How to work with arrays in C#?
Working with arrays in C# is fundamental for managing collections of data efficiently. An array is a fixed-size, ordered collection of elements of the same type. Here’s how you can work with arrays in C#:
- Declaring an Array:
To declare an array, specify the data type of its elements followed by square brackets `[]` and the array name. For example, `int[] numbers;` declares an integer array called `numbers`.
- Initializing an Array:
You can initialize an array at the time of declaration using curly braces `{}` to specify the elements. For instance, `int[] numbers = {1, 2, 3, 4, 5};` initializes an integer array with values.
- Accessing Elements:
Access elements of an array using square brackets and the index, starting from zero. For example, `int firstNumber = numbers[0];` retrieves the first element of the `numbers` array.
- Modifying Elements:
You can change the value of an array element by assigning a new value. For instance, `numbers[1] = 10;` sets the second element to 10.
- Array Length:
Get the length (number of elements) of an array using the `Length` property. For example, `int length = numbers.Length;` retrieves the length of the `numbers` array.
- Iterating Through an Array:
Use loops like `for` or `foreach` to iterate through array elements. For example:
```csharp foreach (int number in numbers) { Console.WriteLine(number); } ```
- Multidimensional Arrays:
C# supports multidimensional arrays, such as two-dimensional arrays (`int[,] matrix`) or jagged arrays (`int[][] jaggedArray`), which are arrays of arrays.
- Array Methods:
C# provides various methods for manipulating arrays, including `Sort()`, `Reverse()`, and `IndexOf()`, which simplify common array operations.
Working with arrays is essential for tasks like data storage, manipulation, and processing in C#. Understanding array fundamentals is a crucial step towards more advanced data structures and algorithms.
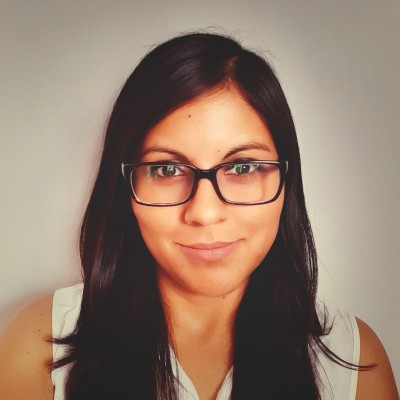
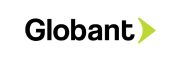