Drupal Module Security, Performance, and More: Best Practices You Need
Drupal is a powerful and flexible content management system that allows developers to create a wide range of websites and web applications. One of the key features that sets Drupal apart from other CMS platforms is its modular architecture, which enables developers to extend and customize Drupal’s functionality through the use of modules. Whether you’re building a simple blog or a complex e-commerce site, following best practices for Drupal module development is crucial for creating maintainable and scalable code. In this blog post, we’ll explore some of the best practices for Drupal module development, along with examples to illustrate each concept.
1. Use Drupal Coding Standards
Drupal has its own coding standards that all module developers should adhere to. These standards help maintain consistency and readability in the codebase. Some key coding standards include:
– PSR-4 Autoloading: Organize your module files into a directory structure that follows the PSR-4 autoloading standard. For example, if your module is named “my_module,” the module files should be located in the `my_module/src` directory.
– Naming Conventions: Follow Drupal’s naming conventions for functions, classes, and variables. Functions should be named using underscores, and classes should use CamelCase. For example, `my_module_function_name()` and `MyModuleClassName`.
– Indentation and Formatting: Use consistent indentation (typically two spaces) and formatting throughout your code. Make sure to properly indent control structures and function declarations.
Example:
```php /** * Implements hook_menu(). */ function my_module_menu() { $items = []; $items['my-page'] = [ 'title' => 'My Page', 'page callback' => 'my_module_page_callback', 'access callback' => 'user_access', 'access arguments' => ['access content'], ]; return $items; } ```
2. Leverage Drupal’s Hook System
Drupal provides a powerful hook system that allows modules to interact with the core and other modules. Hooks enable you to extend Drupal’s functionality and respond to various events. Always use appropriate hooks when developing your module.
Example:
```php /** * Implements hook_preprocess_HOOK() for block templates. */ function my_module_preprocess_block(&$variables) { // Modify block variables here. } ```
3. Secure Your Module
Security is paramount in web development, and Drupal is no exception. Ensure that your module follows security best practices:
– Sanitize User Input: Always sanitize user input to prevent SQL injection, Cross-Site Scripting (XSS), and other security vulnerabilities. Drupal provides functions like `check_plain()` and `filter_xss()` for this purpose.
Example:
```php $unsafe_input = $_POST['user_input']; $sanitized_input = check_plain($unsafe_input); ```
– Permissions and Access Control: Implement proper access control by defining permissions for your module. Only grant access to privileged users when necessary.
Example:
```php /** * Implements hook_permission(). */ function my_module_permission() { return [ 'administer my module' => [ 'title' => t('Administer My Module'), 'description' => t('Access to configure My Module settings.'), ], ]; } ```
– Avoid Direct Database Queries: Whenever possible, use Drupal’s database abstraction layer (DBAL) and functions like `db_select()`, `db_insert()`, and `db_update()` to interact with the database. This helps prevent SQL injection attacks.
Example:
```php $query = db_select('my_table', 't') ->fields('t', ['column1', 'column2']) ->condition('column3', $value) ->execute(); ```
4. Write Clear and Comprehensive Documentation
Good documentation is essential for maintaining and collaborating on Drupal projects. Include clear and comprehensive inline comments and documentation blocks in your code. Explain the purpose of functions, classes, and complex logic.
Example:
```php /** * Implements hook_form_FORM_ID_alter() for the user registration form. * * Adds a custom field to the user registration form. */ function my_module_form_user_register_form_alter(&$form, &$form_state) { // Add a custom field to the registration form. $form['custom_field'] = [ '#type' => 'textfield', '#title' => t('Custom Field'), '#description' => t('Enter your custom field data.'), '#required' => TRUE, ]; } ```
5. Optimize Performance
Drupal websites often need to handle a large number of requests. To ensure your module doesn’t negatively impact performance:
– Caching: Use Drupal’s caching mechanisms to reduce the load on your server. Leverage the `cache_set()` and `cache_get()` functions to store and retrieve cached data.
Example:
```php // Cache a database query result for 1 hour. if (!$data = cache_get('my_module_data')) { $data = db_query('SELECT * FROM {my_table}')->fetchAll(); cache_set('my_module_data', $data, 'cache', time() + 3600); } ```
– Database Queries: Optimize your database queries to be as efficient as possible. Avoid unnecessary joins and limit the data you retrieve.
