Working with CakePHP’s Image Processing Library: Imagine
Image processing is a crucial aspect of modern web development. Whether it’s resizing images for responsive designs, cropping avatars, or applying filters to enhance aesthetics, developers need powerful tools to handle these tasks effectively. CakePHP, a popular PHP framework, offers the Imagine plugin, which simplifies image manipulation with its easy-to-use interface. In this guide, we’ll explore how to work with CakePHP’s Imagine library to perform a variety of image processing tasks, complete with code examples and step-by-step instructions.
Table of Contents
1. Introduction to Imagine and CakePHP Integration
1.1. What is Imagine?
Imagine is a PHP library that provides a comprehensive set of tools for working with images. It allows developers to perform tasks such as resizing, cropping, rotating, and applying filters to images. Imagine supports multiple image formats and provides a fluent and intuitive API, making it an ideal choice for image manipulation tasks in web applications.
1.2. Integration with CakePHP
Imagine can be seamlessly integrated into CakePHP applications through the Imagine plugin. This plugin provides a wrapper around the Imagine library, allowing developers to take advantage of its capabilities within the CakePHP framework. To get started, ensure that you have a CakePHP project set up and running.
2. Installing the Imagine Plugin
2.1. Installation Steps
To install the Imagine plugin for CakePHP, follow these steps:
Step 1: Using Composer
Open a terminal and navigate to your CakePHP project’s root directory. Then, run the following Composer command:
bash composer require cakephp/imagine:dev-master
This command fetches the Imagine plugin and adds it to your project’s dependencies.
Step 2: Loading the Plugin
Next, load the Imagine plugin by adding the following line to your config/bootstrap.php file:
php Plugin::load('Imagine', ['bootstrap' => true, 'routes' => true]);
The plugin is now ready to be used within your CakePHP application.
3. Performing Basic Image Manipulations
3.1. Resizing Images
Imagine makes resizing images a breeze. Let’s say you have an image named “example.jpg” located in the webroot/img directory, and you want to resize it to a width of 300 pixels while maintaining its aspect ratio. Here’s how you can achieve this using Imagine in CakePHP:
Step 1: Importing the Necessary Classes
In your controller or wherever you’re processing the image, import the necessary classes:
php use Imagine\Image\Box; use Imagine\Image\Point; use Imagine\Gd\Imagine; // You can choose a different adapter if desired
Step 2: Resizing the Image
php // Load the image using Imagine $imagine = new Imagine(); $image = $imagine->open(WWW_ROOT . 'img' . DS . 'example.jpg'); // Calculate the new height while maintaining the aspect ratio $originalSize = $image->getSize(); $width = 300; $newHeight = $originalSize->getHeight() * ($width / $originalSize->getWidth()); // Create a new Box instance for the resized dimensions $newSize = new Box($width, $newHeight); // Resize the image $resizedImage = $image->resize($newSize); // Save the resized image $resizedImage->save(WWW_ROOT . 'img' . DS . 'resized_example.jpg');
3.2. Cropping Images
Cropping images is another common task in web development. Imagine simplifies this process as well. Suppose you have an image named “avatar.jpg” and you want to crop it to a square shape with a size of 200×200 pixels, focusing on the center of the image. Here’s how you can achieve this using Imagine in CakePHP:
Step 1: Load the Image
php // Load the image using Imagine $imagine = new Imagine(); $image = $imagine->open(WWW_ROOT . 'img' . DS . 'avatar.jpg');
Step 2: Calculate Crop Position and Dimensions
php // Get the original image dimensions $originalSize = $image->getSize(); $originalWidth = $originalSize->getWidth(); $originalHeight = $originalSize->getHeight(); // Calculate the crop dimensions $cropSize = min($originalWidth, $originalHeight); $cropWidth = $cropSize; $cropHeight = $cropSize; // Calculate the crop position to focus on the center $cropX = ($originalWidth - $cropWidth) / 2; $cropY = ($originalHeight - $cropHeight) / 2;
Step 3: Crop the Image
php // Crop the image $croppedImage = $image->crop(new Point($cropX, $cropY), new Box($cropWidth, $cropHeight)); // Resize the cropped image to 200x200 pixels (optional) $resizedImage = $croppedImage->resize(new Box(200, 200)); // Save the cropped and resized image $resizedImage->save(WWW_ROOT . 'img' . DS . 'cropped_avatar.jpg');
4. Applying Filters to Images
4.1. Adding Filters
Imagine also allows you to apply various filters to images, giving you the power to enhance or transform them. Let’s say you have an image named “landscape.jpg” and you want to apply a grayscale filter to it. Here’s how you can achieve this using Imagine in CakePHP:
Step 1: Load the Image
php // Load the image using Imagine $imagine = new Imagine(); $image = $imagine->open(WWW_ROOT . 'img' . DS . 'landscape.jpg');
Step 2: Apply the Grayscale Filter
php // Apply the grayscale filter $grayscaleImage = $image->effects()->grayscale(); // Save the grayscale image $grayscaleImage->save(WWW_ROOT . 'img' . DS . 'grayscale_landscape.jpg');
Conclusion
CakePHP’s integration with the Imagine library provides developers with a powerful toolset for image processing tasks. From resizing and cropping images to applying filters, Imagine simplifies complex operations with its intuitive API. By following the steps and examples outlined in this guide, you can leverage the capabilities of Imagine to enhance the visual appeal and functionality of your web applications. Whether you’re building an e-commerce site or a content-driven platform, mastering image manipulation with CakePHP and Imagine is a valuable skill that can take your projects to the next level. Start experimenting with the Imagine library today and unlock a new realm of creative possibilities in your web development journey.
Table of Contents
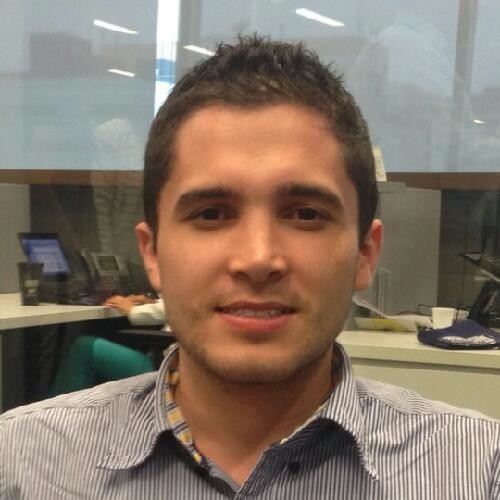
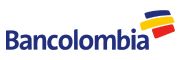