Building RESTful APIs with Flask: A Step-by-Step Tutorial
In today’s interconnected world, building robust and scalable APIs is essential for seamless integration between different applications. Flask, a popular Python web framework, offers a simple and elegant solution for creating RESTful APIs. In this step-by-step tutorial, we will explore the process of building RESTful APIs using Flask and demonstrate how to handle HTTP methods, request/response formats, authentication, and more. Whether you’re a beginner or an experienced developer, this tutorial will help you dive into the world of Flask and empower you to build powerful APIs.
Prerequisites
Before diving into Flask, make sure you have Python and Flask installed on your machine. You can install Flask using pip, the Python package manager, with the following command:
bash pip install flask
Step 1: Setting up the Flask Application
To begin, let’s create a new directory for our Flask application. Open your terminal or command prompt and navigate to your desired directory. Run the following commands to create a virtual environment and install Flask:
bash mkdir flask-api-tutorial cd flask-api-tutorial python3 -m venv env source env/bin/activate pip install flask
Step 2: Creating the API Endpoints
Now that we have our Flask application set up, let’s define our API endpoints. Create a new file named app.py and open it in your preferred text editor. In app.py, import the necessary modules and define the Flask app:
python from flask import Flask app = Flask(__name__) # Define your API endpoints here if __name__ == '__main__': app.run(debug=True)
Step 3: Handling API Routes
To handle API routes, we can use Flask’s route decorators. Let’s start by creating a basic GET endpoint that returns a “Hello, World!” message. Add the following code to app.py:
python @app.route('/api/hello', methods=['GET']) def hello(): return 'Hello, World!'
Step 4: Handling Request Parameters
API endpoints often require additional information through request parameters. Flask provides an easy way to handle them using route parameters or query strings. Let’s create an endpoint that accepts a name parameter and returns a personalized greeting:
python @app.route('/api/greet/<name>', methods=['GET']) def greet(name): return f'Hello, {name}!'
Step 5: Handling POST Requests and JSON Data
To handle POST requests and receive JSON data, we need to import the request module from Flask. Let’s create an endpoint that accepts a JSON payload and echoes it back as the response:
python from flask import request @app.route('/api/echo', methods=['POST']) def echo(): data = request.get_json() return data
Step 6: Error Handling
Error handling is an essential aspect of any API. Flask allows us to define custom error handlers to provide meaningful responses to clients. Let’s create a custom error handler for 404 Not Found errors:
python @app.errorhandler(404) def not_found(error): return '404 Not Found', 404
Step 7: Running the Flask Application
With our API endpoints defined, it’s time to run our Flask application. In your terminal or command prompt, navigate to the project directory and run the following command:
bash python app.py
Conclusion
Congratulations! You’ve successfully built RESTful APIs with Flask. Throughout this tutorial, we covered the basics of Flask, including setting up a Flask application, defining API endpoints, handling various HTTP methods, managing request parameters and JSON data, and implementing error handling. Flask’s simplicity and flexibility make it a powerful choice for developing APIs. Now that you have a solid foundation, continue exploring Flask’s features to build even more robust and scalable APIs.
Remember to check out the Flask documentation and explore additional features like authentication, database integration, and testing. Happy coding!
Note: For the complete code samples and additional examples, refer to the official Flask documentation or the tutorial’s GitHub repository.
Table of Contents
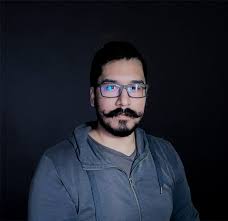