Working with CakePHP’s Http Client: Sending HTTP Requests
In the world of web development, sending HTTP requests is a fundamental task. Whether you’re fetching data from an API, posting form submissions, or interacting with external services, the ability to send HTTP requests programmatically is crucial. CakePHP, a popular PHP framework, provides a powerful tool for handling HTTP requests through its Http Client library. In this blog post, we’ll explore how to work with CakePHP’s Http Client, including sending GET and POST requests, handling responses, and utilizing advanced features.
Table of Contents
1. Getting Started with CakePHP’s Http Client
Before we dive into the nitty-gritty of sending HTTP requests, let’s make sure you have CakePHP set up. If you haven’t already, you can install CakePHP using Composer:
bash composer create-project --prefer-dist cakephp/app myapp
This command creates a new CakePHP project named myapp in the current directory.
Now, let’s explore how to use CakePHP’s Http Client to send HTTP requests.
1.1. Sending a GET Request
Sending a GET request with CakePHP’s Http Client is straightforward. You need to create an instance of the HttpClient class and use it to make the request. Here’s a basic example:
php use Cake\Http\Client; $client = new Client(); $response = $client->get('https://api.example.com/data'); // Get the response body as a string $body = $response->getBody()->getContents(); // Decode JSON response $data = json_decode($body, true); // Use the data print_r($data);
In this example, we create an instance of the HttpClient class and then use the get() method to send a GET request to https://api.example.com/data. We retrieve the response body, decode it as JSON, and use the data as needed.
1.2. Sending a POST Request
Sending a POST request is just as simple as sending a GET request. You can use the post() method of the HttpClient class to send data along with your request. Here’s an example:
php use Cake\Http\Client; $client = new Client(); $data = [ 'username' => 'myusername', 'password' => 'mypassword', ]; $response = $client->post('https://api.example.com/login', $data); // Get the response body as a string $body = $response->getBody()->getContents(); // Decode JSON response $data = json_decode($body, true); // Use the data print_r($data);
In this example, we send a POST request to https://api.example.com/login with the $data array containing the username and password. As before, we retrieve and process the response.
1.3. Handling Responses
CakePHP’s Http Client provides various methods to handle HTTP responses effectively. Let’s explore some common techniques.
1.3.1. Getting Response Status Code
You can retrieve the HTTP status code from the response like this:
php $status = $response->getStatusCode();
This code will give you the HTTP status code (e.g., 200 for a successful request, 404 for not found, etc.), allowing you to perform conditional logic based on the response status.
1.3.2. Checking for Success
To check if a request was successful (status code 2xx), you can use the isSuccess() method:
php if ($response->isSuccess()) { // Request was successful } else { // Request failed }
This can be handy for handling errors or taking different actions based on the success of your request.
1.3.3. Handling Headers
You can access response headers using the getHeaders() method:
php $headers = $response->getHeaders();
This returns an array of headers, which can be useful for extracting information like authentication tokens or handling specific headers from the response.
1.4. Advanced Features
CakePHP’s Http Client offers several advanced features that can enhance your HTTP request handling capabilities.
1.4.1. Handling Cookies
To work with cookies in your requests, you can use the CookieTrait. Here’s how to set and retrieve cookies:
php use Cake\Http\Client; use Cake\Http\Client\CookieTrait; $client = new Client(); $client->setConfig('cookies', new CookieTrait()); // Set a cookie $client->cookie('myCookie', 'myValue'); // Send a request and include cookies $response = $client->get('https://api.example.com/resource'); // Retrieve cookies from the response $cookies = $response->getCookies(); // Access a specific cookie $myCookieValue = $cookies['myCookie']->getValue();
This allows you to manage and interact with cookies, which is often necessary for maintaining sessions or handling authentication.
1.4.2. Sending JSON Requests
When dealing with APIs that expect JSON data in the request body, you can easily send JSON requests with CakePHP’s Http Client:
php use Cake\Http\Client; $client = new Client(); $data = [ 'key' => 'value', 'nested' => ['foo' => 'bar'], ]; $response = $client->post('https://api.example.com/endpoint', json_encode($data), [ 'type' => 'json', ]); // Handle the response
In this example, we use the json_encode() function to convert our data to JSON and specify the content type as JSON using the type option.
2. Error Handling and Exception Handling
When working with HTTP requests, it’s essential to handle errors gracefully. CakePHP’s Http Client can throw exceptions when something goes wrong, allowing you to catch and handle them accordingly. Here’s an example:
php use Cake\Http\Client; use Cake\Http\Client\Exception\HttpException; $client = new Client(); try { $response = $client->get('https://api.example.com/nonexistent'); } catch (HttpException $e) { // Handle the exception echo 'Error: ' . $e->getMessage(); }
In this example, we catch an HttpException and handle it by printing an error message. You can customize your error handling logic based on your application’s requirements.
Conclusion
Working with CakePHP’s Http Client provides a powerful and efficient way to send HTTP requests in your PHP applications. Whether you need to fetch data from external sources, interact with APIs, or handle form submissions, CakePHP’s Http Client simplifies the process and offers advanced features for more complex scenarios.
In this blog post, we’ve covered the basics of sending GET and POST requests, handling responses, and utilizing advanced features such as cookie management and JSON requests. Armed with this knowledge, you can confidently integrate HTTP request functionality into your CakePHP applications, making them more versatile and capable of interacting with external services.
If you’re working with CakePHP, take advantage of the Http Client library to streamline your HTTP request handling and make your web applications more robust and feature-rich. Happy coding!
Table of Contents
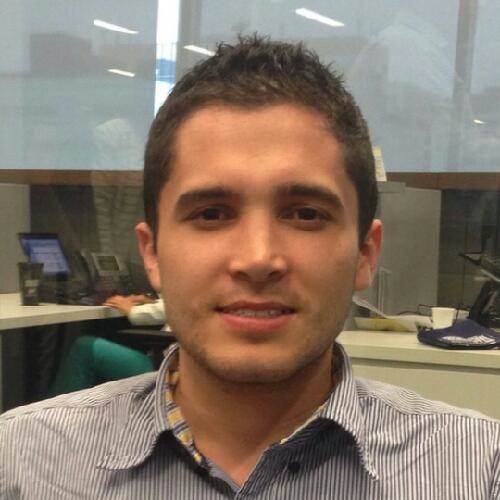
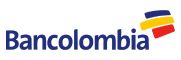