CakePHP Form Handling: Data Validation and Security
CakePHP is a popular PHP framework known for its simplicity and ease of use. One of the essential aspects of web application development is handling forms effectively, ensuring data validation, and maintaining security. In this blog, we’ll dive into CakePHP’s form handling capabilities, exploring data validation techniques and security measures to safeguard your application against vulnerabilities.
1. Understanding Form Handling in CakePHP:
Before we delve into data validation and security, let’s briefly understand how CakePHP handles forms. CakePHP provides a robust FormHelper class that simplifies the process of creating and handling forms. It assists in generating form elements, handling form submissions, and integrating data validation seamlessly.
2. Generating Forms with FormHelper:
Generating forms in CakePHP is straightforward, thanks to the FormHelper class. To create a basic form, you need to define the form’s start and end using the create() and end() methods, respectively. Within the form, you can add various input fields, such as text inputs, checkboxes, radio buttons, etc., using methods like input(), checkbox(), radio(), etc.
php // Sample CakePHP form creation using FormHelper echo $this->Form->create(); echo $this->Form->input('username'); echo $this->Form->input('password', ['type' => 'password']); echo $this->Form->checkbox('remember_me'); echo $this->Form->button('Submit'); echo $this->Form->end();
3. Data Validation in CakePHP:
Data validation is crucial to ensure that the data submitted via forms is accurate and meets the expected criteria. CakePHP offers a built-in validation system that allows developers to define validation rules for each form field easily.
3.1 Setting Up Validation Rules:
Validation rules are typically defined inside the model corresponding to the form data. CakePHP provides a validationDefault method in the model where you can specify these rules using a variety of built-in validation rules like ‘notEmpty’, ’email’, ‘numeric’, ‘maxLength’, etc.
php // Sample validation rules in a CakePHP model class User extends AppModel { public $validationDefault = array( 'username' => array( 'rule' => 'notEmpty', 'message' => 'Please enter your username.' ), 'email' => array( 'rule' => 'email', 'message' => 'Please enter a valid email address.' ), 'age' => array( 'rule' => 'numeric', 'message' => 'Please enter a valid numeric value for age.' ) ); }
3.2 Performing Validation:
Once the validation rules are defined, CakePHP automatically handles the validation process. When you submit a form, the data is first checked against the specified rules. If the data fails validation, CakePHP stores the error messages associated with each field, which can be accessed in the view to display appropriate error messages to the user.
php // Sample controller code handling form submission and validation class UsersController extends AppController { public function add() { if ($this->request->is('post')) { $this->User->set($this->request->data); if ($this->User->validates()) { // Data is valid, process the form submission } else { // Data validation failed, display error messages $errors = $this->User->validationErrors; $this->set('errors', $errors); } } } }
3.3 Displaying Validation Errors in the View:
In the view, you can access the validation errors and display them next to the corresponding form fields. CakePHP provides a simple method called error() for this purpose.
php // Sample view code to display validation errors echo $this->Form->create(); echo $this->Form->input('username'); echo $this->Form->error('username'); // Display username validation error, if any echo $this->Form->input('email'); echo $this->Form->error('email'); // Display email validation error, if any echo $this->Form->input('age'); echo $this->Form->error('age'); // Display age validation error, if any echo $this->Form->button('Submit'); echo $this->Form->end();
4. Handling Security in CakePHP Forms:
Securing your web application’s forms is as important as validating the data. CakePHP provides several built-in security features to prevent common vulnerabilities, such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
4.1 CSRF Protection:
CakePHP automatically includes CSRF protection by embedding CSRF tokens in forms. This prevents malicious users from exploiting your application by submitting unauthorized requests.
php // Sample form with CSRF protection in CakePHP echo $this->Form->create(); // CSRF token automatically added to the form echo $this->Form->input('username'); echo $this->Form->input('password', ['type' => 'password']); echo $this->Form->button('Submit'); echo $this->Form->end();
4.2 Sanitization:
In addition to data validation, CakePHP offers built-in mechanisms to sanitize the form data. Sanitization ensures that the data is free from any harmful content and protects against XSS attacks.
php // Sanitizing form data in CakePHP controller class UsersController extends AppController { public function add() { if ($this->request->is('post')) { $data = $this->request->getData(); $data = $this->User->sanitize($data); // Process the sanitized data } } }
Conclusion
CakePHP’s form handling capabilities provide developers with a powerful and secure way to process user-submitted data. By implementing proper data validation and security measures, you can safeguard your web application from potential vulnerabilities and ensure a smooth user experience. With the techniques discussed in this blog, you can confidently create robust and secure forms in your CakePHP applications. Happy coding!
Table of Contents
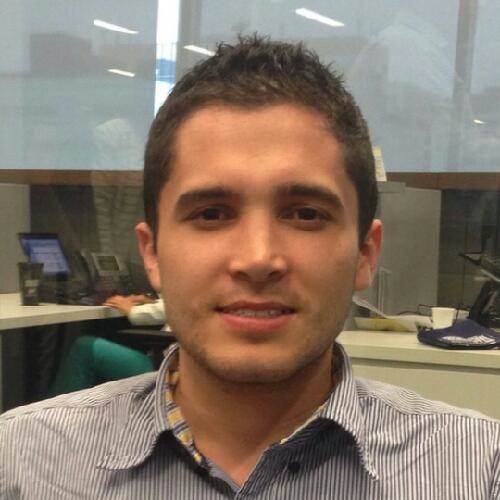
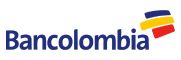