Go and Microservices: Developing Scalable and Resilient Systems
In today’s fast-paced and highly competitive software industry, building scalable and resilient systems is crucial to meet the demands of modern applications. Traditional monolithic architectures often struggle to keep up with the ever-increasing user traffic and the need for rapid iterations. This is where microservices architecture comes into play. By breaking down complex applications into smaller, independent services, developers can achieve greater scalability and resilience. In this blog post, we will explore how Go, a popular programming language, can be leveraged to develop scalable and resilient systems using microservices.
1. What are Microservices?
Microservices are a software architecture pattern where complex applications are decomposed into smaller, independent services. Each service focuses on a specific business capability and can be developed, deployed, and scaled independently. These services communicate with each other through lightweight mechanisms, typically APIs or message queues, to perform larger application tasks.
2. Advantages of Microservices Architecture
Microservices offer several benefits over traditional monolithic architectures:
- Scalability: Microservices allow independent scaling of each service, enabling efficient resource utilization and the ability to handle varying loads. This flexibility enables applications to scale horizontally as demand grows.
- Resilience: In a microservices architecture, failures in one service don’t bring down the entire system. Services can be designed with resilience in mind, allowing them to handle failures gracefully and recover quickly.
- Agility and Iteration: Microservices facilitate faster development cycles as individual services can be developed and deployed independently. This enables teams to iterate on specific functionalities without affecting the entire application.
- Technology Diversity: Different services within a microservices architecture can be built using different technologies, chosen based on their suitability for the specific task. This freedom allows developers to select the best tool for each job.
3. Go: A Powerful Language for Microservices
Go, also known as Golang, has gained significant popularity in recent years due to its simplicity, performance, and excellent support for building concurrent systems. Go’s features make it an excellent choice for developing microservices:
3.1 Lightweight and Fast
Go is designed to be a lightweight language with a small runtime footprint. It compiles to machine code, which results in highly performant binaries. This characteristic makes Go well-suited for developing microservices that need to handle a large number of requests efficiently.
3.2 Concurrency and Goroutines
Go’s built-in concurrency primitives, such as goroutines and channels, make it easy to write concurrent programs. Goroutines are lightweight threads that can be multiplexed onto a smaller number of OS threads, allowing efficient utilization of system resources. This makes Go particularly suitable for handling high-concurrency scenarios often encountered in microservices architectures.
go func fetchData(url string) ([]byte, error) { // Perform HTTP request concurrently using goroutines responseChan := make(chan []byte, 1) errorChan := make(chan error, 1) go func() { resp, err := http.Get(url) if err != nil { errorChan <- err return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { errorChan <- err return } responseChan <- body }() select { case response := <-responseChan: return response, nil case err := <-errorChan: return nil, err } }
In the code sample above, we use goroutines to fetch data from a URL concurrently. The fetchData function returns a channel that receives the response or error. By leveraging goroutines, we can perform multiple HTTP requests concurrently and efficiently.
3.3 Strong Typing and Error Handling
Go’s static typing helps catch many errors at compile-time, reducing the likelihood of runtime failures. Additionally, Go promotes explicit error handling, making it easier to write robust and fault-tolerant code. These characteristics are valuable when building microservices that require high reliability.
4. Designing Microservices with Go
When designing microservices in Go, several considerations should be taken into account to ensure scalability and resilience:
4.1 Service Decoupling and Communication
Microservices should be loosely coupled to allow independent development and deployment. This is typically achieved through well-defined APIs and message-based communication. Go provides excellent support for building HTTP-based APIs using its net/http package. Additionally, message queues like RabbitMQ or Apache Kafka can be used for asynchronous communication between services.
4.2 Service Discovery and Load Balancing
In a microservices architecture, services need to discover each other dynamically. Service discovery mechanisms, such as HashiCorp Consul or Kubernetes’ service discovery features, can be used to facilitate this process. Load balancing is also critical to distribute incoming requests evenly across multiple instances of a service. Tools like NGINX or HAProxy can be used for load balancing in Go microservices.
