Getting Started with Dart: A Beginner’s Guide
Are you interested in learning a new programming language that can be used for web and mobile app development? Look no further than Dart! Dart is a powerful, object-oriented language developed by Google. It is designed to be fast, flexible, and easy to learn. In this beginner’s guide, we will introduce you to the basics of Dart, its features, syntax, and guide you through building your first web and mobile applications.
Introduction to Dart
Dart is a general-purpose programming language that can be used for developing web, mobile, and desktop applications. It was created by Google and was first announced in 2011. Dart combines the best features of languages like JavaScript, Java, and C#. It is a statically-typed language, which means that variables must have a specific type assigned to them. Dart supports both object-oriented and functional programming paradigms, making it a versatile language for various types of applications.
Setting Up Dart Development Environment
Before we start coding in Dart, we need to set up our development environment. Dart has excellent tooling support that makes development smooth and efficient. Here are the steps to get started:
- Download and install the Dart SDK from the official Dart website.
- Set up your preferred code editor or IDE. Popular choices include Visual Studio Code, IntelliJ IDEA, and Android Studio.
- Configure the Dart SDK in your code editor or IDE.
- Verify the installation by running dart –version in your terminal/command prompt.
With the development environment set up, we are ready to write our first Dart program.
Dart Syntax and Basics
Let’s start by understanding the basic syntax and structure of Dart programs. Dart uses a C-style syntax that is familiar to many programmers. Here’s an example of a simple “Hello, World!” program in Dart:
dart void main() { print('Hello, World!'); }
In Dart, the main() function is the entry point of the program. We use the print() function to display the output. Dart has a strong type system, but it also supports type inference, allowing you to omit type annotations in many cases.
Variables and Data Types in Dart
Variables are used to store values in Dart. Dart provides several data types, including int, double, String, bool, and more. You can declare variables using the var keyword or specify the type explicitly. Here’s an example:
dart var message = 'Hello, Dart!'; int age = 25; double piValue = 3.14159; bool isDartAwesome = true;
Dart also supports type inference, so you can omit the type annotation and let the compiler infer the type based on the assigned value.
Control Flow and Loops in Dart
Control flow statements allow you to conditionally execute code blocks or repeat code multiple times. Dart provides if-else statements for conditional execution, for and while loops for iteration, and switch statements for multi-way branching. Here’s an example that demonstrates these control flow statements:
dart int num = 5; if (num > 0) { print('Positive'); } else if (num < 0) { print('Negative'); } else { print('Zero'); } for (int i = 0; i < 5; i++) { print(i); } int count = 0; while (count < 3) { print('Dart is fun!'); count++; }
Functions in Dart
Functions in Dart are used to group a set of statements together and give them a name. They help in organizing and reusing code. Dart supports both named and anonymous functions. Here’s an example of a named function:
dart void greet(String name) { print('Hello, $name!'); } // Calling the function greet('Alice');
In Dart, functions can also have optional parameters, default parameter values, and can return values.
Object-Oriented Programming in Dart
Dart is an object-oriented language, which means it supports classes and objects. You can create your own classes and define properties (attributes) and methods (behavior) for those classes. Here’s an example:
dart class Person { String name; int age; void sayHello() { print('Hello, my name is $name.'); } } // Creating an object of the Person class var person = Person(); person.name = 'Alice'; person.age = 25; person.sayHello();
Dart also supports inheritance, interfaces, and other essential object-oriented programming concepts.
Working with Collections in Dart
Collections are used to store multiple values in Dart. Dart provides several collection types, including List, Set, and Map. Here are some examples:
dart // List var numbers = [1, 2, 3, 4, 5]; // Set var fruits = {'apple', 'banana', 'orange'}; // Map var person = { 'name': 'Alice', 'age': 25, };
Dart provides a rich set of methods and operators to manipulate collections efficiently.
Error Handling in Dart
Error handling is an essential part of any programming language. Dart provides try-catch-finally blocks to handle exceptions. Here’s an example:
dart try { // Some code that might throw an exception } catch (e) { // Handle the exception } finally { // Code that will always execute, whether an exception occurred or not }
Dart also supports custom exceptions and asynchronous error handling.
Building a Web Application with Dart
Dart can be used to build web applications using frameworks like Flutter and AngularDart. In this section, we’ll explore how to build a simple web application using Dart and the Flutter framework. We’ll cover topics like UI components, handling user input, and data management.
Building a Mobile Application with Dart
Dart’s real strength lies in its ability to build mobile applications using the Flutter framework. In this section, we’ll dive into Flutter and learn how to build a mobile application from scratch. We’ll cover topics like widgets, navigation, state management, and accessing native device features.
Conclusion
Congratulations! You have completed the beginner’s guide to Dart programming. You’ve learned the basics of Dart, including its syntax, data types, control flow, functions, object-oriented programming, collections, error handling, and building web and mobile applications. Dart is a versatile language that empowers developers to create robust and efficient applications. Now, it’s time to take what you’ve learned and start building your own projects with Dart!
We hope this guide has provided you with a solid foundation to continue your Dart journey. Keep exploring the language, experimenting with different projects, and leveraging the rich ecosystem of libraries and frameworks available. Happy coding with Dart!
Table of Contents
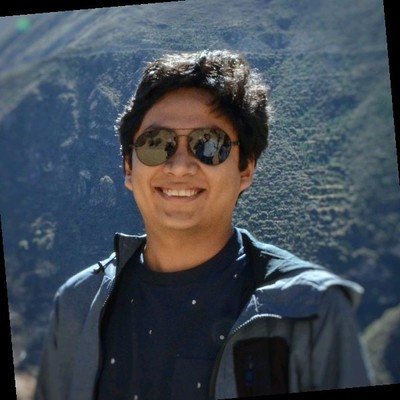
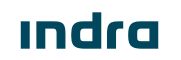