Dart for IoT Development: Building Smart Connected Devices
The Internet of Things (IoT) has transformed the way we interact with the world around us. From smart homes to industrial automation, IoT devices have become an integral part of our lives. To develop efficient and powerful IoT devices, choosing the right programming language is crucial. Dart, a versatile language developed by Google, is gaining popularity in the IoT community due to its performance, ease of use, and cross-platform capabilities. In this blog, we will explore the capabilities of Dart for IoT development and demonstrate how to build smart connected devices using this cutting-edge language.
Why Choose Dart for IoT Development?
Before delving into building smart connected devices, let’s understand why Dart is an excellent choice for IoT development.
- Performance: Dart is a compiled language that delivers impressive performance. It allows developers to write fast and efficient code, making it ideal for resource-constrained IoT devices that require real-time responsiveness.
- Cross-platform Support: Dart’s compatibility with multiple platforms is a significant advantage for IoT development. It can be used on various operating systems, including Linux, Windows, and macOS, facilitating seamless integration with different IoT hardware.
- Strong Typing and Safety: Dart’s static type system reduces the chances of runtime errors, enhancing the reliability and safety of IoT applications. This is particularly crucial when dealing with critical tasks or handling sensitive data in IoT devices.
- Asynchronous Programming: IoT devices often require handling multiple tasks concurrently. Dart’s native support for asynchronous programming using async and await simplifies managing concurrent operations and event-driven programming.
- Rich Standard Library: Dart’s standard library provides numerous built-in functions and packages, enabling rapid development and reducing the need for external dependencies.
- Flutter Ecosystem: Dart is the language of choice for building mobile and web applications with Flutter. This makes it easy to create a unified ecosystem, enabling seamless integration between IoT devices and their companion apps.
Now that we understand the advantages of using Dart for IoT development, let’s dive into building our smart connected device.
Building a Smart Thermostat with Dart
In this section, we will walk through the process of building a smart thermostat using Dart. The smart thermostat will be capable of reading temperature data, adjusting settings remotely, and sending alerts when certain conditions are met.
1. Setting up the Environment
Before we start coding, ensure that you have Dart SDK installed on your development machine. You can download the latest version of the Dart SDK from the official Dart website.
Once Dart is installed, create a new Dart project and set up the necessary dependencies for our smart thermostat.
2. Reading Temperature Data
To read temperature data from a sensor, we need to interface with it using appropriate hardware. For this example, we’ll assume we have a temperature sensor connected to an analog-to-digital converter (ADC) on our IoT device.
Below is a code snippet to read temperature data from the sensor using Dart:
dart import 'dart:io'; // Assuming the ADC is connected to GPIO pin 1 const int temperatureSensorPin = 1; void main() { // Set up GPIO pin var gpio = File('/sys/class/gpio/gpio$temperatureSensorPin/value'); gpio.writeAsStringSync('in'); // Function to read temperature from ADC double readTemperature() { var rawValue = int.parse(gpio.readAsStringSync().trim()); // Convert raw ADC value to temperature in Celsius double temperature = (rawValue * 0.48876) - 50.0; return temperature; } // Read temperature and print print('Current Temperature: ${readTemperature()}°C'); }
In this code, we read the raw ADC value from the GPIO pin connected to the temperature sensor and convert it into Celsius using a simple formula.
3. Implementing Remote Access
To make our thermostat “smart,” we need to provide a way to access and control it remotely. We can achieve this by setting up a simple web server using the shelf package, which is part of Dart’s standard library.
Below is a code snippet to create a basic web server that allows us to control the thermostat remotely:
dart import 'dart:io'; import 'package:shelf/shelf.dart'; import 'package:shelf/shelf_io.dart' as io; // Global variable to store the desired temperature double desiredTemperature = 20.0; // Handler for the web server Response handleRequest(Request request) { if (request.url.path == '/temperature') { if (request.method == 'GET') { // Respond with the current temperature return Response.ok('Current Temperature: ${readTemperature()}°C'); } else if (request.method == 'POST') { // Set the desired temperature var newTemperature = double.tryParse(request.readAsStringSync()); if (newTemperature != null) { desiredTemperature = newTemperature; return Response.ok('Desired Temperature set to $desiredTemperature°C'); } else { return Response.badRequest('Invalid Temperature Value'); } } } return Response.notFound('Not Found'); } void main() async { var handler = const Pipeline().addMiddleware(logRequests()).addHandler(handleRequest); var server = await io.serve(handler, 'localhost', 8080); print('Server running on localhost:${server.port}'); }
In this code, we create a web server that listens on port 8080 for incoming requests. When a GET request is made to the “/temperature” endpoint, the server responds with the current temperature. When a POST request is made with a new temperature value in the request body, the server updates the desired temperature.
4. Sending Alerts
To make our smart thermostat even smarter, let’s implement an alert mechanism that notifies the user when the temperature exceeds a predefined threshold. We’ll use the dart:io package to send email notifications for simplicity.
Below is a code snippet to send email alerts when the temperature exceeds the threshold:
dart import 'dart:io'; import 'package:shelf/shelf.dart'; import 'package:shelf/shelf_io.dart' as io; import 'package:mailer/mailer.dart'; import 'package:mailer/smtp_server.dart'; const double temperatureThreshold = 25.0; const String senderEmail = 'your_email@example.com'; const String senderPassword = 'your_email_password'; const String recipientEmail = 'recipient@example.com'; void sendEmailAlert() async { final smtpServer = gmail(senderEmail, senderPassword); final message = Message() ..from = Address(senderEmail) ..recipients.add(recipientEmail) ..subject = 'Temperature Alert' ..text = 'The current temperature is above the threshold (${readTemperature()}°C).'; try { final sendReport = await send(message, smtpServer); print('Message sent: ${sendReport.sent}'); } on MailerException catch (e) { print('Message not sent: $e'); } } void main() async { // Previous code for the web server // Continuously monitor temperature and send alerts if needed while (true) { var currentTemperature = readTemperature(); if (currentTemperature > temperatureThreshold) { sendEmailAlert(); } sleep(Duration(minutes: 5)); // Check temperature every 5 minutes } }
In this code, we continuously monitor the temperature and send an email alert if it exceeds the predefined threshold.
Conclusion
Dart’s versatility, performance, and cross-platform support make it an excellent choice for IoT development. In this blog, we explored the benefits of using Dart for building smart connected devices. We also walked through building a smart thermostat with Dart, showcasing its capabilities in handling IoT tasks.
With Dart’s growing ecosystem and community support, developers can now build robust and scalable IoT solutions, catering to various industries and applications. Embrace the power of Dart for IoT development and unlock the potential of smart connected devices in this ever-evolving IoT landscape. Happy coding!
Table of Contents
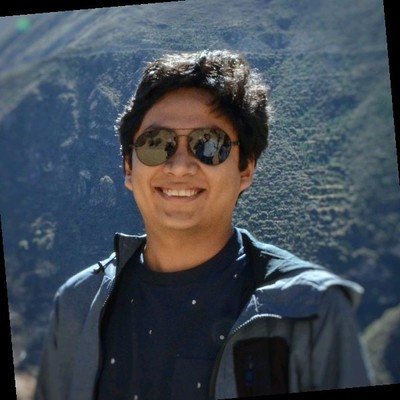
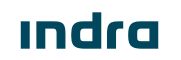