Unit Testing in C#: Ensuring Code Quality
In the realm of software development, ensuring code quality is paramount to building robust and reliable applications. Unit testing, a fundamental practice in test-driven development (TDD), plays a crucial role in achieving this goal. By systematically testing individual units of code, developers can identify and rectify bugs and design flaws early in the development cycle. This blog post will delve into the world of unit testing in C# and explore its benefits, best practices, and code samples.
What is Unit Testing?
Unit testing is a software testing technique that focuses on testing individual units or components of a program to verify their correctness. In the context of C# development, a unit typically refers to a method or function. Unit tests are written to verify the behavior of these individual units and ensure they work as expected. These tests are automated, repeatable, and can be run frequently to catch bugs early in the development process.
Benefits of Unit Testing
1. Bug Detection and Prevention
One of the primary benefits of unit testing is early bug detection and prevention. By writing tests for each unit of code, developers can catch issues before they propagate to other parts of the system. This leads to better overall code quality and reduces the likelihood of encountering critical bugs in production.
2. Code Refactoring and Maintainability
Unit tests act as a safety net when refactoring code. By having a comprehensive test suite, developers can confidently modify code without the fear of breaking existing functionality. This facilitates code refactoring, which leads to cleaner, more maintainable code over time.
3. Improved Collaboration and Code Documentation
Unit tests serve as living documentation for the codebase. When new developers join a project, they can easily understand how individual units of code are supposed to behave by examining the associated unit tests. Additionally, writing tests forces developers to think about the expected behavior of their code, leading to better-designed interfaces and clearer specifications.
Unit Testing Frameworks in C#
C# provides several popular unit testing frameworks that simplify the process of writing and executing unit tests. Some of the prominent frameworks include MSTest, NUnit, and xUnit.net. These frameworks offer various features such as test discovery, assertions, and test runners that make unit testing in C# more efficient and manageable.
1. MSTest
MSTest is the built-in unit testing framework in Visual Studio. It offers a simple and intuitive way to write tests and provides features like test initialization and cleanup. MSTest supports parallel test execution and integrates seamlessly with the Visual Studio IDE.
Sample code using MSTest:
csharp [TestClass] public class MathTests { [TestMethod] public void TestAddition() { // Arrange var math = new Math(); // Act var result = math.Add(2, 3); // Assert Assert.AreEqual(5, result); } }
2. NUnit
NUnit is a widely used open-source unit testing framework for .NET. It offers a rich set of assertions, test fixtures, and attributes for organizing tests. NUnit supports data-driven testing, parallel test execution, and extensibility through custom attributes and extensions.
Sample code using NUnit:
csharp [TestFixture] public class MathTests { [Test] public void TestAddition() { // Arrange var math = new Math(); // Act var result = math.Add(2, 3); // Assert Assert.AreEqual(5, result); } }
3. xUnit.net
xUnit.net is another popular open-source unit testing framework that follows the principles of simplicity, extensibility, and test parallelization. It provides an extensive set of attributes, assertions, and test runners. xUnit.net encourages a data-driven approach to testing and promotes clean test code by removing unnecessary abstractions.
Sample code using xUnit.net:
csharp public class MathTests { [Fact] public void TestAddition() { // Arrange var math = new Math(); // Act var result = math.Add(2, 3); // Assert Assert.Equal(5, result); } }
Writing Unit Tests in C#
1. Test Class and Test Methods
To write unit tests in C#, you create a separate test class that contains individual test methods. Each test method should focus on testing a specific unit of code and be independent of other tests. The [TestMethod] attribute in MSTest, [Test] attribute in NUnit, or [Fact] attribute in xUnit.net is used to mark a method as a test method.
2. Assertions and Expected Results
Assertions are used to verify the expected behavior of the unit being tested. They help ensure that the code under test produces the desired output. Common assertions include checking for equality, inequality, nullity, exceptions, and more. The testing frameworks provide various assertion methods to handle different scenarios.
3. Test Fixtures and Test Initialization
Test fixtures provide a way to share common setup and cleanup code across multiple tests. They are particularly useful when tests require the same set of objects or resources. The [TestFixture] attribute in NUnit and [TestClass] attribute in MSTest define a test fixture. Test initialization code can be placed in a setup method, executed before each test, and cleanup code in a teardown method, executed after each test.
