Implementing Email Functionality in CakePHP Applications
In today’s digital world, communication plays a pivotal role in the success of any application. Email remains one of the most versatile and widely used methods of communication, making it essential to integrate email functionality into your CakePHP applications. In this guide, we’ll explore the step-by-step process of implementing email functionality to enhance user engagement and interaction.
Table of Contents
1. Why Email Functionality Matters
Before we dive into the technical aspects, let’s discuss why integrating email functionality is crucial for your CakePHP applications.
1.1. User Engagement:
Emails are a direct and personal way to engage with users. Whether it’s sending account activation links, password reset instructions, or personalized notifications, emails help you maintain a strong connection with your users.
1.2. Communication:
Emails enable effective communication between your application and its users. You can provide updates about new features, promotions, or important announcements through email, ensuring that users stay informed.
1.3. User Experience:
Well-designed and timely emails can enhance the overall user experience. Sending confirmation emails for actions taken within the application instills confidence and trust in your users.
1.4. Marketing and Retention:
Emails can also serve as a powerful marketing tool. You can implement email campaigns to re-engage users who haven’t interacted with your application in a while, offering incentives or highlighting new features.
2. Setting Up Email Configuration
Before you can start sending emails, you need to configure your CakePHP application to use the appropriate email settings. CakePHP provides a flexible and customizable email configuration system.
Step 1: Open Config/app.php
Navigate to config/app.php in your CakePHP application. Look for the ‘EmailTransport’ and ‘Email’ sections within the ‘Email’ configuration array.
Step 2: Configure Email Transport
Choose an email transport method based on your needs. CakePHP supports various transport options, including ‘smtp’, ‘mail’, and ‘debug’ for testing purposes. For example, to configure the SMTP transport, modify the ‘EmailTransport’ section as follows:
php 'EmailTransport' => [ 'default' => [ 'className' => 'Smtp', 'host' => 'smtp.example.com', 'port' => 587, 'username' => 'your_username', 'password' => 'your_password', 'tls' => true, ], ],
Replace the placeholder values with your actual SMTP server details.
Step 3: Configure Email Settings
In the same Config/app.php file, locate the ‘Email’ section and set default email settings:
php 'Email' => [ 'default' => [ 'transport' => 'default', 'from' => 'you@example.com', 'charset' => 'utf-8', 'headerCharset' => 'utf-8', ], ],
Adjust the ‘from’ address and other settings according to your preferences.
3. Sending Basic Emails
With the email configuration in place, you’re ready to start sending basic emails from your CakePHP application.
Step 1: Load the Email Component
In your controller, load the Email component by adding the following line at the top of your controller file:
php use Cake\Mailer\Email;
Step 2: Create and Send an Email
Now you can create an instance of the Email class and send a basic email:
php $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Hello from CakePHP') ->send('This is a basic email sent from CakePHP.');
Replace ‘recipient@example.com’ with the recipient’s email address and customize the subject and message as needed.
4. Sending HTML Emails
To make your emails visually appealing and informative, you can send HTML-formatted emails using CakePHP.
Step 1: Create a Template
First, create an email template in src/Template/Email/html/example.ctp. You can structure it using HTML and CakePHP’s template variables:
html <!DOCTYPE html> <html> <head> <title><?= $subject ?></title> </head> <body> <h1><?= $messageTitle ?></h1> <p><?= $messageContent ?></p> </body> </html>
Step 2: Send HTML Email
Modify your controller code to send an HTML email using the template:
php $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('CakePHP HTML Email') ->viewBuilder() ->setTemplate('html/example') ->setVars([ 'subject' => 'CakePHP HTML Email', 'messageTitle' => 'Hello there!', 'messageContent' => 'This is an HTML email sent from CakePHP.', ]) ->send();
5. Sending Attachments
You can also enhance your emails by including attachments, such as images or documents.
Step 1: Attach Files
To attach files, use the attachments() method:
php $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Email with Attachment') ->viewBuilder() ->setTemplate('html/example') ->setVars([ 'subject' => 'Email with Attachment', 'messageTitle' => 'Check out this attachment!', 'messageContent' => 'An attachment is included.', ]) ->attachments([ 'document.pdf' => [ 'file' => WWW_ROOT . 'files' . DS . 'document.pdf', 'mimetype' => 'application/pdf', ], ]) ->send();
Replace ‘document.pdf’ with the desired attachment name and update the file path and MIME type accordingly.
Conclusion
Integrating email functionality into your CakePHP application opens up a world of possibilities for user engagement, communication, and marketing. By following the steps outlined in this guide, you can seamlessly implement email features such as sending basic emails, HTML-formatted emails, and attachments. Stay connected with your users and provide them with valuable updates and information, enhancing their overall experience with your application.
Table of Contents
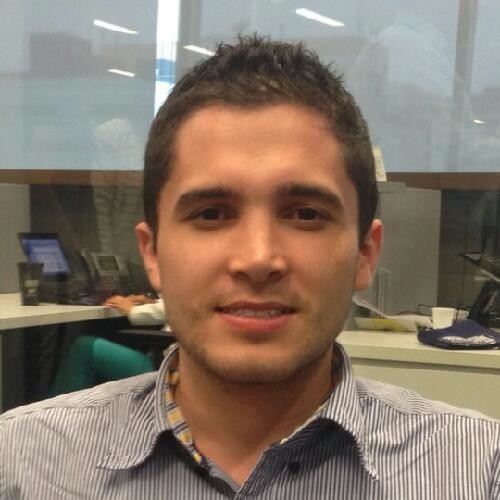
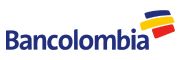