CakePHP Pagination: Efficiently Handling Large Data Sets
In the world of web development, managing large data sets is a common challenge that every developer faces at some point. Imagine a scenario where you’re working on an e-commerce website that needs to display a list of products to users. With thousands of products in the database, loading and displaying all of them at once would not only lead to a sluggish user experience but also put unnecessary strain on your server. This is where pagination comes to the rescue, and CakePHP provides a powerful toolset for efficiently handling such scenarios.
Table of Contents
1. Understanding Pagination
Pagination is a technique used to divide a large set of data into smaller, manageable chunks called “pages.” These pages are then displayed one at a time to the user, often accompanied by navigation controls like “Previous” and “Next.” This approach not only enhances user experience by providing faster load times but also optimizes server resources.
CakePHP, a popular PHP framework known for its elegant and efficient coding conventions, offers a robust pagination system that simplifies the implementation of pagination in your web applications. Let’s delve into the details of how CakePHP’s pagination works and how you can leverage it to efficiently manage large data sets.
2. Setting Up CakePHP Pagination
Before diving into the specifics of CakePHP’s pagination, make sure you have a CakePHP application up and running. If you’re new to CakePHP, the official documentation provides comprehensive guides to help you get started.
To begin using pagination in CakePHP, you need to include the Paginator component in your controller. Open the desired controller file and add the following line at the beginning of the class:
php use Cake\Controller\Component\PaginatorComponent;
Next, you’ll need to configure the pagination settings for your controller action. Within the action, you can define options such as the number of items per page, the current page, and conditions for fetching data. Here’s an example of how to set up pagination for a list of products:
php public function index() { $this->loadComponent('Paginator'); $query = $this->Products->find(); // Replace 'Products' with your model's name $this->paginate = [ 'limit' => 10, // Number of items per page 'order' => ['created' => 'desc'], // Order by creation date in descending order ]; $products = $this->paginate($query); $this->set('products', $products); }
In this example, the paginate method applies the configured settings to the query and fetches the appropriate data for the current page. The retrieved data is then passed to the view for rendering.
3. Displaying Pagination Controls
Once you have set up pagination in your controller, it’s time to display the pagination controls in your view. CakePHP provides convenient helpers for generating pagination links and controls. Here’s how you can use them:
php <!-- Inside your view file --> <div class="pagination"> <?= $this->Paginator->prev('Previous') ?> <?= $this->Paginator->numbers() ?> <?= $this->Paginator->next('Next') ?> </div>
The prev, numbers, and next methods generate the “Previous,” page numbers, and “Next” links, respectively. These links are automatically generated based on the pagination settings you defined earlier.
4. Efficiently Handling Large Data Sets
One of the key benefits of using CakePHP’s pagination is its ability to efficiently handle large data sets without overwhelming your server’s resources. When a user requests a specific page, CakePHP fetches only the necessary data for that page, rather than retrieving the entire dataset from the database. This optimized approach significantly improves load times and ensures a smooth browsing experience for your users.
Moreover, CakePHP’s pagination system seamlessly integrates with other features of the framework, such as data filtering, sorting, and searching. This means you can implement search functionalities, apply filters, and sort data columns—all while maintaining the benefits of pagination.
5. Advanced Pagination Techniques
CakePHP’s pagination system offers more than just basic pagination controls. You can implement advanced techniques to further enhance the user experience and provide more flexibility. Here are a few techniques you might find useful:
5.1. Customizing Pagination Settings Per Request
In some cases, you might want to customize the pagination settings based on specific user requests. CakePHP allows you to override the default pagination settings within your controller action. For instance, if you want to display a larger number of items per page for a particular view, you can do so easily:
php public function customView() { $this->paginate = [ 'limit' => 20, // Display 20 items per page for this view ]; $data = $this->paginate($this->Products); $this->set('data', $data); }
5.2. Using AJAX for Smooth Pagination
To provide an even more seamless browsing experience, you can implement AJAX-based pagination. This technique allows users to switch between pages without the need for a full page reload. With CakePHP’s flexibility, integrating AJAX-based pagination is a breeze. Simply set up your AJAX requests to fetch the paginated data and update the content dynamically.
javascript // Example AJAX call using jQuery $('.pagination a').on('click', function(event) { event.preventDefault(); var url = $(this).attr('href'); $.ajax({ url: url, type: 'GET', success: function(data) { $('#content').html(data); } }); });
5.3. Caching Pagination Results
In scenarios where your data doesn’t change frequently, you can leverage caching to further optimize pagination. By caching the paginated results, you reduce the need for repetitive database queries, leading to faster page load times. CakePHP supports various caching mechanisms, including file-based and database-based caching.
Conclusion
Efficiently handling large data sets is crucial for delivering a fast and seamless user experience in web applications. CakePHP’s pagination system empowers developers with a powerful toolset to tackle this challenge effectively. By dividing data into manageable pages, providing navigation controls, and offering advanced customization options, CakePHP ensures that your application can gracefully handle even the largest data sets without compromising performance.
Incorporating pagination not only enhances the user experience but also showcases your development skills in optimizing resource usage. Whether you’re building an e-commerce platform, a content-heavy blog, or any application with substantial data, CakePHP’s pagination will be your ally in creating responsive and efficient web experiences. So, dive into the world of CakePHP pagination and take your web development projects to the next level.
Table of Contents
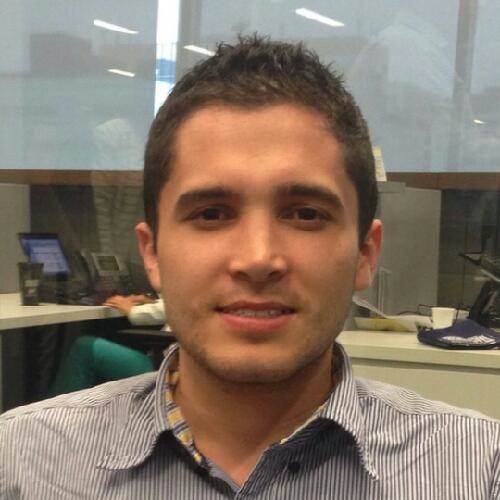
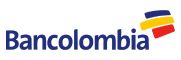