. Here’s an example of implementing the Singleton pattern:
csharp public sealed class Singleton { private static Singleton instance; private static readonly object lockObject = new object(); private Singleton() { } public static Singleton Instance { get { if (instance == null) { lock (lockObject) { if (instance == null) { instance = new Singleton(); } } } return instance; } } } // Usage: Singleton singleton = Singleton.Instance;
2. Factory Pattern:
The Factory pattern provides an interface for creating objects, allowing subclasses to decide which class to instantiate. It promotes loose coupling and enhances code maintainability. Here’s an example of using the Factory pattern:
csharp public abstract class Product { public abstract void Use(); } public class ConcreteProductA : Product { public override void Use() { // Use ConcreteProductA } } public class ConcreteProductB : Product { public override void Use() { // Use ConcreteProductB } } public abstract class Creator { public abstract Product CreateProduct(); } public class ConcreteCreatorA : Creator { public override Product CreateProduct() { return new ConcreteProductA(); } } public class ConcreteCreatorB : Creator { public override Product CreateProduct() { return new ConcreteProductB(); } } // Usage: Creator creator = new ConcreteCreatorA(); Product product = creator.CreateProduct(); product.Use();
3. Observer Pattern:
The Observer pattern defines a one-to-many dependency between objects, where changes in one object trigger updates in dependent objects. It allows for loosely coupled communication and simplifies the implementation of event-driven systems. Here’s an example of implementing the Observer pattern:
csharp public interface IObserver { void Update(); } public class ConcreteObserver : IObserver { public void Update() { // Handle update } } public interface IObservable { void AddObserver(IObserver observer); void RemoveObserver(IObserver observer); void NotifyObservers(); } public class ConcreteObservable : IObservable { private List<IObserver> observers = new List<IObserver>(); public void AddObserver(IObserver observer) { observers.Add(observer); } public void RemoveObserver(IObserver observer) { observers.Remove(observer); } public void NotifyObservers() { foreach (var observer in observers) { observer.Update(); } } } // Usage: var observable = new ConcreteObservable(); var observer = new ConcreteObserver(); observable.AddObserver(observer); observable.NotifyObservers();
Asynchronous Programming
1. Asynchronous Methods and Await:
Asynchronous programming enables you to write non-blocking code, improving the responsiveness and scalability of your applications. The async and await keywords simplify the process of working with asynchronous methods. Here’s an example:
csharp public async Task<int> LongRunningOperationAsync() { await Task.Delay(1000); // Simulating a long-running operation return 42; } // Usage: int result = await LongRunningOperationAsync();
2. Parallel Programming:
Parallel programming allows you to divide a problem into smaller tasks and execute them concurrently, leveraging multiple processor cores for improved performance. The Parallel class provides useful methods for parallelizing operations. Here’s an example:
csharp Parallel.For(0, 10, i => { // Perform parallel task }); // or Parallel.ForEach(collection, item => { // Perform parallel task });
Performance Optimization:
1. Profiling and Benchmarking:
Profiling and benchmarking tools help identify performance bottlenecks and optimize critical sections of your code. Tools like Visual Studio Profiler and benchmarking libraries such as BenchmarkDotNet are invaluable for performance analysis and optimization.
2. Memory Management Techniques:
Understanding memory management is crucial for writing high-performance C# code. Techniques such as object pooling, reducing memory allocations, and minimizing garbage collection pressure can significantly enhance performance and reduce memory overhead.
3. Multi-threading and Concurrency:
Leveraging multi-threading and concurrency techniques can improve performance by allowing your application to perform multiple tasks simultaneously. However, it requires careful synchronization and coordination to avoid issues like race conditions and deadlocks.
Conclusion
By exploring advanced C# programming techniques, including delegates and events, generics, extension methods, design patterns, asynchronous programming, and performance optimization, you’ve expanded your programming arsenal. These techniques will empower you to write more efficient, maintainable, and scalable code. Continuously honing your skills and keeping up with advancements in the C# ecosystem will unlock endless possibilities for creating robust and high-performance applications. Happy coding!
Table of Contents
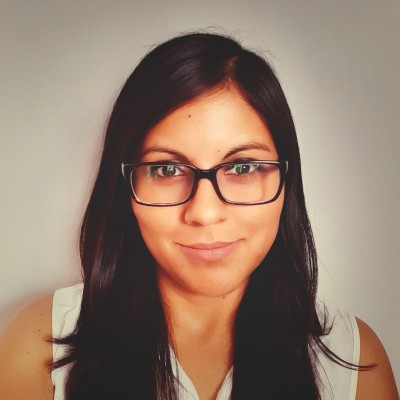
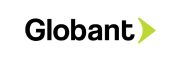