4.3 Data Storage and Persistence
Each microservice typically has its dedicated data storage requirements. Go provides various database drivers and libraries for interacting with different databases, such as MySQL, PostgreSQL, or MongoDB. Additionally, Go’s support for ORM (Object-Relational Mapping) libraries like GORM or SQLBoiler simplifies database access and manipulation.
4.4 Fault Tolerance and Resilience
Microservices need to be designed to handle failures gracefully. Techniques like circuit breakers, retries, and timeouts can be employed to ensure resilience. Libraries like Hystrix or GoResiliency provide fault tolerance mechanisms that can be easily integrated into Go microservices.
5. Implementing a Microservice in Go
Now, let’s dive into the practical aspect of implementing a microservice in Go. We’ll walk through the steps involved in setting up a Go development environment, defining the service interface, implementing business logic, exposing API endpoints, and testing the service.
5.1 Setting Up a Go Development Environment
To get started, ensure that Go is installed on your system. You can download the latest stable release from the official Go website (golang.org) and follow the installation instructions for your operating system. Once installed, verify that Go is correctly set up by running the following command in your terminal:
shell go version
This should display the installed Go version.
5.2 Defining the Service Interface
Before implementing the service, it’s crucial to define the service interface and the messages exchanged between the service and its clients. This can be done using a protocol definition language like Protocol Buffers or OpenAPI (formerly known as Swagger). These tools provide a language-agnostic way to describe APIs and generate client and server code in various programming languages.
For example, using Protocol Buffers, you can define a simple message structure and service interface:
protobuf syntax = "proto3"; message Request { string message = 1; } message Response { string result = 1; } service MyService { rpc Process(Request) returns (Response); }
Once the interface is defined, you can use the protoc compiler to generate Go code from the protocol definition.
5.3 Implementing Business Logic
With the service interface defined, it’s time to implement the business logic of the microservice. Go provides an extensive standard library and many third-party packages that can be leveraged to build robust microservices. Implement the logic according to your service’s requirements and leverage the power of Go’s concurrency features when appropriate.
go type MyService struct {} func (s *MyService) Process(ctx context.Context, req *pb.Request) (*pb.Response, error) { // Business logic implementation result := "Hello, " + req.Message return &pb.Response{Result: result}, nil }
In the code snippet above, we define a MyService struct and implement the Process method as specified in the generated Go code from the protocol definition. This method handles the incoming requests and performs the necessary business logic.
5.4 Exposing API Endpoints
To expose the microservice’s functionality over HTTP, we can utilize Go’s net/http package. Implement HTTP handlers that receive requests, parse the necessary data, and invoke the corresponding service methods.
go func main() { service := &MyService{} http.HandleFunc("/process", func(w http.ResponseWriter, r *http.Request) { // Parse request and invoke the service method req := &pb.Request{Message: r.FormValue("message")} res, err := service.Process(context.Background(), req) if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return } // Write response w.Header().Set("Content-Type", "application/json") json.NewEncoder(w).Encode(res) }) log.Fatal(http.ListenAndServe(":8080", nil)) }
In this example, we define an HTTP handler for the /process endpoint. The handler extracts the request data, invokes the Process method of the MyService instance, and sends the response back to the client.
5.5 Testing and Continuous Integration
Testing is an integral part of building reliable microservices. Go has excellent support for testing with its built-in testing package. Write unit tests to ensure the correctness of your service’s business logic and integration tests to verify the behavior of the entire microservice.
To automate testing and ensure code quality, integrate your Go microservice with a continuous integration (CI) system like Jenkins, Travis CI, or CircleCI. Set up a pipeline that automatically builds, tests, and deploys your microservice whenever changes are pushed to the version control system.
6. Deploying and Scaling Go Microservices
Once your Go microservice is ready, it needs to be deployed and scaled to handle real-world workloads. Containerization and orchestration tools have become the de facto standards for deploying microservices. Let’s explore some of the options available for deploying and scaling Go microservices.
6.1 Containerization with Docker
Docker provides a lightweight and portable containerization platform that simplifies the deployment of microservices. By packaging your Go microservice into a Docker container, you can ensure consistency across different environments and streamline the deployment process. Define a Dockerfile that describes the necessary steps to build and run your Go microservice within a Docker container.
