Flask Blueprints: Organizing Large Flask Applications
When building large Flask applications, it’s crucial to maintain a clean and organized codebase. Flask Blueprints provide a powerful way to structure your application into smaller, modular components. In this blog post, we’ll dive into the concept of Flask Blueprints and explore how they can help you build scalable Flask applications. We’ll also provide code samples and best practices to guide you along the way.
What are Flask Blueprints?
Flask Blueprints are a way to divide a Flask application into reusable modules. They allow you to organize your application into logical components, such as different parts of your website or API endpoints. Blueprints encapsulate routes, views, templates, and static files, making it easier to manage and extend your Flask application as it grows.
Benefits of Flask Blueprints
Using Flask Blueprints offers several benefits for organizing large Flask applications:
- Modularity: Blueprints enable you to break down your application into smaller, self-contained modules. This promotes code reuse and makes it easier to collaborate with other developers.
- Scalability: With Blueprints, you can scale your application by adding or removing modules as needed. Each module can be developed independently and plugged into the main application seamlessly.
- Organization: Blueprints provide a structured way to organize your routes, views, and templates. This makes it easier to navigate and maintain your codebase, especially in larger projects.
Implementing Flask Blueprints
To demonstrate how Flask Blueprints work, let’s consider a simple blogging application. We’ll organize our application into three modules: authentication, blog posts, and user profiles.
Step 1: Create the Blueprint Instances
First, we’ll create a new file for each module and define a Blueprint instance in each file. Here’s an example for the authentication module:
python # auth.py from flask import Blueprint auth_bp = Blueprint('auth', __name__) @auth_bp.route('/login') def login(): return 'Login page' @auth_bp.route('/register') def register(): return 'Register page'
Similarly, you can create blueprints for the blog posts and user profiles modules.
Step 2: Register the Blueprints
In your main application file, you need to register the created blueprints. Here’s an example:
python # app.py from flask import Flask from auth import auth_bp from blog import blog_bp from profiles import profiles_bp app = Flask(__name__) app.register_blueprint(auth_bp) app.register_blueprint(blog_bp) app.register_blueprint(profiles_bp)
Step 3: Access the Blueprints
Now that your blueprints are registered, you can access their routes using the Blueprint’s name. Here’s an example:
python # app.py (continued) @app.route('/') def home(): return 'Home page' @app.route('/about') def about(): return 'About page' @app.route('/blog') def blog(): return 'Blog page' @app.route('/profile') def profile(): return 'Profile page'
By using the blueprints, you can keep related routes and views organized within their respective modules.
Conclusion
Flask Blueprints provide a powerful tool for organizing large Flask applications. They promote modularity, scalability, and code organization, making it easier to manage and extend your application. By following the steps outlined in this blog post and leveraging the provided code samples, you can start building scalable Flask applications with confidence. Happy coding!
Table of Contents
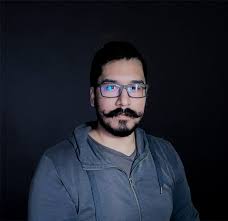