Explain the concept of stateful and stateless widgets in Flutter.
In Flutter, widgets are the building blocks of the user interface, and they can be broadly categorized into two types: stateful and stateless widgets. Understanding the distinction between these two is crucial for efficient Flutter development.
Stateless Widgets:
Stateless widgets are immutable, meaning their properties cannot change once they are initialized. They represent parts of the user interface that don’t change dynamically based on user interactions or other factors. Stateless widgets are particularly useful for static content or UI components that remain constant throughout the app’s lifecycle. An example of a stateless widget is the `Container` widget, which defines a box model for positioning and styling.
```dart class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return Container( color: Colors.blue, child: Text('Hello, Stateless!'), ); } } ```
Stateful Widgets:
In contrast, stateful widgets are dynamic and mutable. They can rebuild themselves in response to user actions, device events, or changes in data. Stateful widgets maintain a separate state object that can be updated over time, triggering the rebuilding of the widget tree. An example is the `TextField` widget, which allows users to input text, and its state would change as users type.
```dart class InputField extends StatefulWidget { @override _InputFieldState createState() => _InputFieldState(); } class _InputFieldState extends State<InputField> { String inputValue = ''; @override Widget build(BuildContext context) { return TextField( onChanged: (value) { setState(() { inputValue = value; }); }, decoration: InputDecoration(labelText: 'Type something...'), ); } } ```
Key Points:
- Stateless widgets are static and unchanging, while stateful widgets are dynamic and can be updated.
- Stateful widgets use a separate state object to manage mutable data.
- Examples of stateless widgets include `Container` and `Text`, while examples of stateful widgets include `TextField` and `ListView`.
- Understanding when to use each type of widget is crucial for optimizing Flutter app performance and responsiveness.
Table of Contents
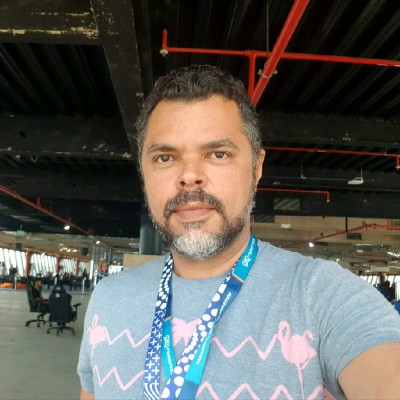
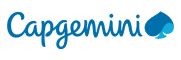