What is the difference between throw and throw ex in C#?
The difference between `throw` and `throw ex` in C# lies in how they handle exceptions and preserve the call stack information:
- `throw`:
– When you use `throw` without the `ex` keyword (e.g., `throw new Exception(“Error message”)`), you create a new exception object and throw it.
– This preserves the original call stack information, including the source of the exception and where it was thrown in the code.
– The call stack information is valuable for debugging because it allows you to trace the origin of the exception back to the point where it was initially thrown.
– Using `throw` is generally recommended in most cases, as it provides better information for diagnosing and debugging issues.
- `throw ex`:
– When you use `throw ex` (e.g., `throw new Exception(“Error message”)`) with the `ex` keyword, you effectively re-throw the caught exception.
– While this may seem similar to using `throw`, it has a significant drawback: it resets the call stack information.
– The call stack information is overwritten, and the exception appears to have originated from the point where it was re-thrown rather than its original source.
– As a result, debugging becomes more challenging because you lose the context of where the exception initially occurred.
Here’s a code example to illustrate the difference:
```csharp try { // Code that may throw an exception } catch (Exception ex) { // Using 'throw' to preserve call stack information Console.WriteLine("Original exception: " + ex.StackTrace); throw; // Preserve the original call stack } ```
In this example, using `throw` without the `ex` keyword ensures that the original call stack information is preserved, allowing for more effective debugging. In contrast, using `throw ex` would reset the call stack, making it harder to trace the root cause of the exception.
In most scenarios, it is recommended to use `throw` without `ex` to maintain the integrity of the call stack and facilitate easier debugging when handling exceptions in C#.
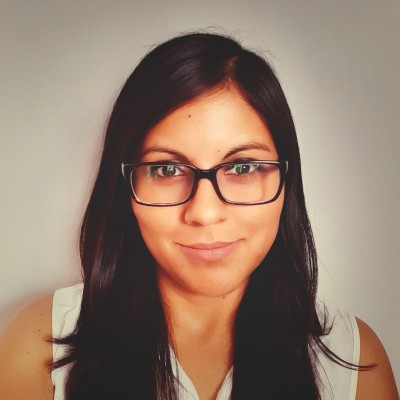
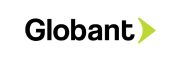