Reactive UI with Dart and Flutter: Building Dynamic User Interfaces
In the world of modern app development, creating user interfaces that are not only visually appealing but also responsive and dynamic is crucial. This is where reactive UI comes into play. Reactive UI is a paradigm that leverages reactive programming concepts to build interfaces that automatically update in response to changes in the underlying data. In this article, we will delve into the world of reactive UI with Dart and Flutter, showcasing how this approach can simplify and enhance the process of creating dynamic user interfaces. We’ll explore the core concepts, benefits, and provide hands-on code examples to get you started on your journey to building reactive UIs.
Table of Contents
1. Understanding Reactive UI:
Reactive UI revolves around the idea of observing and reacting to changes in data. This concept is particularly powerful in frameworks like Flutter, which embraces the reactive programming paradigm. At its core, reactive UI allows developers to express the user interface as a function of the underlying data, ensuring that the UI automatically updates whenever the data changes. This eliminates the need for manual UI updates and ensures a consistent and responsive user experience.
2. Key Concepts:
2.1. Streams and Observables:
In Dart and Flutter, streams and observables are the building blocks of reactive programming. Streams represent sequences of asynchronous events, while observables are the abstractions that allow us to react to changes in these streams. By using streams and observables, developers can establish a clear and reactive flow of data between different components of the application.
2.2. Widgets and Rebuilds:
Flutter widgets are the visual building blocks of a UI. With reactive UI, widgets can be associated with streams or observables, allowing them to automatically rebuild whenever the associated data changes. This eliminates the need to manually trigger UI updates and simplifies the process of keeping the interface in sync with the underlying data.
3. Benefits of Reactive UI:
3.1. Enhanced Productivity:
Reactive UI reduces the amount of boilerplate code required to manage UI updates. Developers can focus more on the logic and functionality of their apps, knowing that the UI will automatically react to data changes. This results in a more streamlined and productive development process.
3.2. Consistent User Experience:
With reactive UI, UI updates are consistent and predictable. Users no longer encounter outdated or inconsistent views, as the UI updates in real-time to reflect the most recent data changes. This leads to a smoother and more engaging user experience.
3.3. Separation of Concerns:
Reactive UI encourages the separation of business logic from UI concerns. This separation enhances code organization, making it easier to maintain and test different components of the application independently.
4. Building a Reactive UI in Dart and Flutter:
4.1. Setting up Dependencies:
To get started, make sure you have Flutter installed. Create a new Flutter project and add the necessary dependencies for reactive programming. Popular packages like rxdart provide a rich set of tools for working with streams and observables.
dart dependencies: flutter: sdk: flutter rxdart: ^0.27.2
4.2. Creating an Observable:
Let’s say we’re building a simple counter app. We can create an observable using BehaviorSubject from rxdart to represent the counter value. This observable will allow us to react to changes in the counter value.
dart import 'package:rxdart/rxdart.dart'; class CounterBloc { final _counter = BehaviorSubject<int>.seeded(0); Stream<int> get counterStream => _counter.stream; void increment() { _counter.add(_counter.value + 1); } void dispose() { _counter.close(); } }
4.3. Building the UI:
Now that we have our observable, we can build the UI that reacts to changes in the counter value. We’ll use the StreamBuilder widget to accomplish this.
dart class CounterApp extends StatelessWidget { final CounterBloc _counterBloc = CounterBloc(); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Reactive UI Example')), body: Center( child: StreamBuilder<int>( stream: _counterBloc.counterStream, builder: (context, snapshot) { return Text( 'Counter: ${snapshot.data}', style: TextStyle(fontSize: 24), ); }, ), ), floatingActionButton: FloatingActionButton( onPressed: () => _counterBloc.increment(), child: Icon(Icons.add), ), ), ); } }
4.4. Putting It All Together:
In this example, the UI automatically updates whenever the counter value changes. This is because the StreamBuilder is listening to changes in the counterStream provided by our CounterBloc.
Conclusion
Reactive UI is a powerful approach to building dynamic and responsive user interfaces. By embracing reactive programming concepts with Dart and Flutter, developers can streamline UI updates, ensure a consistent user experience, and improve code organization. The combination of streams, observables, and widgets enables developers to create applications that react to changes in data without the need for manual intervention. As you embark on your journey to mastering reactive UI, remember that practice and experimentation are key. With the right tools and mindset, you can create stunning and engaging user interfaces that keep your users coming back for more.
Table of Contents
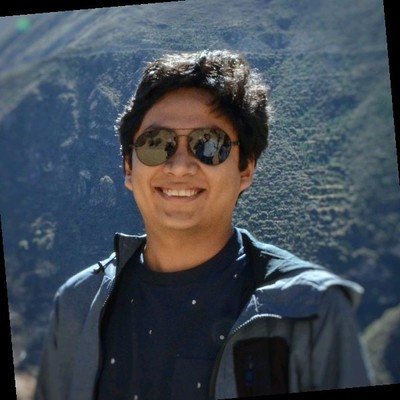
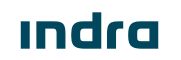