Flask Templates: Creating Dynamic Web Pages with Jinja2
In web development, creating dynamic web pages that can adapt to different data and user inputs is crucial. Flask, a popular Python web framework, provides an excellent templating engine called Jinja2. Jinja2 allows developers to separate the presentation logic from the business logic, making it easier to maintain and modify web applications. In this blog post, we will explore Flask templates and dive into the powerful features of Jinja2 with code samples.
Setting Up Flask and Jinja2
Before we start, make sure you have Flask installed. You can install it using pip:
ruby $ pip install Flask
Next, create a new Flask application and set up the basic structure. Here’s an example:
python from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('index.html') if __name__ == '__main__': app.run(debug=True)
Creating a Basic Template
To create a Flask template, we use HTML files with additional Jinja2 syntax. Let’s create a basic template called index.html:
html <!DOCTYPE html> <html> <head> <title>Flask Templates</title> </head> <body> <h1>Welcome to Flask Templates</h1> </body> </html>
Passing Data to Templates
One of the powerful features of Jinja2 is the ability to pass data from the server-side to the templates. Let’s modify our home function to pass a variable called name to the template:
python @app.route('/') def home(): name = 'John Doe' return render_template('index.html', name=name)
And update the index.html template to display the name:
html <!DOCTYPE html> <html> <head> <title>Flask Templates</title> </head> <body> <h1>Welcome to Flask Templates, {{ name }}!</h1> </body> </html>
Using Control Structures
Jinja2 also provides control structures like loops and conditionals. Let’s enhance our template to display a list of items:
python @app.route('/') def home(): items = ['Apple', 'Banana', 'Orange'] return render_template('index.html', items=items)
And update the index.html template:
html <!DOCTYPE html> <html> <head> <title>Flask Templates</title> </head> <body> <h1>Welcome to Flask Templates!</h1> <ul> {% for item in items %} <li>{{ item }}</li> {% endfor %} </ul> </body> </html>
Conclusion
Flask templates, powered by Jinja2, offer a flexible and efficient way to create dynamic web pages. We explored the basics of Flask templates and learned how to pass data and use control structures. This allows developers to build web applications that adapt to changing requirements and deliver personalized experiences to users. With Flask and Jinja2, the possibilities are endless. Happy templating!
Remember, this blog post only scratches the surface of Flask templates and Jinja2. Feel free to explore the official Flask and Jinja2 documentation to uncover more advanced techniques and features.
Table of Contents
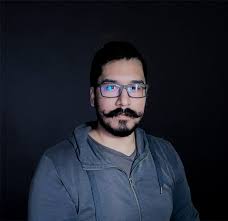