Test-Driven Development (TDD) and Unit Testing
Test-Driven Development (TDD) is an iterative development approach that emphasizes writing tests before writing the production code. TDD works hand in hand with unit testing, as it helps drive the design and implementation of the code. TDD follows the red-green-refactor cycle, where tests are initially written and executed, then the code is written to make the tests pass, and finally, the code is refactored to improve its design and maintainability.
1. Red-Green-Refactor Cycle
In the red phase, tests are written for the desired behavior, but they fail because the code is not implemented yet. In the green phase, the minimum code necessary is written to make the tests pass. Once the tests pass, the code is refactored in the refactor phase to improve its structure without changing its behavior. This cycle repeats until the desired functionality is achieved.
2. Mocking and Dependency Injection
To isolate units of code during testing, it is common to use mocking frameworks and dependency injection. Mocking frameworks help create mock objects that simulate the behavior of external dependencies, allowing you to test individual units in isolation. Dependency injection enables the substitution of real dependencies with mocks or stubs, making unit testing more effective.
3. Test Coverage and Continuous Integration
Test coverage measures the extent to which the code is covered by tests. It is essential to have good test coverage to ensure that critical code paths are tested. Continuous Integration (CI) practices involve running unit tests automatically and regularly as part of the development process. CI tools like Jenkins, Travis CI, or Azure Pipelines can be configured to execute unit tests on every code commit, providing rapid feedback on code changes.
Best Practices for Effective Unit Testing
1. Keep Tests Independent and Isolated
Unit tests should be independent and not rely on the state or order of other tests. Each test should set up its required state and clean up after itself. This ensures that tests can be run individually or in any order without causing interference or unexpected failures.
2. Test Naming Conventions and Readability
Well-named tests help developers understand their purpose and intent. Use descriptive names that clearly convey what aspect of the code is being tested and what the expected outcome is. Additionally, write tests that are easy to read and understand, making it simpler for developers to maintain and debug them in the future.
3. Maintain a Good Test Suite Structure
Organize your tests into logical groupings using test fixtures, namespaces, or test categories. This helps in locating and running specific sets of tests when needed. A well-structured test suite enables developers to navigate and manage tests efficiently.
4. Use Test Doubles for External Dependencies
When unit testing code that depends on external services or resources, such as databases or web APIs, it is crucial to use test doubles like mocks or stubs. Test doubles help simulate the behavior of the dependencies, allowing tests to focus on the unit under test and avoiding external dependencies during testing.
5. Continuous Integration and Automated Testing
Integrate unit testing into your development workflow by incorporating it into your CI process. Automated testing ensures that tests are executed consistently and frequently, providing early feedback on code changes and reducing the chances of introducing bugs.
Common Unit Testing Pitfalls and How to Avoid Them
1. Writing Fragile Tests
Fragile tests are tests that break frequently due to changes in unrelated code or implementation details. To avoid fragile tests, focus on testing behavior rather than implementation details. Use appropriate abstractions and avoid tightly coupling tests to specific code internals.
2. Neglecting Edge Cases
Unit tests should cover a wide range of scenarios, including edge cases and boundary conditions. Neglecting these cases can leave critical parts of the code untested, leading to potential issues in production. Ensure that your test suite includes tests for all possible inputs and corner cases.
3. Over-Testing or Under-Testing
Finding the right balance is crucial when it comes to unit testing. Over-testing can lead to excessive maintenance overhead and slow down development, while under-testing may result in incomplete coverage and increased risks. Use code coverage tools to monitor test coverage and ensure that critical code paths are adequately tested.
Conclusion
Unit testing is an essential practice for ensuring code quality and building reliable software applications. By systematically testing individual units of code, developers can catch bugs early, facilitate code refactoring, and improve collaboration within development teams. By following best practices and leveraging the rich unit testing frameworks available in C#, developers can elevate the quality of their code and enhance the software development process as a whole. Start incorporating unit testing in your C# projects today to reap the benefits of improved code quality and robustness.
Table of Contents
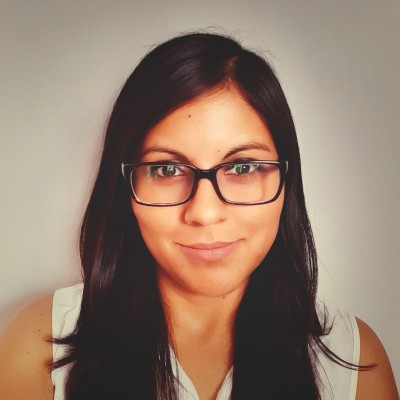
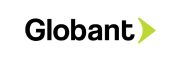