Example:
```php $query = db_select('my_table', 't') ->fields('t', ['column1']) ->condition('column2', $value) ->range(0, 10) // Limit to the first 10 results. ->execute(); ```
6. Maintain Compatibility
Drupal often undergoes updates and changes. Ensure that your module is compatible with the latest version of Drupal by staying informed about core updates and contributed modules. Test your module on various Drupal versions to ensure compatibility.
7. Version Control and Collaboration
Use version control systems like Git to manage your module’s codebase. Hosting your code on platforms like GitHub or GitLab facilitates collaboration with other developers and makes it easier to track changes.
Conclusion
Drupal module development is a skill that improves with practice and adherence to best practices. By following Drupal coding standards, leveraging Drupal’s hook system, prioritizing security, documenting your code, optimizing performance, and staying compatible with Drupal updates, you can create high-quality and maintainable modules. Remember that the Drupal community is a valuable resource for learning and sharing knowledge, so don’t hesitate to seek help and contribute back to the community as you develop your Drupal modules.
Table of Contents
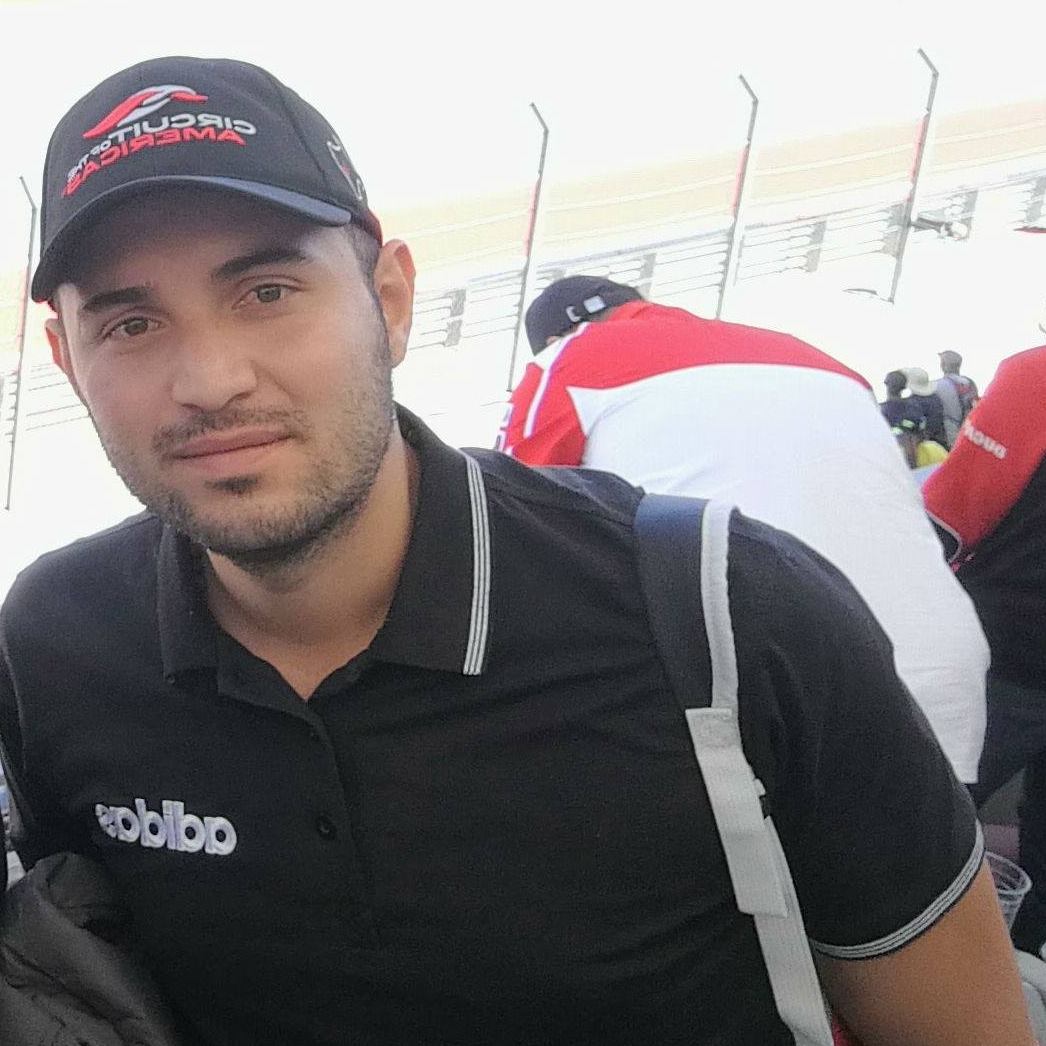
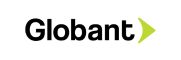