Hiring Flask Developers: A Comprehensive Interview Questions Guide
In the realm of web development, Flask has risen as a versatile and lightweight Python framework for building web applications. To navigate the process of hiring Flask developers effectively, this guide equips you with essential interview questions and strategies to identify top-tier candidates with the technical expertise, problem-solving acumen, and Flask proficiency your team requires.
Table of Contents
1. How to Hire Flask Developers
Set your course for hiring Flask developers with these strategic steps:
- Job Requirements: Define specific job prerequisites outlining the desired skills and experience for your Flask development role.
- Search Channels: Utilize job boards, online platforms, and Python communities to unearth potential Flask developer candidates.
- Screening: Evaluate candidates based on their Flask proficiency, Python skills, and supplementary knowledge.
- Technical Assessment: Develop a comprehensive technical evaluation to assess candidates’ coding abilities, understanding of Flask’s architecture, and problem-solving capabilities.
2. Key Skills to Look for in Flask Developers
When evaluating Flask developers, prioritize these core skills:
- Flask Proficiency: A deep understanding of Flask’s core concepts, routing, views, and extensions for building scalable and maintainable web applications.
- Python Expertise: Proficiency in Python programming, as Flask is a Python framework, and familiarity with Python’s syntax, data structures, and libraries.
- Web Development: Knowledge of front-end technologies like HTML, CSS, and JavaScript, as well as the ability to create interactive and user-friendly web interfaces.
- Database Integration: Experience in integrating various databases like SQLite, MySQL, or PostgreSQL with Flask applications, including designing and querying databases.
- RESTful API Design: Familiarity with designing and implementing RESTful APIs using Flask-RESTful or other extensions.
- Version Control: Proficiency in using version control systems like Git to collaborate effectively with team members on codebases.
3. Flask Developer Hiring Process Overview
Here’s a high-level overview of the Flask developer hiring process:
3.1 Define Job Requirements and Skillsets
Lay a strong foundation by outlining clear job requirements, specifying the skills and experience you’re seeking in Flask developer candidates.
3.2 Craft Compelling Job Descriptions
Create enticing job descriptions that accurately convey the responsibilities and opportunities associated with the Flask development role.
3.3 Develop Flask Developer Interview Questions
Design a comprehensive set of interview questions covering Flask intricacies, RESTful API design, database interactions, and web development technologies.
4. Sample Flask Developer Interview Questions and Answers
Explore these sample questions and answers to assess candidates’ Flask skills:
Q1. Explain the purpose of Flask in web development. How does Flask enable the creation of web applications?
A: Flask is a micro web framework for Python that simplifies the process of building web applications. It provides tools, libraries, and routing mechanisms to create routes, handle requests, and render templates, making web development more efficient.
Q2. Describe the process of setting up a basic Flask application. What are the essential components and directories?
A: To set up a basic Flask app, you’d import the Flask module, create an instance of the Flask class, and define routes using the @app.route
decorator. Essential components include routes, views, and templates stored in the “templates” directory.
Q3. Implement a Flask route that handles a GET request to the “/hello” endpoint and returns a “Hello, World!” message.
from flask import Flask app = Flask(__name__) @app.route('/hello') def hello(): return 'Hello, World!' if __name__ == '__main__': app.run()
Q4. Explain the role of Flask extensions. How can you use extensions to add additional functionality to a Flask application?
A: Flask extensions are third-party libraries that provide additional features and tools to enhance a Flask application. Extensions can be used to add functionalities like database integration, authentication, API development, and more.
Q5. What is Flask-WTF? How can you use it to handle form validation and data submission in Flask applications?
A: Flask-WTF is an extension that simplifies form handling and validation in Flask applications. It provides tools to create and render HTML forms, validate form data, and protect against cross-site request forgery (CSRF) attacks.
Q6. Describe the process of using SQLAlchemy with Flask for database integration. How can you define models and perform database operations?
A: SQLAlchemy is a popular database toolkit in Python. With Flask-SQLAlchemy, you can define models as Python classes and interact with databases using Python objects. You can create, read, update, and delete records using SQLAlchemy’s query methods.
Q7. Explain how you can implement authentication in a Flask application. What are the considerations for securely managing user passwords?
A: Authentication in Flask involves verifying user identities. You can use Flask-Login or other extensions to manage user sessions and authentication tokens. For secure password management, use libraries like Werkzeug to hash and salt passwords before storing them.
