Flask and SQLalchemy: Integrating Databases into Your Web App
In today’s digital world, web applications often rely heavily on databases to store and manage data. Flask, a popular Python web framework, provides an excellent foundation for building web applications. When it comes to working with databases, Flask seamlessly integrates with SQLAlchemy, a powerful and flexible Object-Relational Mapping (ORM) library. In this blog post, we will explore how to integrate databases into your Flask web application using SQLAlchemy.
What is SQLAlchemy?
SQLAlchemy is a Python library that provides a set of high-level and low-level API for working with relational databases. It allows developers to interact with databases using Python objects, making it easier to manage database operations. SQLAlchemy provides a powerful ORM that abstracts away the complexities of writing raw SQL queries and provides a more intuitive and Pythonic way of working with databases.
Setting up Flask and SQLAlchemy
To get started, let’s set up a basic Flask application and configure SQLAlchemy to connect to a database. First, make sure you have Flask and SQLAlchemy installed:
bash $ pip install flask sqlalchemy
Next, let’s create a new Flask application in a file named app.py and import the necessary modules:
python from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///your_database.db' db = SQLAlchemy(app)
In the above code, we imported the Flask module and created a Flask application. We also imported the SQLAlchemy module and initialized it with our Flask application. The SQLALCHEMY_DATABASE_URI configuration variable specifies the connection URL for the database. In this example, we are using a SQLite database named your_database.db.
Defining Database Models
In SQLAlchemy, database tables are represented as Python classes called models. Each attribute of the model class represents a column in the corresponding database table. Let’s define a simple User model that represents a user in our application:
python class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(50), unique=True) email = db.Column(db.String(120), unique=True) def __repr__(self): return f'<User {self.username}>'
In the above code, we defined a User model that has three columns: id, username, and email. The id column is marked as the primary key, and both the username and email columns are marked as unique. The __repr__ method specifies how the model should be represented when printed.
Creating Database Tables
Before we can use our models, we need to create the corresponding database tables. SQLAlchemy provides a convenient way to create database tables based on the defined models. Open a Python shell or add the following code to your app.py file:
python @app.cli.command('create_db') def create_db(): db.create_all() print('Database tables created.')
Now, you can run the following command to create the database tables:
bash $ flask create_db
Performing Database Operations
Once the database tables are created, we can perform various database operations using our models. Let’s see some examples:
1. Adding a User
python user = User(username='john_doe', email='john@example.com') db.session.add(user) db.session.commit()
2. Querying Users
python users = User.query.all() for user in users: print(user.username)
3. Updating a User
python user = User.query.filter_by(username='john_doe').first() user.email = 'john_new@example.com' db.session.commit()
4. Deleting a User
python user = User.query.filter_by(username='john_doe').first() db.session.delete(user) db.session.commit()
Conclusion
In this blog post, we explored how to integrate databases into your Flask web application using SQLAlchemy. We learned how to set up Flask and SQLAlchemy, define database models, create database tables, and perform various database operations. SQLAlchemy’s powerful ORM makes working with databases in Flask a breeze, allowing you to focus on building your web application without worrying about low-level database interactions. Happy coding!
By integrating SQLAlchemy into your Flask web application, you gain a powerful tool for working with databases efficiently and effectively. The provided code samples demonstrate the basic setup, model definition, table creation, and common database operations. With this knowledge, you can confidently develop robust web applications that leverage the full potential of databases.
Table of Contents
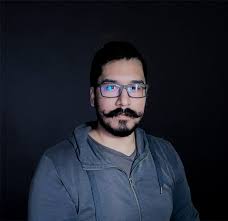