Authentication and Authorization with Flask-Login
In today’s digital landscape, ensuring secure user management is crucial for web applications. Flask-Login, a popular Flask extension, provides an elegant solution for implementing authentication and authorization. In this tutorial, we will explore how to integrate Flask-Login into your Flask application, enabling you to authenticate users and manage access to different resources.
What is Authentication and Authorization?
Authentication and authorization are two essential components of user management in web applications.
- Authentication is the process of verifying the identity of a user. It ensures that the user is who they claim to be by validating their credentials, such as a username and password.
- Authorization is the process of granting or denying access to specific resources based on the user’s authenticated identity. It defines what actions a user can perform and what data they can access within the application.
Why Flask-Login?
Flask-Login is a powerful Flask extension that simplifies the implementation of user authentication and authorization. It provides a user session management system and handles common tasks such as login, logout, and remembering user sessions.
Flask-Login offers the following features:
- User session management: Tracks the user’s session and manages user login state.
- Secure session management: Uses secure cookies to store session data.
- Remember me functionality: Allows users to stay logged in across sessions.
- Integration with Flask’s authentication mechanisms: Works seamlessly with Flask’s user authentication system.
- Flexible user model support: Adapts to your custom user models.
Now, let’s dive into the implementation of authentication and authorization using Flask-Login.
Installing Flask-Login
To get started, ensure you have Flask installed in your virtual environment. Then, install Flask-Login using pip:
shell pip install flask-login
Integrating Flask-Login into Your Flask Application
1. Setting up the User Model
Before using Flask-Login, you need to have a User model that represents your application’s users. Here’s an example using SQLAlchemy:
python from flask_login import UserMixin from sqlalchemy import Column, Integer, String from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base, UserMixin): __tablename__ = 'users' id = Column(Integer, primary_key=True) username = Column(String(64), unique=True) password = Column(String(128))
2. Initializing Flask-Login
Next, initialize Flask-Login in your Flask application:
python from flask import Flask from flask_login import LoginManager app = Flask(__name__) app.config['SECRET_KEY'] = 'your_secret_key' # Replace with your secret key login_manager = LoginManager(app)
3. Implementing User Loader
To load a user from the user model, Flask-Login requires a user loader function. The user loader function retrieves the user based on their unique identifier (ID). Here’s an example:
python @login_manager.user_loader def load_user(user_id): # Retrieve the user from the database based on the ID return User.query.get(int(user_id))
4. Creating Login Views
Create views for login and logout routes in your Flask application:
python from flask import render_template, redirect, url_for, flash from flask_login import login_user, logout_user, login_required @app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': # Validate the user's credentials user = User.query.filter_by(username=request.form['username']).first() if user and user.password == request.form['password']: login_user(user) return redirect(url_for('dashboard')) flash('Invalid username or password.', 'error') return render_template('login.html') @app.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('login'))
5. Securing Routes
To restrict access to certain routes, use the @login_required decorator:
python @app.route('/dashboard') @login_required def dashboard(): # Only logged-in users can access this route return render_template('dashboard.html')
Conclusion
Implementing authentication and authorization in Flask applications is simplified with Flask-Login. By following this comprehensive guide, you have learned how to integrate Flask-Login into your Flask application, handle user login and logout, and secure routes based on user authentication.
Flask-Login’s flexibility and seamless integration with Flask’s ecosystem make it a powerful tool for managing user sessions and access control. Take advantage of Flask-Login to build secure and user-friendly web applications with ease.
Remember, prioritizing user security is crucial for creating trustworthy and reliable web applications in today’s digital world.
Table of Contents
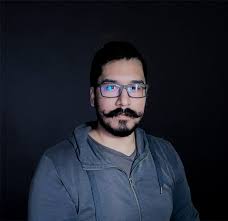