Machine Learning Deployment with Dart: Serving Models in Production
In today’s rapidly evolving technological landscape, machine learning has become a pivotal aspect of various applications and industries. From recommending personalized content on streaming platforms to autonomous driving systems, machine learning models are making significant contributions. However, developing and training a machine learning model is only half the battle; deploying these models effectively in production is equally important. This blog will delve into the realm of deploying machine learning models using Dart, providing insights into the process, tools, and benefits of serving models in production.
Table of Contents
1. Understanding the Deployment Process
Deploying a machine learning model involves making the model accessible to users or other software systems so that it can generate predictions or classifications. In the context of Dart, a versatile programming language developed by Google, deploying machine learning models entails incorporating the model into your Dart application, allowing it to function seamlessly within your production environment.
2. Benefits of Using Dart for Model Deployment
- Consistency: By using Dart for both application development and model deployment, you ensure a consistent codebase, simplifying maintenance and updates.
- Efficiency: Dart’s just-in-time (JIT) compilation and ahead-of-time (AOT) compilation options contribute to efficient execution, ensuring that your deployed models perform optimally.
- Interoperability: Dart’s interoperability with other programming languages and its ability to create native applications make it an excellent choice for integrating machine learning into various systems.
3. Tools for Deploying Machine Learning Models in Dart
To successfully deploy machine learning models in Dart, you’ll need a set of tools that streamline the process and ensure the best possible performance. Let’s explore some of these tools:
3.1. TensorFlow Dart
TensorFlow, a popular open-source machine learning library, has a dedicated package for Dart known as TensorFlow Dart. This package provides bindings to TensorFlow’s C API, allowing you to leverage TensorFlow’s capabilities directly within your Dart application.
Example: Loading and using a pre-trained TensorFlow model in Dart using the TensorFlow Dart package.
dart import 'package:tfdart/tf.dart'; void main() { final model = TfLiteModel.fromFile('path/to/your/model.tflite'); final interpreter = Interpreter.fromModel(model); final input = [1.0, 2.0, 3.0]; final output = List.filled(1 * 1, 0).reshape([1, 1]); interpreter.run(input, output); print('Prediction: ${output[0][0]}'); }
3.2. Dart FFI
Dart Foreign Function Interface (FFI) enables Dart code to call native C APIs directly. This is particularly useful when integrating with existing machine learning libraries or custom inference engines written in C or C++.
Example: Using Dart FFI to call a C function for model inference.
dart import 'dart:ffi'; import 'package:ffi/ffi.dart'; class Model extends Struct {} typedef ModelLoadFunction = Pointer<Model> Function(); typedef ModelRunFunction = Float Function(Pointer<Model>, Pointer<Float>); void main() { final modelLib = DynamicLibrary.open('path/to/model_library.so'); final loadModel = modelLib .lookupFunction<ModelLoadFunction, ModelLoadFunction>('load_model'); final runModel = modelLib .lookupFunction<ModelRunFunction, ModelRunFunction>('run_model'); final modelPtr = loadModel(); final input = calloc<Float>(inputSize); // Fill input with data final result = runModel(modelPtr, input); print('Prediction: $result'); calloc.free(input); }
3.3. Flutter for UI Integration
If you’re deploying machine learning models in Dart for mobile or web applications, Flutter provides an excellent platform for UI integration. Flutter’s declarative UI framework makes it seamless to incorporate machine learning-powered features into your application’s user interface.
Example: Using Flutter to create a simple mobile app that uses a deployed machine learning model.
dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'ML Model App', home: Scaffold( appBar: AppBar(title: Text('Machine Learning Model')), body: Center( child: ElevatedButton( onPressed: () { // Call the deployed model and display the result }, child: Text('Predict'), ), ), ), ); } }
4. Best Practices for Model Deployment in Dart
Deploying machine learning models involves not only integrating code but also ensuring reliability, scalability, and maintainability. Here are some best practices to consider:
4.1. Version Control for Models
Treat your machine learning models as code artifacts and manage them with version control systems like Git. This allows you to track changes, collaborate with team members, and easily revert to previous versions if needed.
4.2. Containerization
Consider using containerization tools like Docker to package your Dart application along with its dependencies, including the machine learning model. This simplifies deployment across different environments and ensures consistency.
4.3. Monitoring and Logging
Implement robust monitoring and logging mechanisms to keep track of your deployed models’ performance and identify potential issues promptly. Tools like Prometheus and Grafana can be invaluable for monitoring model health.
4.4. Security Considerations
Ensure that your deployed models are protected against potential security threats. Follow security best practices such as securing communication channels, applying proper authentication, and regularly updating dependencies.
4.5. Scalability
Design your deployment architecture to be scalable. This might involve using load balancers and deploying your application across multiple instances to handle increased traffic and demand.
Conclusion
Deploying machine learning models with Dart opens up a realm of possibilities for seamlessly integrating AI-powered capabilities into your applications. From TensorFlow Dart and Dart FFI to Flutter’s UI integration, Dart provides a versatile ecosystem for deploying models efficiently and effectively. By following best practices and considering factors like version control, containerization, and security, you can ensure a successful deployment that brings the power of machine learning to your production systems. As technology continues to advance, embracing the synergy between machine learning and Dart can lead to innovative and impactful solutions across industries.
Table of Contents
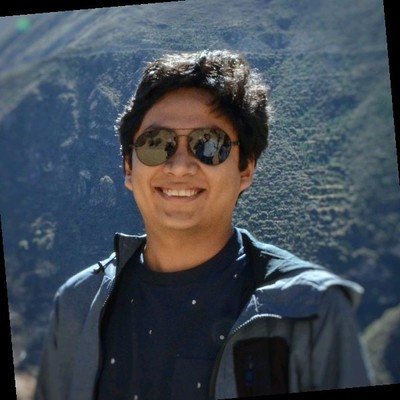
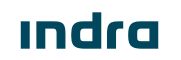