How to use properties in C#?
Properties in C# are a way to encapsulate the state of an object and provide controlled access to its internal data. They allow you to expose fields (variables) of a class while enforcing rules and logic to read and write that data. Properties have a get accessor (used for reading) and optionally a set accessor (used for writing) and are defined using the `get` and `set` keywords. Here’s how you can use properties in C#:
- Define a Property:
To define a property, declare it within a class using the `get` and `set` accessors. The `get` accessor defines how to retrieve the property’s value, and the `set` accessor defines how to assign a new value to the property.
- Use Auto-Implemented Properties:
C# provides a convenient way to create properties known as auto-implemented properties. With auto-implemented properties, the compiler automatically generates the backing field for you. Here’s an example:
```csharp public int Age { get; set; } ```
In this example, `Age` is an auto-implemented property with a default getter and setter.
- Custom Logic in Properties:
You can also add custom logic within property accessors. For instance, you can perform validation checks, compute values on-the-fly, or log changes. Here’s an example with custom logic:
```csharp private string _name; public string Name { get { return _name; } set { if (!string.IsNullOrEmpty(value)) { _name = value; } } } ```
In this case, the `Name` property ensures that the value being set is not null or empty before assigning it to the `_name` field.
- Access Properties:
To access properties of an object, you use the dot notation. For example:
```csharp Person person = new Person(); person.Name = "John"; int age = person.Age; ```
In this code, we set the `Name` property and retrieve the `Age` property of the `Person` object.
Properties provide a structured and controlled way to interact with an object’s data. They allow you to hide the internal implementation details of a class while enforcing rules and logic, promoting data encapsulation and maintainability in your C# code.
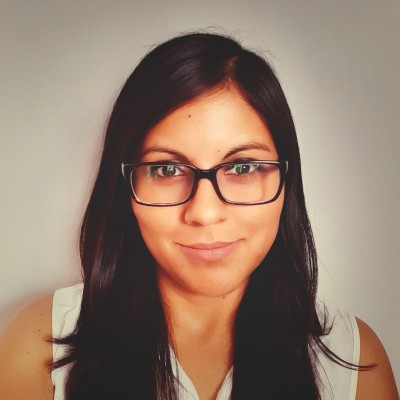
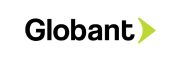