CakePHP and Firebase Integration: Real-Time Data Syncing
In today’s fast-paced digital world, real-time data syncing is becoming increasingly essential for web applications. Users expect instant updates, and businesses need to stay competitive by providing seamless, up-to-the-second interactions. To meet these demands, integrating Firebase with CakePHP can be a game-changer. In this comprehensive guide, we will explore how to achieve real-time data syncing through CakePHP and Firebase integration. We’ll cover everything from setting up Firebase to implementing it within your CakePHP application, with detailed code samples and best practices.
Table of Contents
1. Why CakePHP and Firebase Integration?
Before diving into the technical details, it’s crucial to understand why integrating CakePHP with Firebase is a smart move for real-time data syncing in web applications.
1.1. Real-Time Updates
Firebase offers real-time database capabilities, allowing your CakePHP application to push updates to clients instantly. Whether it’s a chat application, collaborative tool, or live dashboard, Firebase ensures that changes are reflected in real-time without the need for constant polling.
1.2. Scalability
Firebase is built to scale effortlessly. As your CakePHP application grows, Firebase can handle increased loads without a hitch. This scalability ensures your real-time features remain reliable and responsive even as your user base expands.
1.3. Cross-Platform Compatibility
Firebase works seamlessly across various platforms, including web, iOS, Android, and even desktop applications. This cross-platform compatibility allows you to deliver real-time experiences to users, regardless of their chosen device or operating system.
1.4. Serverless Architecture
Firebase offers serverless functionality, meaning you can focus on your application’s logic without the need to manage servers. This reduces operational overhead, enabling you to develop and deploy features faster.
Now that you understand the benefits, let’s walk through the steps to integrate CakePHP and Firebase for real-time data syncing.
2. Prerequisites
Before you begin, ensure you have the following prerequisites in place:
2.1. CakePHP Project
You should have a working CakePHP project ready for integration. If you haven’t already, you can create a new CakePHP project or use an existing one.
2.2. Firebase Account
Sign up for a Firebase account if you don’t already have one. Firebase offers a generous free tier, making it accessible for projects of all sizes.
2.3. Firebase SDK
Install the Firebase JavaScript SDK into your CakePHP project. You can include it using npm or add the Firebase CDN to your HTML templates.
Now that you have the prerequisites in place, let’s move on to the integration steps.
3. Integration Steps
Step 1: Firebase Project Setup
- Create a Firebase Project: Log in to your Firebase account and create a new project. Give it a meaningful name related to your CakePHP application.
- Get Firebase Configuration: In your Firebase project settings, you’ll find a configuration object containing keys like apiKey, authDomain, projectId, and others. These keys are crucial for initializing Firebase in your CakePHP application.
Step 2: Firebase Initialization in CakePHP
In your CakePHP project, initialize Firebase by including the Firebase JavaScript SDK and configuring it with the keys obtained in the previous step.
javascript // Include Firebase SDK <script src="https://www.gstatic.com/firebasejs/9.0.2/firebase-app.js"></script> <script src="https://www.gstatic.com/firebasejs/9.0.2/firebase-database.js"></script> // Initialize Firebase <script> var firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; firebase.initializeApp(firebaseConfig); </script>
Ensure you replace YOUR_API_KEY, YOUR_AUTH_DOMAIN, and other placeholders with your Firebase project’s actual values.
Step 3: Real-Time Data Syncing
With Firebase initialized, you can now start syncing real-time data between your CakePHP backend and Firebase’s real-time database. Let’s consider a basic example of syncing chat messages.
Sending Messages from CakePHP to Firebase
php // CakePHP Controller Action to Send a Message public function sendMessage() { $messageData = $this->request->getData(); // Retrieve message data from the request // Save message to your CakePHP database // Push the message to Firebase $firebaseDatabase = Firebase::getInstance()->getDatabase(); $messagesRef = $firebaseDatabase->getReference('messages'); $messagesRef->push($messageData); // Respond to the client $this->set('message', $messageData); $this->set('_serialize', 'message'); }
Receiving Messages from Firebase in CakePHP
javascript // JavaScript code to listen for new messages from Firebase var messagesRef = firebase.database().ref('messages'); messagesRef.on('child_added', function(snapshot) { var message = snapshot.val(); // Handle the new message in your CakePHP frontend });
In this example, whenever a new message is added to Firebase’s real-time database, it triggers the child_added event, and CakePHP’s frontend can react to it in real-time.
Step 4: Authentication and Security Rules
Firebase provides robust authentication and security rules to protect your data. You can integrate Firebase Authentication to secure your CakePHP application and Firebase Realtime Database. This step ensures that only authenticated users can read and write data.
Step 5: Handling Disconnects and Reconnections
In real-time applications, it’s crucial to handle disconnects and reconnections gracefully. Firebase automatically manages these scenarios, ensuring that users stay connected even when network conditions are unstable. You can use Firebase’s onDisconnect method to clean up resources or update data when a client disconnects.
4. Best Practices
As you integrate CakePHP and Firebase for real-time data syncing, keep these best practices in mind:
4.1. Optimize Data Structure
Design your Firebase database structure to suit your application’s needs. Use Firebase’s NoSQL capabilities to efficiently organize and query your data.
4.2. Use Firebase Functions
Leverage Firebase Functions to run server-side logic for complex real-time interactions. This keeps your frontend code clean and responsive.
4.3. Monitor and Debug
Firebase offers a suite of monitoring and debugging tools to track performance, spot errors, and optimize your real-time features.
4.4. Think About Offline Support
Consider how your application will handle offline scenarios. Firebase provides offline support, but you should design your frontend to work gracefully when connectivity is lost.
Conclusion
Integrating CakePHP with Firebase empowers your web application with real-time data syncing capabilities that delight users and keep them engaged. By following the integration steps and best practices outlined in this guide, you can create seamless, responsive, and scalable real-time features for your CakePHP project. Stay ahead of the competition and provide your users with the real-time experiences they expect in today’s digital landscape. Happy coding!
Real-time data syncing is a key requirement for many modern web applications, and integrating CakePHP with Firebase can make this task significantly easier. This comprehensive guide has walked you through the why and how of integrating these technologies, from project setup to real-time data syncing and best practices. By following these steps and best practices, you can create robust, real-time features that will keep your users engaged and your application competitive in today’s fast-paced digital landscape. Happy coding!
Are you ready to enhance your CakePHP application with real-time capabilities? Start integrating Firebase today and unlock a world of possibilities for your web application. Whether it’s real-time chat, live updates, or collaborative tools, Firebase has you covered. Stay ahead of the curve, and keep your users engaged with seamless, real-time experiences. Happy coding!
Table of Contents
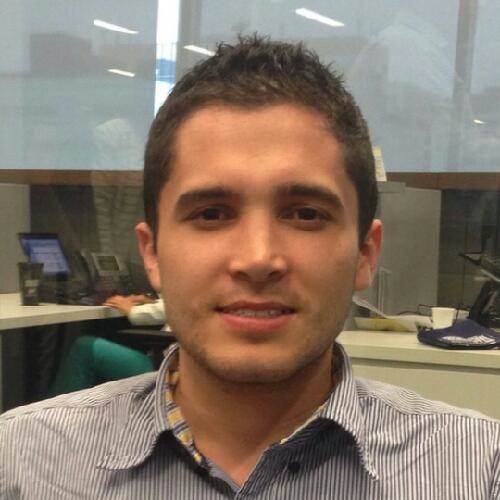
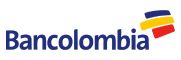