C# Q & A
How to create and use query types in Entity Framework Core?
Creating and using query types in Entity Framework Core involves several steps to effectively map and work with data that doesn’t correspond to traditional entity types. Query types are particularly valuable when dealing with views, stored procedures, or custom SQL queries in your database. Here’s a step-by-step guide:
- Define a Query Type Class: Start by creating a C# class that represents your query type. This class should contain properties that match the columns or computed values from the source you intend to query. To indicate that this class is a query type, decorate it with `[Keyless]` attribute. For example:
```csharp [Keyless] public class SalesSummary { public int ProductId { get; set; } public string ProductName { get; set; } public decimal TotalSales { get; set; } } ```
- Configure the Query Type: In your `DbContext` class, override the `OnModelCreating` method and use the `Query` method to configure your query type. You specify the SQL query, view, or stored procedure you want to map to this query type using the `ToView`, `ToSqlQuery`, or `ToStoredProcedure` method. For example:
```csharp protected override void OnModelCreating(ModelBuilder modelBuilder) { modelBuilder .Query<SalesSummary>() .ToView("vw_SalesSummary"); // Replace with your view name } ```
- Query the Data: Now that you’ve defined and configured your query type, you can use it in your LINQ queries just like regular entity types. Entity Framework Core will automatically project the results into instances of your query type. For example:
```csharp var sales = dbContext.SalesSummaries .Where(s => s.ProductId == productId) .ToList(); ```
- Benefits: Query types are read-only, which means you can’t use them to perform insert, update, or delete operations. However, they excel at efficiently querying and projecting data from complex database structures, making them suitable for reporting, analytics, or scenarios where performance is critical.
Creating and using query types in Entity Framework Core involves defining a C# class, configuring it in your `DbContext`, and then seamlessly querying your data using LINQ. Query types are a valuable feature for handling data that doesn’t fit the traditional entity model, allowing you to work with database views and custom queries effectively.
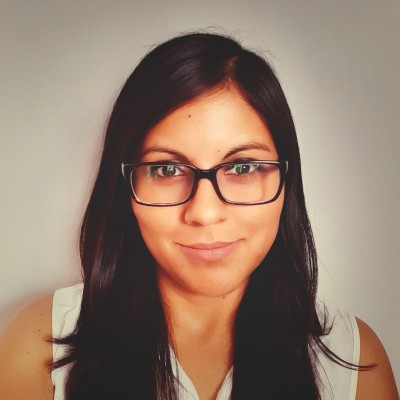
Previously at
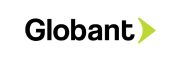
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.