Using CakePHP’s Test Suite: Writing and Running Tests
In the realm of web development, ensuring the functionality and reliability of your applications is of paramount importance. Bugs and errors can quickly tarnish user experiences and damage your brand’s reputation. This is where testing comes into play. One of the most powerful tools at your disposal for testing web applications is CakePHP’s Test Suite. In this guide, we’ll walk you through the process of writing and running tests using CakePHP’s Test Suite to help you achieve more robust and dependable applications.
Table of Contents
1. Understanding the Importance of Testing
Before delving into the specifics of CakePHP’s Test Suite, let’s take a moment to understand why testing is crucial for web development.
1.1. Ensuring Functionality
Testing allows you to verify that your application works as intended. It helps you catch and rectify issues before they reach the users, leading to a smoother user experience.
1.2. Preventing Regressions
As you make updates or add new features, there’s a risk of inadvertently breaking existing functionality. Tests act as safety nets, ensuring that your changes don’t introduce regressions.
1.3. Saving Time and Resources
While writing tests initially requires an investment of time, it ultimately saves time and effort in the long run. Detecting and fixing bugs early on is far more cost-effective than addressing them after deployment.
2. Exploring CakePHP’s Test Suite
CakePHP, a popular PHP framework, provides a comprehensive Test Suite that covers different levels of testing: unit tests, integration tests, and functional tests.
2.1. Unit Tests
Unit tests focus on testing individual components or “units” of your application in isolation. This ensures that each part of your code functions correctly on its own.
To write a unit test in CakePHP, you can extend the TestCase class and use methods like testSomething() to define your tests. Here’s a simplified example:
php // File: ArticleTest.php use App\Model\Table\ArticleTable; use Cake\TestSuite\TestCase; class ArticleTest extends TestCase { public $fixtures = ['app.Articles']; public function setUp(): void { parent::setUp(); $this->Article = new ArticleTable(); } public function testGetArticleById() { $article = $this->Article->getArticleById(1); $this->assertEquals('First Article', $article->title); } }
2.2. Integration Tests
Integration tests take a step further by testing the interactions between various components. This ensures that different parts of your application work together seamlessly.
In CakePHP, integration tests involve more complex setups, often requiring database interactions. The Cake\I18n\Time class can be used to handle time-related assertions. Here’s a snippet:
php // File: CommentsIntegrationTest.php use Cake\ORM\TableRegistry; use Cake\TestSuite\IntegrationTestTrait; use Cake\TestSuite\TestCase; class CommentsIntegrationTest extends TestCase { use IntegrationTestTrait; public $fixtures = ['app.Articles', 'app.Comments']; public function testAddComment() { $this->post('/articles/add-comment/1', ['comment' => 'Great article']); $this->assertResponseSuccess(); $comments = TableRegistry::getTableLocator()->get('Comments'); $query = $comments->find()->where(['article_id' => 1]); $this->assertEquals(1, $query->count()); } }
2.3. Functional Tests
Functional tests provide an even higher level of testing by evaluating the entire application’s functionality from a user’s perspective. This involves simulating user interactions.
CakePHP’s Test Suite supports functional tests through the use of request and response objects. Here’s an example:
php // File: UsersControllerTest.php use Cake\TestSuite\IntegrationTestTrait; use Cake\TestSuite\TestCase; class UsersControllerTest extends TestCase { use IntegrationTestTrait; public function testLoginRedirect() { $this->get('/users/login'); $this->assertResponseOk(); $data = ['username' => 'user', 'password' => 'pass']; $this->post('/users/login', $data); $this->assertRedirect(['controller' => 'Dashboard', 'action' => 'index']); } }
3. Running CakePHP Tests
Once you’ve written your tests using CakePHP’s Test Suite, it’s time to run them and validate your application’s behavior.
3.1. Using the Test Shell
CakePHP provides a Test Shell, a command-line tool that allows you to run your tests. Navigate to your application’s root directory and execute:
bash bin/cake test
You can also target specific test cases, test methods, or plugins using various command-line options.
3.2. Integrating with Continuous Integration
To maintain a robust testing workflow, consider integrating your CakePHP tests with a continuous integration (CI) system like Jenkins, Travis CI, or GitHub Actions. This ensures that your tests are automatically run whenever new code is pushed, providing early feedback on any potential issues.
Conclusion
CakePHP’s Test Suite offers a powerful toolkit for ensuring the reliability and functionality of your web applications. By writing unit tests, integration tests, and functional tests, you can catch bugs early and create a more polished user experience. Remember, testing is not just a one-time activity; it’s an ongoing process that contributes to the long-term success of your projects. So, embrace testing, make it an integral part of your development workflow, and enjoy the benefits of more stable and dependable applications.
Table of Contents
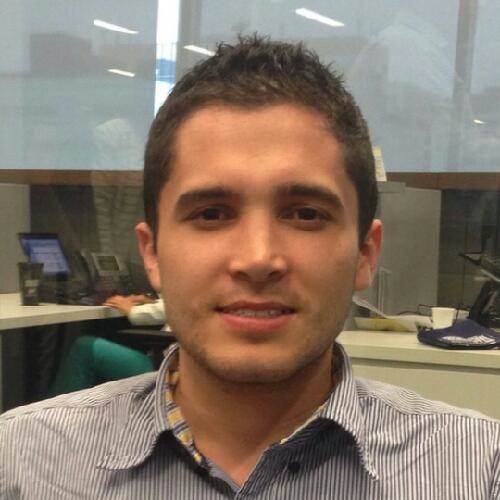
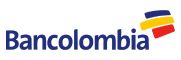