Dockerfile FROM golang:1.17-alpine WORKDIR /app COPY go.mod go.sum ./ RUN go mod download COPY . . RUN go build -o myservice . CMD ["./myservice"]
In this example Dockerfile, we use the official Go Docker image as the base image, copy the necessary Go module files, download the dependencies, copy the entire application code, build the binary, and set the command to start the microservice.
Once the Dockerfile is defined, you can build the Docker image and run your Go microservice within a container.
6.2 Orchestration with Kubernetes
Kubernetes is a popular container orchestration platform that simplifies the management and scaling of containerized applications. It provides features like service discovery, load balancing, automatic scaling, and self-healing. Deploying a Go microservice to Kubernetes involves creating a deployment manifest that describes the desired state of your microservice.
yaml apiVersion: apps/v1 kind: Deployment metadata: name: myservice-deployment spec: replicas: 3 selector: matchLabels: app: myservice template: metadata: labels: app: myservice spec: containers: - name: myservice image: myservice:latest ports: - containerPort: 8080
In the example deployment manifest above, we specify the desired number of replicas, the container image to use, and the port on which the microservice listens. Kubernetes will ensure that the specified number of replicas are running and handle load balancing and scaling automatically.
6.3 Scaling and Load Balancing
Scaling Go microservices can be achieved by either horizontally scaling the instances or vertically scaling the underlying resources. Horizontal scaling involves adding more instances of the microservice to handle increased traffic. Load balancers can distribute incoming requests evenly across these instances. Tools like Kubernetes, Docker Swarm, or cloud providers’ managed services simplify horizontal scaling.
Vertical scaling, on the other hand, involves increasing the resources available to each instance, such as CPU and memory. This can be done manually or by leveraging auto-scaling features provided by cloud providers.
7. Monitoring and Observability
Monitoring and observability are crucial for maintaining the health and performance of microservices. Various tools and techniques can help you gain insights into your Go microservices.
7.1 Logging and Tracing
Proper logging is essential for debugging and understanding the behavior of your microservices. Go provides a powerful logging package called log, which allows you to write logs to different output sources, such as files or centralized logging systems.
Tracing is another valuable technique for understanding the flow of requests across microservices. OpenTelemetry is a popular open-source framework that provides tracing capabilities. By instrumenting your Go microservices with OpenTelemetry, you can collect detailed tracing information and visualize the request flow.
7.2 Metrics and Monitoring Tools
Monitoring tools like Prometheus and Grafana can be used to collect and visualize various metrics from your Go microservices. Go provides a expvar package, which allows you to expose custom metrics in a format compatible with Prometheus. You can then configure Prometheus to scrape these metrics and use Grafana to create informative dashboards.
7.3 Distributed Tracing
Distributed tracing provides end-to-end visibility into the entire request lifecycle as it traverses multiple microservices. Tools like Jaeger or Zipkin enable distributed tracing in Go microservices. By instrumenting your microservices with tracing libraries and configuring them to report trace data to a central tracing system, you can gain insights into request latency, identify performance bottlenecks, and troubleshoot issues.
Conclusion
In this blog post, we explored the powerful combination of Go and microservices for building scalable and resilient systems. We discussed the advantages of microservices architecture, highlighted the strengths of Go as a programming language, and provided practical guidance for designing, implementing, deploying, and monitoring Go microservices.
By leveraging Go’s simplicity, concurrency features, and ecosystem of libraries, developers can create robust microservices that scale horizontally, handle failures gracefully, and meet the demands of modern applications. Embracing microservices and Go together opens up a world of possibilities for building scalable and resilient systems.
So, if you’re ready to take your software architecture to the next level, dive into the world of Go and microservices, and unlock the potential for scalable and resilient systems that can meet the challenges of today’s software landscape.
Table of Contents
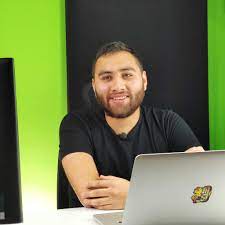
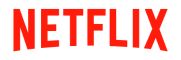