Getting Started with CakePHP: A Beginner’s Guide
CakePHP is a popular open-source framework written in PHP that allows developers to build robust and scalable web applications quickly. It follows the Model-View-Controller (MVC) architectural pattern, making it easy to separate concerns and maintain code readability. In this beginner’s guide, we will walk you through the process of getting started with CakePHP, from installation to building your first web application.
What is CakePHP?
CakePHP is a free and open-source PHP framework that simplifies web application development. It provides a set of libraries and tools to streamline common tasks, such as database access, form handling, and security. CakePHP follows the conventions-over-configuration principle, which means developers can focus on building their application’s unique features without having to worry about repetitive setup or configuration.
Installing CakePHP
To start using CakePHP, you need to have PHP installed on your system. Visit the official CakePHP website (cakephp.org) to download the latest stable version. Extract the downloaded archive to your web server’s document root or a directory of your choice.
Understanding the MVC Pattern
CakePHP follows the Model-View-Controller (MVC) architectural pattern. MVC separates the application logic into three interconnected components:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation and user interface.
- Controller: Controls the flow of the application, handling user requests and coordinating the model and view.
Understanding how MVC works is crucial for developing CakePHP applications. It promotes code organization, reusability, and maintainability.
Creating a New CakePHP Project
To create a new CakePHP project, open your command-line interface and navigate to the directory where you extracted CakePHP. Run the following command to create a new project:
shell $ composer create-project --prefer-dist cakephp/app myapp
This command will create a new CakePHP project named “myapp” in a directory called “myapp” relative to your current location.
Configuring Database Connection
CakePHP relies on a database for data storage. Open the config/app_local.php file in your project and locate the Datasources section. Update the ‘default’ configuration with your database details, such as hostname, database name, username, and password.
php 'Datasources' => [ 'default' => [ 'host' => 'localhost', 'port' => '3306', 'username' => 'your_username', 'password' => 'your_password', 'database' => 'your_database', ], ],
Building Models
Models in CakePHP represent the data and business logic of your application. Create a new model file in the src/Model/Table directory, following CakePHP’s naming conventions. For example, to create a PostsTable model, create a file named PostsTable.php with the following code:
php // src/Model/Table/PostsTable.php namespace App\Model\Table; use Cake\ORM\Table; class PostsTable extends Table { public function initialize(array $config): void { $this->addBehavior('Timestamp'); } }
Creating Controllers and Actions
Controllers handle the application logic and interact with models and views. Create a new controller file in the src/Controller directory, following CakePHP’s naming conventions. For example, to create a PostsController, create a file named PostsController.php with the following code:
php // src/Controller/PostsController.php namespace App\Controller; use App\Controller\AppController; class PostsController extends AppController { public function index() { $this->set('posts', $this->Posts->find('all')); } }
Designing Views
Views are responsible for the presentation and user interface of your application. Create a new view file in the src/Template/Posts directory. For example, to create an index.ctp view, create a file named index.ctp with the following code:
php <!-- src/Template/Posts/index.ctp --> <h1>Posts</h1> <table> <tr> <th>Title</th> <th>Created</th> </tr> <?php foreach ($posts as $post): ?> <tr> <td><?= $post->title ?></td> <td><?= $post->created->format('Y-m-d H:i:s') ?></td> </tr> <?php endforeach; ?> </table>
Routing URLs
CakePHP uses routing to map URLs to controllers and actions. Open the config/routes.php file in your project and define custom routes to control the URL structure. For example, to map the URL /posts to the index action of the PostsController, add the following code:
php // config/routes.php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::defaultRouteClass('DashedRoute'); Router::scope('/', function (RouteBuilder $routes) { $routes->connect('/posts', ['controller' => 'Posts', 'action' => 'index']); });
Working with Forms
CakePHP simplifies form handling and validation. In your view file, you can use CakePHP’s form helper to create forms easily. For example, to create a form that adds a new post, use the following code:
php <?= $this->Form->create(null, ['url' => ['controller' => 'Posts', 'action' => 'add']]) ?> <?= $this->Form->control('title') ?> <?= $this->Form->control('body', ['rows' => '3']) ?> <?= $this->Form->button('Add Post') ?> <?= $this->Form->end() ?>
Adding Validation and Security
CakePHP provides built-in validation rules to ensure data integrity. In your model file, you can define validation rules for each field. For example, to require a title and set a maximum length for the body field, add the following code to your model:
php // src/Model/Table/PostsTable.php use Cake\Validation\Validator; class PostsTable extends Table { public function validationDefault(Validator $validator): Validator { $validator ->requirePresence('title') ->notEmptyString('title') ->maxLength('body', 255); return $validator; } }
Testing and Debugging
CakePHP includes powerful testing and debugging tools. You can write test cases to ensure your application behaves as expected. To run tests, use the following command:
shell $ vendor/bin/phpunit
Additionally, CakePHP provides debugging utilities like the CakePHP Debug Kit, which offers insight into the application’s internal state during development.
Deploying Your CakePHP Application
To deploy your CakePHP application, ensure your web server is configured correctly. Set the document root to the webroot directory of your project. Additionally, configure your web server to rewrite URLs using the .htaccess file provided by CakePHP.
Conclusion
Congratulations! You have completed the beginner’s guide to CakePHP. You have learned how to install CakePHP, understand the MVC pattern, create models, controllers, and views, and work with forms and validation. CakePHP offers a wide range of features and tools that can enhance your web development experience. Explore the official documentation and experiment with different components to unlock the full potential of this powerful PHP framework.
With CakePHP, you can build dynamic web applications efficiently while following best practices and maintaining code quality. Start your CakePHP journey today and enjoy the benefits of this robust framework!
Table of Contents
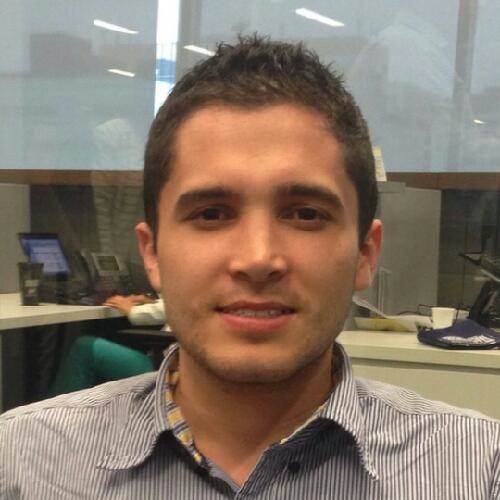
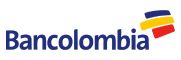