Q8. What is Flask’s approach to handling static files such as CSS, JavaScript, and images? How can you serve static files in a Flask application?
A: Flask provides a built-in way to serve static files from the “static” directory. You can use the url_for('static', filename='...')
function to generate URLs for static files, ensuring proper caching and versioning.
Q9. Explain the concept of Flask Blueprints. How can you use Blueprints to modularize and organize routes in a Flask application?
A: Flask Blueprints are a way to divide a Flask application into smaller, modular components. They allow you to organize routes, views, and templates into separate units, which can be registered and reused across applications.
Q10. How can you deploy a Flask application to production? Mention different deployment options and best practices.
A: Flask applications can be deployed to production using platforms like Heroku, AWS, or traditional web hosting. To ensure a smooth deployment, configure production settings, implement security measures, enable HTTPS, and optimize performance.
Q11. Explain the purpose of Flask’s request
and session
objects. Provide an example of using these objects to handle form submissions and manage user sessions.
A: The request
object in Flask contains information about the incoming HTTP request, while the session
object is used to store data that persists across multiple requests.
from flask import Flask, request, session app = Flask(__name__) app.secret_key = 'your_secret_key' @app.route('/login', methods=['POST']) def login(): if request.method == 'POST': username = request.form['username'] password = request.form['password'] # Authenticate user and set session data if authenticate(username, password): session['username'] = username return 'Login successful' else: return 'Login failed' @app.route('/dashboard') def dashboard(): if 'username' in session: return f'Welcome, {session["username"]}!' else: return 'Please log in first' if __name__ == '__main__': app.run()
Q12. Describe the role of Flask’s built-in template engine, Jinja2. How can you use Jinja2 to render dynamic content in Flask views?
A: Jinja2 is a powerful and flexible template engine used in Flask for rendering dynamic content in HTML views. It supports template inheritance, macros, filters, and more.
<!-- templates/base.html --> <!DOCTYPE html> <html> <head> <title>{% block title %}{% endblock %}</title> </head> <body> {% block content %}{% endblock %} </body> </html> <!-- templates/index.html --> {% extends "base.html" %} {% block title %}Flask App{% endblock %} {% block content %} <h1>Hello, {{ user }}!</h1> {% endblock %}
Q13. Explain the concept of Flask’s request and response lifecycle. How does Flask handle the flow of data from the client’s request to the server’s response?
A: In Flask, when a client sends an HTTP request, the request is routed to a corresponding view function. The view function processes the request, interacts with the database if needed, and generates a response, which is then sent back to the client.
Q14. How can you implement URL parameter handling in Flask? Provide an example of defining a route that accepts an integer parameter and displays related content.
A: URL parameters in Flask are captured using the <>
syntax in route definitions. The captured values are passed as arguments to the view function.
from flask import Flask app = Flask(__name__) @app.route('/user/<int:user_id>') def get_user(user_id): # Fetch user data from the database using user_id user_data = fetch_user_data(user_id) return f'User ID: {user_id}, Name: {user_data["name"]}' if __name__ == '__main__': app.run()
5. Hiring Flask Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your Flask development project’s specifics, preferred skills, and desired experience levels.
Step 2: Identify the Right Match: Within a brief period, CloudDevs presents you with carefully selected Flask developers from their network of pre-vetted professionals. Review their profiles and select the candidate whose expertise aligns seamlessly with your project’s goals.
Step 3: Begin a Trial Engagement: Engage in discussions with your chosen developer to ensure a smooth integration into your team. Once satisfied, formalize the collaboration and embark on a risk-free trial period spanning one week.
By leveraging the expertise of CloudDevs, you can seamlessly identify and hire top-tier Flask developers, ensuring your team possesses the skills necessary to craft exceptional web applications.
6. Conclusion
With these comprehensive interview questions and insights at your disposal, you’re well-equipped to assess Flask developers comprehensively. Whether you’re building RESTful APIs or dynamic web applications, securing the right Flask developers through CloudDevs is vital for the success of your Python web development projects.
Table of Contents
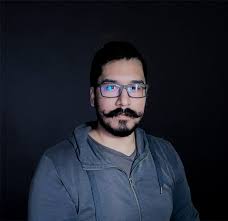