Mastering CakePHP Routing: A Comprehensive Guide
Routing plays a crucial role in any web application development framework, and CakePHP is no exception. It serves as the backbone of handling URLs and mapping them to the appropriate controllers and actions. In this comprehensive guide, we will delve deep into CakePHP routing and explore various techniques, configuration options, and code samples to help you master this essential aspect of CakePHP development.
1. Understanding CakePHP Routing
1.1 What is Routing?
Routing is the process of matching URLs to the appropriate controllers and actions in a web application. It enables the application to respond to different URLs and execute the corresponding code logic. In CakePHP, routing is handled by the Router class, which performs the mapping between URLs and controller actions.
1.2 How does CakePHP Routing Work?
CakePHP routing follows a convention-over-configuration approach, allowing developers to define custom routes while still providing sensible defaults. The Router class uses a set of predefined rules to match URLs against the available routes in your application’s routes.php configuration file.
2. Basic Routing
2.1 Default Routing Configuration
By default, CakePHP follows a simple routing scheme where the URL is mapped to a controller and an action. For example, the URL “/articles/view/1” would be mapped to the “view” action of the “Articles” controller, passing the parameter “1” as the article ID.
2.2 Creating Custom Routes
CakePHP provides a flexible routing system that allows you to define custom routes for your application. Custom routes can be created using the Router::connect() method, which takes a URL pattern and maps it to a specific controller and action.
2.3 Route Elements and Parameters
Routes can include elements and parameters that allow for dynamic and flexible URL patterns. Elements are enclosed in curly braces {} and act as placeholders for dynamic segments in the URL. Parameters are used to capture and pass specific values from the URL to the controller actions.
3. Advanced Routing Techniques
3.1 Route Conditions
CakePHP routing supports conditions that can be applied to routes to further refine their matching criteria. Conditions can be based on request methods, HTTP headers, or custom callback functions, allowing you to create more fine-grained routing rules.
3.2 Named Routes
Named routes provide a convenient way to generate URLs based on predefined routes. Instead of hard-coding URLs in your application, you can use the named routes to generate URLs dynamically, making your code more maintainable and flexible.
3.3 Prefix Routing
Prefix routing allows you to group a set of routes under a common URL prefix. This is useful when you want to apply specific routing rules to a group of related actions or controllers. For example, you can define a prefix for an administrative section of your application.
3.4 Scoped Routing
Scoped routing is similar to prefix routing but provides a more structured way of grouping routes. With scoped routing, you can define a scope for a set of routes, which encapsulates both the URL prefix and the routing rules. It helps organize routes and makes them easier to manage.
3.5 Reverse Routing
Reverse routing allows you to generate URLs based on the defined routes in your application. It is particularly useful when you have complex or dynamic routes and need to generate URLs dynamically in your views or controllers.
4. Routing Configuration
4.1 Route Classes
CakePHP provides the flexibility to define custom route classes that can be used for advanced routing scenarios. Route classes allow you to extend the default routing behavior and implement custom logic or parsing rules.
4.2 Persisting Routes
By default, routes in CakePHP are loaded from the routes.php configuration file on every request. However, you can optimize the routing performance by persisting the routes in the cache. This reduces the overhead of parsing and loading routes on every request, resulting in improved application performance.
4.3 Dynamic Routing
Dynamic routing enables you to define routes dynamically based on the data or configuration stored in your application. This is particularly useful when you want to create SEO-friendly URLs or handle dynamic content routing.
5. Code Samples
5.1 Simple Route Definition
php // routes.php use Cake\Routing\RouteBuilder; $routes->connect('/about', ['controller' => 'Pages', 'action' => 'display', 'about']);
5.2 Route with Parameters
php // routes.php use Cake\Routing\RouteBuilder; $routes->connect('/articles/:id', ['controller' => 'Articles', 'action' => 'view']) ->setPatterns(['id' => '\d+']) ->setPass(['id']);
5.3 Route with Conditions
php // routes.php use Cake\Routing\RouteBuilder; $routes->connect('/api', ['controller' => 'Api', 'action' => 'index']) ->setMethods(['GET', 'POST']) ->setHeaders(['Accept' => 'application/json']);
5.4 Named Routes Example
php // routes.php use Cake\Routing\RouteBuilder; $routes->connect('/profile', ['controller' => 'Users', 'action' => 'profile'], ['_name' => 'profile']);
Conclusion
Mastering CakePHP routing is essential for building robust and flexible web applications. In this comprehensive guide, we have covered the basics of CakePHP routing, advanced techniques, routing configuration, and provided code samples to enhance your understanding. By applying these concepts and leveraging the power of CakePHP routing, you can create clean and user-friendly URLs while maintaining control over your application’s navigation and functionality.
Table of Contents
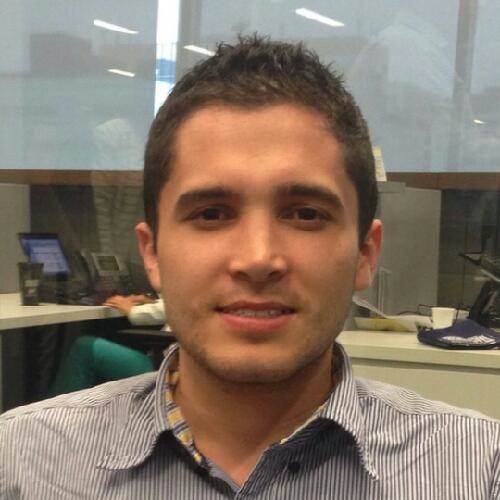
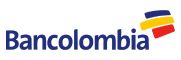