How to use dependency injection in ASP.NET Core Razor Pages?
Using Dependency Injection (DI) in ASP.NET Core Razor Pages is a fundamental practice that allows you to inject and utilize services or dependencies within your Razor Page classes. DI helps promote modularity, testability, and maintainability in your web applications. Here’s a step-by-step guide on how to use DI in ASP.NET Core Razor Pages:
- Service Registration:
Start by registering your services or dependencies with the built-in IoC (Inversion of Control) container in the `Startup.cs` file within the `ConfigureServices` method. This registration tells ASP.NET Core how to create and manage instances of your services. For example:
```csharp services.AddScoped<IMyService, MyService>(); ```
In this code, we’re registering an interface `IMyService` and associating it with the concrete implementation `MyService`. You can use `AddTransient`, `AddScoped`, or `AddSingleton` based on your desired service lifetime.
- Constructor Injection:
In your Razor Page class, request the required services through constructor injection. The IoC container will automatically provide these dependencies when creating an instance of your Razor Page. For example:
```csharp public class MyPageModel : PageModel { private readonly IMyService _myService; public MyPageModel(IMyService myService) { _myService = myService; } // Use _myService within your Razor Page logic } ```
Here, `IMyService` is injected into `MyPageModel`’s constructor, making it accessible for use throughout the page.
- Service Usage:
You can now use the injected service (`_myService` in this case) within your Razor Page’s methods and properties. This enables you to access data, perform business logic, or interact with other dependencies easily.
- Benefits:
Utilizing DI in Razor Pages offers numerous advantages. It encourages separation of concerns, making your code more organized and maintainable. It also simplifies unit testing, as you can easily substitute real services with mock implementations during testing. Additionally, DI allows you to change or extend functionality by altering service registrations, without needing to modify existing code.
Using Dependency Injection in ASP.NET Core Razor Pages is a crucial practice for creating scalable and maintainable web applications. It streamlines the management of dependencies, promotes clean architecture, and facilitates effective testing of your Razor Pages.
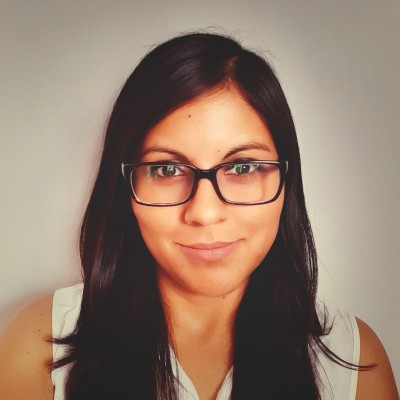
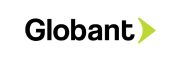