What is asynchronous programming in C#?
Asynchronous programming in C# is a technique that allows you to write non-blocking code, which is particularly valuable when dealing with operations that may take time to complete, such as I/O-bound tasks or network requests. Instead of waiting for a task to finish before moving on, asynchronous programming enables your application to continue executing other tasks while the initial operation is in progress. This improves the overall responsiveness and efficiency of your program.
Key components of asynchronous programming in C# include:
- Async and Await Keywords: C# introduces the `async` and `await` keywords, which are used to define and await asynchronous operations. The `async` keyword indicates that a method contains asynchronous code, and the `await` keyword is used to pause the execution of the method until the awaited task is completed.
- Tasks: Asynchronous operations are often represented as `Task` objects. A `Task` represents a unit of work that can be executed asynchronously. You can create and await tasks to perform operations without blocking the main thread.
- Concurrency: Asynchronous programming enables concurrency by allowing multiple tasks to run concurrently without blocking the execution thread. This is particularly beneficial in user interfaces to maintain responsiveness.
- I/O Operations: Asynchronous programming is commonly used for I/O-bound tasks, such as reading and writing files, making web requests, or querying databases. These operations typically involve waiting for external resources, making them ideal candidates for asynchrony.
- Exception Handling: Proper exception handling is essential in asynchronous code. Unhandled exceptions can be challenging to diagnose, so developers must ensure that exceptions are captured and handled appropriately in asynchronous methods.
Here’s a simplified example of asynchronous programming in C#:
```csharp using System; using System.Net.Http; using System.Threading.Tasks; class Program { static async Task Main() { Console.WriteLine("Start of Main"); // Asynchronously fetch a web page string htmlContent = await DownloadWebPageAsync("https://example.com"); Console.WriteLine("Length of the web page: " + htmlContent.Length); Console.WriteLine("End of Main"); } static async Task<string> DownloadWebPageAsync(string url) { using (var httpClient = new HttpClient()) { return await httpClient.GetStringAsync(url); } } } ```
In this example, the `await` keyword is used to asynchronously download a web page without blocking the main thread. Asynchronous programming is a crucial part of modern C# development, ensuring that applications remain responsive and efficient when dealing with time-consuming operations.
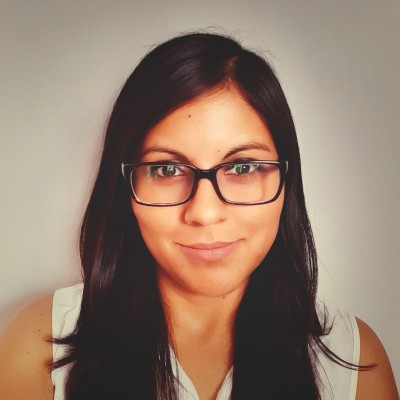
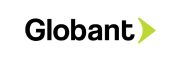